JavaScript Animations
Last Updated :
27 Dec, 2023
JavaScript is a very powerful scripting language. We can create animations in JavaScript using some CSS properties on the DOM elements. In this article, we will create some animations using JavaScript.
We can use the following methods to create animations using JavaScript:
Creating animation using the setTimeout() method
The setTimeout() method can be used to call the animation function once after the given time. We can use it with an event and make it call the callback function every time the event occurs.
Syntax:
setTimeout(callback_function, delay_time);
Example: The below example implements the setTimeout() method to create a JavaScript animation.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 .0">
< title >JavaScript - Animations</ title >
< style >
.animate {
font-size: 25px;
color: green;
font-weight: 600;
}
</ style >
</ head >
< body >
< center >
< h1 >GeeksforGeeks</ h1 >
< p id = "para" >
Hover on this text to see the
animation after 100 milliseconds.
</ p >
</ center >
< script >
const myPara = document.
getElementById('para');
// Function to add animation
function animatePara() {
myPara.classList.add('animate');
}
// Function to remove animation
function removeAnimation() {
myPara.classList.remove('animate');
}
// Attaching functions with events
// to add and remove animation
myPara.addEventListener('mouseover', () => {
setTimeout(animatePara, 100);
})
myPara.addEventListener('mouseout', () => {
setTimeout(removeAnimation, 100);
})
</ script >
</ body >
</ html >
|
Output:

Creating animation using the setInterval() method
The setInterval() method can be used to create the infinite animations that runs automatically and infinitely. It also takes a callback function and repeatedtly calls it after the given delay time.
Syntax:
setInterval(callback_function, delay_time);
Example: The below example uses the setInterval() method to create animation using JavaScript.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >JavaScript - Animations</ title >
< style >
.animate {
font-size: 25px;
color: green;
font-weight: 600;
}
.processing {
height: 20px;
width: 200px;
border-radius: 15px;
border: 2px solid #000;
position: relative;
}
.processing_inner {
color: green;
position: absolute;
left: 0;
transition: all 0.5s ease-in;
}
.processing_inner_animate {
left: 100px;
transition: all 0.5s ease-out;
}
</ style >
</ head >
< body >
< center >
< h1 >GeeksforGeeks</ h1 >
< p id = "para" >
This text will automatically toggle
its color and font size after 1000
milliseconds.
</ p >
< div class = "processing" >
< div id = "process_bar" class = "processing_inner" >
GeeksforGeeks
</ div >
</ div >
</ center >
< script >
const myPara = document.
getElementById('para');
const processBar = document.
getElementById('process_bar');
function animatePara() {
myPara.classList.toggle('animate');
processBar.classList.
toggle('processing_inner_animate')
}
setInterval(animatePara, 1000);
</ script >
</ body >
</ html >
|
Output:
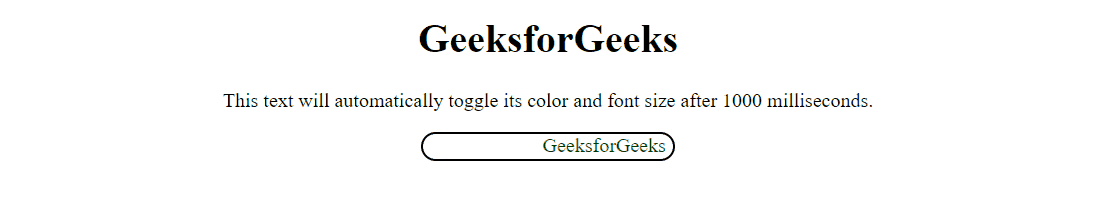
Creating animation by using style property
The style property can be used to add some inline styles to an element. We can use this property with a event to add and remove styles on the occurrence of the event.
Syntax:
element.style.css_property_name = value
Example: In the below code, we will use the style property to create an animation using JavaScript.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >JavaScript - Animations</ title >
< style >
.defInp {
border: none;
width: 200px;
padding: 5px;
}
.defInp:focus-visible {
outline: none;
}
.border {
height: 2px;
width: 200px;
background-color: #ccc;
position: relative;
}
.inner_border {
height: 2px;
width: 0;
position: absolute;
left: 0;
background-color: blue;
transition: all 0.3s ease-in;
}
.animate {
width: 100%;
}
</ style >
</ head >
< body >
< center >
< h1 >GeeksforGeeks</ h1 >
< label for = "myInput" >Input Box: </ label >< br />< br />
< input type = "text" placeholder = "Enter Something..."
id = "myInput" class = "defInp" >
< div class = "border" >
< div class = "inner_border" id = "borBot" ></ div >
</ div >
</ center >
< script >
const myInput = document.
getElementById('myInput');
const borBot = document.
getElementById('borBot');
function animateInp() {
borBot.classList.add('animate');
}
function remAnimateInp() {
borBot.classList.remove('animate');
}
myInput.addEventListener('focus', animateInp);
myInput.addEventListener('blur', remAnimateInp);
</ script >
</ body >
</ html >
|
Output:
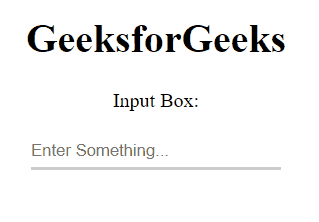
Share your thoughts in the comments
Please Login to comment...