Iterative diagonal traversal of binary tree
Last Updated :
31 May, 2023
Consider lines of slope -1 passing between nodes. Given a Binary Tree, print all diagonal elements in a binary tree belonging to the same line.
Input : Root of below tree
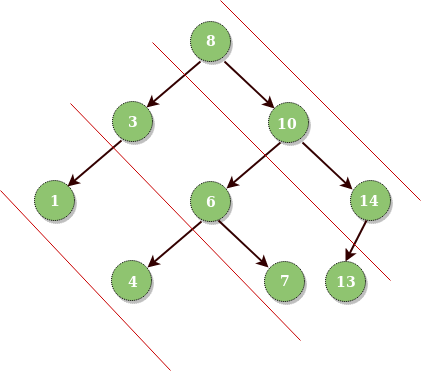
Output :
Diagonal Traversal of binary tree :
8 10 14
3 6 7 13
1 4
We have discussed the recursive solution in the below post.
Diagonal Traversal of Binary Tree
In this post, an iterative solution is discussed. The idea is to use a queue to store only the left child of the current node. After printing the data of the current node make the current node to its right child if present.
A delimiter NULL is used to mark the starting of the next diagonal.
Below is the implementation of the above approach.
C++
#include <bits/stdc++.h>
using namespace std;
struct Node {
int data;
Node *left, *right;
};
Node* newNode( int data)
{
Node* node = (Node*) malloc ( sizeof (Node));
node->data = data;
node->left = node->right = NULL;
return (node);
}
void diagonalPrint(Node* root)
{
if (root == NULL)
return ;
queue<Node*> q;
q.push(root);
q.push(NULL);
while (!q.empty()) {
Node* temp = q.front();
q.pop();
if (temp == NULL) {
if (q.empty())
return ;
cout << endl;
q.push(NULL);
}
else {
while (temp) {
cout << temp->data << " " ;
if (temp->left)
q.push(temp->left);
temp = temp->right;
}
}
}
}
int main()
{
Node* root = newNode(8);
root->left = newNode(3);
root->right = newNode(10);
root->left->left = newNode(1);
root->left->right = newNode(6);
root->right->right = newNode(14);
root->right->right->left = newNode(13);
root->left->right->left = newNode(4);
root->left->right->right = newNode(7);
diagonalPrint(root);
}
|
Java
import java.util.*;
public class solution
{
static class Node {
int data;
Node left, right;
};
static Node newNode( int data)
{
Node node = new Node();
node.data = data;
node.left = node.right = null ;
return (node);
}
static void diagonalPrint(Node root)
{
if (root == null )
return ;
Queue<Node> q= new LinkedList<Node>();
q.add(root);
q.add( null );
while (q.size()> 0 ) {
Node temp = q.peek();
q.remove();
if (temp == null ) {
if (q.size()== 0 )
return ;
System.out.println();
q.add( null );
}
else {
while (temp!= null ) {
System.out.print( temp.data + " " );
if (temp.left!= null )
q.add(temp.left);
temp = temp.right;
}
}
}
}
public static void main(String args[])
{
Node root = newNode( 8 );
root.left = newNode( 3 );
root.right = newNode( 10 );
root.left.left = newNode( 1 );
root.left.right = newNode( 6 );
root.right.right = newNode( 14 );
root.right.right.left = newNode( 13 );
root.left.right.left = newNode( 4 );
root.left.right.right = newNode( 7 );
diagonalPrint(root);
}
}
|
Python3
class Node:
def __init__( self ,data):
self .val = data
self .left = None
self .right = None
def diagonalprint(root):
if root is None :
return
q = []
q.append(root)
q.append( None )
while len (q) > 0 :
temp = q.pop( 0 )
if not temp:
if len (q) = = 0 :
return
print ( ' ' )
q.append( None )
else :
while temp:
print (temp.val, end = ' ' )
if temp.left:
q.append(temp.left)
temp = temp.right
root = Node( 8 )
root.left = Node( 3 )
root.right = Node( 10 )
root.left.left = Node( 1 )
root.left.right = Node( 6 )
root.right.right = Node( 14 )
root.right.right.left = Node( 13 )
root.left.right.left = Node( 4 )
root.left.right.right = Node( 7 )
diagonalprint(root)
|
C#
using System;
using System.Collections;
class GFG
{
public class Node
{
public int data;
public Node left, right;
};
static Node newNode( int data)
{
Node node = new Node();
node.data = data;
node.left = node.right = null ;
return (node);
}
static void diagonalPrint(Node root)
{
if (root == null )
return ;
Queue q = new Queue();
q.Enqueue(root);
q.Enqueue( null );
while (q.Count > 0)
{
Node temp = (Node) q.Peek();
q.Dequeue();
if (temp == null )
{
if (q.Count == 0)
return ;
Console.WriteLine();
q.Enqueue( null );
}
else
{
while (temp != null )
{
Console.Write( temp.data + " " );
if (temp.left != null )
q.Enqueue(temp.left);
temp = temp.right;
}
}
}
}
public static void Main(String []args)
{
Node root = newNode(8);
root.left = newNode(3);
root.right = newNode(10);
root.left.left = newNode(1);
root.left.right = newNode(6);
root.right.right = newNode(14);
root.right.right.left = newNode(13);
root.left.right.left = newNode(4);
root.left.right.right = newNode(7);
diagonalPrint(root);
}
}
|
Javascript
<script>
class Node
{
constructor(data) {
this .left = null ;
this .right = null ;
this .data = data;
}
}
function newNode(data)
{
let node = new Node(data);
return (node);
}
function diagonalPrint(root)
{
if (root == null )
return ;
let q= [];
q.push(root);
q.push( null );
while (q.length>0) {
let temp = q[0];
q.shift();
if (temp == null ) {
if (q.length==0)
return ;
document.write( "</br>" );
q.push( null );
}
else {
while (temp!= null ) {
document.write( temp.data + " " );
if (temp.left!= null )
q.push(temp.left);
temp = temp.right;
}
}
}
}
let root = newNode(8);
root.left = newNode(3);
root.right = newNode(10);
root.left.left = newNode(1);
root.left.right = newNode(6);
root.right.right = newNode(14);
root.right.right.left = newNode(13);
root.left.right.left = newNode(4);
root.left.right.right = newNode(7);
diagonalPrint(root);
</script>
|
Output
8 10 14
3 6 7 13
1 4
Time Complexity: O(n), where n is the total number of nodes in the binary tree.
Auxiliary Space: O(n), As we use a queue to store the nodes, the space complexity is also O(n).
Method: Without using a delimiter
Just like level order traversal, use a queue. Little modification is to be done.
if(curr.left != null) -> add it to the queue
and move curr pointer to right of curr.
if curr = null, then remove a node from queue.
Implementation:
C++
#include <bits/stdc++.h>
using namespace std;
struct Node {
int data;
Node *left, *right;
};
Node* newNode( int data)
{
Node* node = (Node*) malloc ( sizeof (Node));
node->data = data;
node->left = node->right = NULL;
return (node);
}
Node* root;
void traverse()
{
if (root == NULL)
return ;
Node* curr = root;
queue<Node*> q;
while (!q.empty() || curr != NULL) {
if (curr != NULL) {
cout << curr->data << " " ;
if (curr->left != NULL)
q.push(curr->left);
curr = curr->right;
}
else {
curr = q.front();
q.pop();
}
}
}
int main()
{
root = newNode(8);
root->left = newNode(3);
root->right = newNode(10);
root->left->left = newNode(1);
root->left->right = newNode(6);
root->right->right = newNode(14);
root->right->right->left = newNode(13);
root->left->right->left = newNode(4);
root->left->right->right = newNode(7);
traverse();
}
|
Java
import java.util.Queue;
import java.util.LinkedList;
class Node{
int data;
Node left;
Node right;
Node( int data){
this .data = data;
left = right = null ;
}
}
public class DiagonalTraversal {
Node root = null ;
void traverse() {
if (root == null )
return ;
Node curr = root;
Queue<Node> q = new LinkedList<>();
while (!q.isEmpty() || curr!= null ) {
if (curr != null ) {
System.out.print(curr.data+ " " );
if (curr.left != null )
q.add(curr.left);
curr = curr.right;
}
else {
curr = q.remove();
}
}
}
public static void main(String args[]) {
DiagonalTraversal tree = new DiagonalTraversal();
tree.root = new Node( 8 );
tree.root.left = new Node( 3 );
tree.root.right = new Node( 10 );
tree.root.left.left = new Node( 1 );
tree.root.left.right = new Node( 6 );
tree.root.right.right = new Node( 14 );
tree.root.right.right.left = new Node( 13 );
tree.root.left.right.left = new Node( 4 );
tree.root.left.right.right = new Node( 7 );
tree.traverse();
}
}
|
Python3
class Node:
def __init__( self , data):
self .data = data
self .left = None
self .right = None
root = Node( 0 )
def traverse():
if root is None :
return
curr = root
q = []
while ( len (q)! = 0 or curr ! = None ):
if (curr ! = None ):
print (curr.data,end = " " )
if (curr.left ! = None ):
q.append(curr.left)
curr = curr.right
else :
curr = q.pop( 0 )
root = Node( 8 )
root.left = Node( 3 )
root.right = Node( 10 )
root.left.left = Node( 1 )
root.left.right = Node( 6 )
root.right.right = Node( 14 )
root.right.right.left = Node( 13 )
root.left.right.left = Node( 4 )
root.left.right.right = Node( 7 )
traverse()
|
C#
using System;
using System.Collections.Generic;
public class Node
{
public int data;
public Node left;
public Node right;
public Node( int data)
{
this .data = data;
left = right = null ;
}
}
public class DiagonalTraversal{
Node root = null ;
void traverse()
{
if (root == null )
return ;
Node curr = root;
Queue<Node> q = new Queue<Node>();
while (q.Count != 0 || curr != null )
{
if (curr != null )
{
Console.Write(curr.data + " " );
if (curr.left != null )
q.Enqueue(curr.left);
curr = curr.right;
}
else
{
curr = q.Dequeue();
}
}
}
static public void Main()
{
DiagonalTraversal tree = new DiagonalTraversal();
tree.root = new Node(8);
tree.root.left = new Node(3);
tree.root.right = new Node(10);
tree.root.left.left = new Node(1);
tree.root.left.right = new Node(6);
tree.root.right.right = new Node(14);
tree.root.right.right.left = new Node(13);
tree.root.left.right.left = new Node(4);
tree.root.left.right.right = new Node(7);
tree.traverse();
}
}
|
Javascript
<script>
class Node
{
constructor(data)
{
this .data = data;
this .left = this .right = null ;
}
}
let root = null ;
function traverse()
{
if (root == null )
return ;
let curr = root;
let q = [];
while (q.length!=0 || curr!= null ) {
if (curr != null ) {
document.write(curr.data+ " " );
if (curr.left != null )
q.push(curr.left);
curr = curr.right;
}
else {
curr = q.shift();
}
}
}
root = new Node(8);
root.left = new Node(3);
root.right = new Node(10);
root.left.left = new Node(1);
root.left.right = new Node(6);
root.right.right = new Node(14);
root.right.right.left = new Node(13);
root.left.right.left = new Node(4);
root.left.right.right = new Node(7);
traverse();
</script>
|
Output
8 10 14 3 6 7 13 1 4
Time Complexity: O(n), As we are traversing every element only once.
Auxiliary Space: O(n), Extra space is used to store the elements in the queue.
Share your thoughts in the comments
Please Login to comment...