Substrings: A Comprehensive Guide
Last Updated :
29 Apr, 2024
Substrings are a fundamental concept in computer science and programming. They play a crucial role in various applications, from text manipulation to data processing. In this blog post, we’ll explore what substrings are, their full form, use cases, examples, when to use them, when to avoid them, best practices, common problems, and other relevant information.
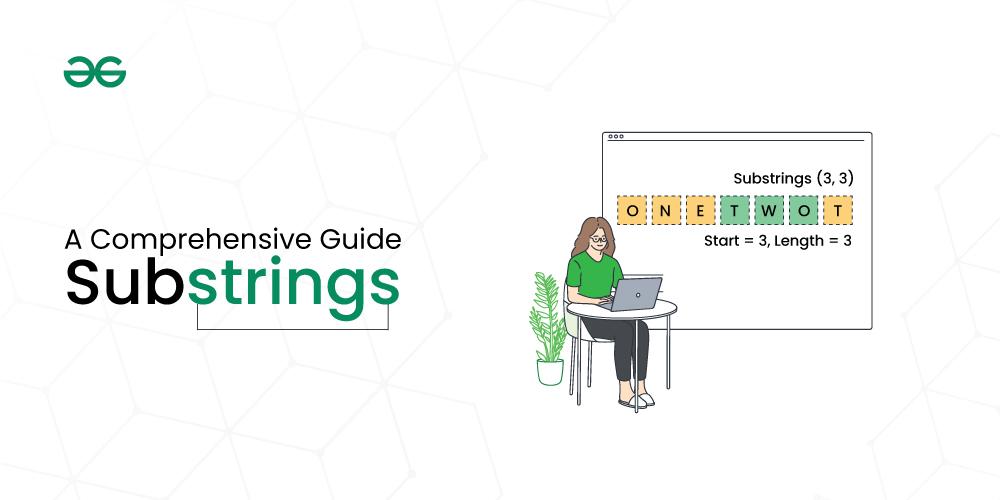
Substrings: A Comprehensive Guide
Definition: A substring is a contiguous sequence of characters within a larger string. It is essentially a smaller portion of a string extracted from the original string. Substrings are often used for various text manipulation tasks, including searching, comparing, and extracting data.
Full-Form: Substring is a portmanteau of “sub” and “string,” implying a part of a string.
2. Use Cases of Substring
Substrings find applications in a wide range of domains, including:
- Text Processing: Searching for specific words or patterns within a text.
- Data Extraction: Extracting relevant information from structured data (e.g., extracting a date from a string containing various information).
- String Manipulation: Modifying a string by replacing or deleting a part of it.
- Pattern Matching: Identifying patterns in sequences of characters.
- Data Validation: Verifying whether a string conforms to a specific format or structure.
- Programming: Splitting strings into substrings to process and manipulate data.
3. Examples of Substring
Let’s consider a few examples to illustrate the concept of substrings:
Example 1: Text Processing
C++
#include <iostream>
#include <string>
int main() {
// Original string
std::string originalString = "Hello, World!";
// Extract the substring "Hello" using the substr() method
std::string substring = originalString.substr(0, 5);
// Print the extracted substring
std::cout << substring << std::endl; // Output: Hello
return 0;
}
//This code is contributed by Utkarsh.
Java
public class Main {
public static void main(String[] args) {
// Original string
String originalString = "Hello, World!";
// Extract the substring "Hello" using the substring() method
String substring = originalString.substring(0, 5);
// Print the extracted substring
System.out.println(substring); // Output: Hello
}
}
Python3
original_string = "Hello, World!"
# Extract the substring "Hello"
substring = original_string[0:5]
print(substring) # Output: Hello
JavaScript
// Original string
let originalString = "Hello, World!";
// Extract the substring "Hello" using the substr() method
let substring = originalString.substr(0, 5);
// Print the extracted substring
console.log(substring); // Output: Hello
Example 2: Data Extraction
C++
#include <iostream>
#include <string>
int main() {
std::string data = "Name: John Doe, Age: 30, Location: New York";
// Find the starting position of the name
size_t nameStart = data.find("Name: ") + 6;
// Find the ending position of the name
size_t nameEnd = data.find(", Age: ", nameStart);
// Extract the name substring
std::string name = data.substr(nameStart, nameEnd - nameStart);
std::cout << name << std::endl; // Output should be "John Doe"
return 0;
}
//This code is contributed by Kishan.
Java
public class Main {
public static void main(String[] args) {
String data = "Name: John Doe, Age: 30, Location: New York";
// Find the starting position of the name
int nameStart = data.indexOf("Name: ") + 6;
// Find the ending position of the name
int nameEnd = data.indexOf(", Age: ", nameStart);
// Extract the name substring
String name = data.substring(nameStart, nameEnd);
System.out.println(name); // Output should be "John Doe"
}
}
//This code is contribited by Adarsh.
Python3
data = "Name: John Doe, Age: 30, Location: New York"
# Extracts the name "John Doe"
name = data[data.index("Name: ") + 6:data.index(", Age: ")]
C#
using System;
class MainClass {
public static void Main (string[] args) {
string data = "Name: John Doe, Age: 30, Location: New York";
// Find the starting position of the name
int nameStart = data.IndexOf("Name: ") + 6;
// Find the ending position of the name
int nameEnd = data.IndexOf(", Age: ", nameStart);
// Extract the name substring
string name = data.Substring(nameStart, nameEnd - nameStart);
Console.WriteLine(name); // Output should be "John Doe"
}
}
Javascript
let data = "Name: John Doe, Age: 30, Location: New York";
// Extracts the name "John Doe"
let name = data.substring(data.indexOf("Name: ") + 6, data.indexOf(", Age: "));
console.log(name); // Output should be "John Doe"
Example 3: String Manipulation
Python3
original_string = "Good morning, sunshine!"
modified_string = original_string.replace("morning", "evening")
print(modified_string)
JavaScript
let originalString = "Good morning, sunshine!";
let modifiedString = originalString.replace("morning", "evening");
console.log(modifiedString);
OutputGood evening, sunshine!
4. When to Use Substrings
You should consider using substrings when:
- You need to extract or manipulate a specific part of a larger string.
- Searching for specific patterns, keywords, or values within a string.
- Parsing structured data where relevant information is embedded in a larger text.
- Implementing algorithms that require sliding windows or comparisons.
5. When Not to Use Substrings
Avoid using substrings in the following scenarios:
- When the position of the substring is dynamic or uncertain, as this may lead to errors.
- In performance-critical applications, excessive substring operations can be inefficient.
- When working with binary data or non-textual data, as substrings are primarily designed for text.
6. Best Practices
To make the most of substrings, consider these best practices:
- Ensure you have boundary checks to prevent out-of-range errors.
- Use libraries or built-in functions for substring operations to handle edge cases.
- Minimize unnecessary substring operations for better performance.
- Be cautious when working with non-ASCII characters and character encodings, as substrings may behave differently.
7. How to use Substring in Different Programming languages
C++
#include <iostream>
#include <string>
using namespace std;
// Drivers code
int main()
{
string original_string = "Hello, World!";
string substring = original_string.substr(0, 5);
cout << "Substring: " << substring << endl;
return 0;
}
C
#include <stdio.h>
#include <string.h>
int main()
{
char original_string[] = "Hello, World!";
char substring[6];
strncpy(substring, original_string, 5);
substring[5] = '\0';
printf("Substring: %s\n", substring);
return 0;
}
Java
public class SubstringExample {
public static void main(String[] args) {
String originalString = "Hello, World!";
String substring = originalString.substring(0, 5);
System.out.println("Substring: " + substring);
}
}
Python3
original_string = "Hello, World!"
substring = original_string[0:5]
print("Substring:", substring)
C#
using System;
class Program
{
static void Main()
{
// Original string
string originalString = "Hello, World!";
// Extracting a substring from the original string
// Starting from index 0 and taking 5 characters
string substring = originalString.Substring(0, 5);
// Printing the extracted substring
Console.WriteLine("Substring: " + substring);
}
}
Javascript
let originalString = "Hello, World!";
let substring = originalString.substring(0, 5);
console.log("Substring: " + substring);
8. Problems on Substring:
Problem
| Link of the problem
|
---|
Number of substrings of one string present in other
| Read
|
Print all substring of a number without any conversion
| Read
|
Substring Reverse Pattern
| Read
|
Find the count of palindromic sub-string of a string in its sorted form
| Read
|
Check if a string contains a palindromic sub-string of even length
| Read
|
Longest sub string of 0’s in a binary string which is repeated K times
| Read
|
Longest substring with atmost K characters from the given set of characters
| Read
|
Lexicographically all Shortest Palindromic Substrings from a given string
| Read
|
Shortest Palindromic Substring
| Read
|
Count of all unique substrings with non-repeating characters
| Read
|
Count of substrings of length K with exactly K distinct characters
| Read
|
Count of substrings containing only the given character
| Read
|
Count of Distinct Substrings occurring consecutively in a given String
| Read
|
Check if a String contains Anagrams of length K which does not contain the character X
| Read
|
9. Common Problems and Solutions
Problem 1: Off-by-One Errors
Off-by-one errors are common when working with substrings. To avoid them, carefully manage your index positions, and remember that indexing is typically zero-based.
Problem 2: Inefficient Substring Operations
If you need to extract multiple substrings from a large string, consider using regular expressions or more efficient algorithms to avoid performance issues.
Problem 3: Encoding and Character Issues
When working with different character encodings, be aware that the length of a substring may not be equal to the number of characters it contains. This can lead to unexpected behavior, so handle character encodings properly.
Conclusion:
Substrings are a fundamental tool in programming and data processing, allowing you to work with smaller, more manageable parts of larger strings. When used correctly, they can simplify complex tasks, such as text processing and data extraction. However, it’s essential to be mindful of potential issues like off-by-one errors and inefficient operations. By following best practices and understanding their use cases and limitations, you can harness the power of substrings effectively in your coding adventures.
Share your thoughts in the comments
Please Login to comment...