Integrating a ML Model with React and Flask
Last Updated :
13 Mar, 2024
Here, we have a task of how to integrate a machine learning model with React, and Flask. In this article, we will see how to integrate a machine-learning model with React and Flask.
What is the Car Prediction Model?
A car price prediction model is designed to predict selling prices based on specific user input parameters. These essential parameters include Year of Purchase, Present Price, Kilometers Driven, Fuel Type, Seller Type, Transmission Type, and No. of Owners. Our main focus here is on integrating this machine learning model into a web application using React and Flask.
Car Prediction System with React and Flask
Below, is the guide to Integrating a Machine Learning Model with React and Flask using a car prediction model. Here, we will cover the article in 2 parts, frontend and then backend.
Prerequisite: Flask, React, and Machine Learning
Creating a Backend for Integrating an ML Model
To start the project and create the backend in Flask, first, open the terminal and install the flask :
pip install flask
cd backend
File Structure
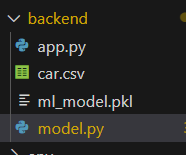
Setting Necessary Files
model.py: Below, Python code begins by importing necessary libraries and reading a car dataset from a CSV file. It preprocesses the data by encoding categorical columns and then prepares the feature matrix (X) and target variable (Y). The dataset is split into training and testing sets, allocating 10% for testing with a specified random seed. Subsequently, a Lasso Regression model is initialized and trained on the training data.
Download car.csv file by click here.
Python3
# Import necessary libraries
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import Lasso
import pickle
# Load the dataset
car_dataset = pd.read_csv('car data.csv')
# Encoding Categorical Columns
car_dataset.replace({'Fuel_Type': {'Petrol': 0, 'Diesel': 1, 'CNG': 2},
'Seller_Type': {'Dealer': 0, 'Individual': 1},
'Transmission': {'Manual': 0, 'Automatic': 1}}, inplace=True)
# Prepare Features (X) and Target Variable (Y)
X = car_dataset.drop(['Car_Name', 'Selling_Price'], axis=1)
Y = car_dataset['Selling_Price']
# Train-Test Split
X_train, X_test, Y_train, Y_test = train_test_split(X, Y, test_size=0.1, random_state=2)
# Initialize and Train the Lasso Regression Model
lr = Lasso()
lr.fit(X_train, Y_train)
# Export the Trained Model using Pickle
pickle.dump(lr, open('ml_model.pkl', 'wb'))
app.py :Below Flask API deploys a pre-trained Lasso Regression model for predictions. It uses Flask with CORS support, pandas for data handling, and Pickle to load the model. The ‘/predict‘ route accepts JSON data via POST requests, makes predictions, and returns results. The root route (‘/’) confirms the API is running. When executed, the app runs in debug mode on port 5000.
To use the machine learning model in our project, we need to install certain libraries. To do so, please execute the command provided below:
pip install pandas
pip install sklearn
pip install requests
Python3
from flask import Flask, request, jsonify
import pandas as pd
from flask_cors import CORS # CORS for handling Cross-Origin Resource Sharing
import pickle
# Create a Flask application instance
app = Flask(__name__)
# Enable CORS for all routes, allowing requests from any origin
CORS(app,resources={r"/*":{"origins":"*"}})
model = pickle.load(open('ml_model.pkl', 'rb'))
# Define a route for handling HTTP GET requests to the root URL
@app.route('/', methods=['GET'])
def get_data():
data = {
"message":"API is Running"
}
return jsonify(data)
# Define a route for making predictions
@app.route('/predict', methods=['POST'])
def predict():
try:
data = request.get_json()
query_df = pd.DataFrame([data])
prediction = model.predict(query_df)
return jsonify({'Prediction': list(prediction)})
except Exception as e:
return jsonify({'error': str(e)})
if __name__ == '__main__':
app.run(debug=True, port=5000)
Creating Frontend for Our Car Prediction Model
To start the project in react use this command
npx create-react-app calculator_frontend
cd calculator_frontend
File Structure:
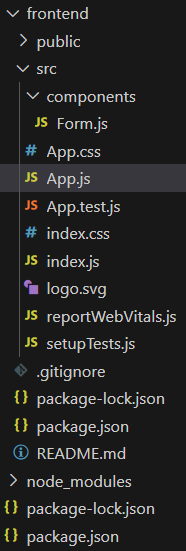
Creating User InterFace
Form.js: In below code , Form
component in React manages a form for predicting car prices. It uses state hooks to handle loading, form data, and result display. User inputs for car details trigger real-time updates via the handleChange
function. Clicking the “Predict Selling Price” button sends a POST request to a Flask backend, fetching and displaying the prediction. The UI includes input fields for key details like year, price, kilometers, fuel, seller type, transmission, and owner count.
Javascript
import React, { useState } from "react";
const Form = () => {
// State to manage loading state
const [isLoading, setIsloading] = useState(false);
// State to manage form data
const [formData, setFormData] = useState({
Year: "",
Present_Price: "",
Kms_Driven: "",
Fuel_Type: "",
Seller_Type: "",
Transmission: "",
Owner: "",
});
// State to manage prediction result
const [result, setResult] = useState("");
// State to manage displaying result
const [showSpan, setShowSpan] = useState(false);
const handleChange = (event) => {
const value = event.target.value;
const name = event.target.name;
let inputData = { ...formData };
inputData[name] = value;
setFormData(inputData);
};
// Function to handle the 'Predict Selling Price' button click
const handlePredictClick = () => {
const url = "http://localhost:5000/predict";
setIsloading(true);
const jsonData = JSON.stringify(formData);
// Fetch request to the Flask backend
fetch(url, {
headers: {
Accept: "application/json",
"Content-Type": "application/json",
},
method: "POST",
body: jsonData,
})
.then((response) => response.json())
.then((response) => {
setResult(response.Prediction);
setIsloading(false);
setShowSpan(true);
});
};
return (
<>
<div className="container text-center mt-4">
<h1 className="text-center">Car Price Prediction</h1>
<div className="container">
<form method="post" acceptCharset="utf-8" name="Modelform">
<div className="text-center mt-3">
<label>
<b>Enter Year of Purchase:</b>
</label>
<br />
<input
type="text"
className="form-control"
id="Year"
name="Year"
value={formData.Year}
onChange={handleChange}
placeholder="Enter Year of Purchase "
/>
</div>
<div className="form-group">
<label>
<b>Enter Present Price(in Lakhs):</b>
</label>
<br />
<input
type="text"
className="form-control"
id="Present_Price"
name="Present_Price"
value={formData.Present_Price}
onChange={handleChange}
placeholder="Enter Present Price(in Lakhs)"
/>
</div>
<div className="form-group">
<label>
<b>
Enter the Number of Kilometres that the car has travelled:
</b>
</label>
<br />
<input
type="text"
className="form-control"
id="Kms_Driven"
name="Kms_Driven"
value={formData.Kms_Driven}
onChange={handleChange}
placeholder="Enter the kilometres driven "
/>
</div>
<div className="form-group">
<label>
<b>Select the Fuel Type:</b>
</label>
<br />
<select
className="selectpicker form-control"
id="Fuel_Type"
name="Fuel_Type"
value={formData.Fuel_Type}
onChange={handleChange}
required
>
<option value="" disabled>
Select
</option>
<option value="0">Petrol</option>
<option value="1">Diesel</option>
<option value="2">CNG</option>
</select>
</div>
<div className="form-group">
<label>
<b>Select the Seller Type:</b>
</label>
<br />
<select
className="selectpicker form-control"
id="Seller_Type"
name="Seller_Type"
value={formData.Seller_Type}
onChange={handleChange}
required
>
<option value="" disabled>
Select
</option>
<option value="0">Dealer</option>
<option value="1">Individual</option>
</select>
</div>
<div className="form-group">
<label>
<b>Select the Transmission Type:</b>
</label>
<br />
<select
className="selectpicker form-control"
id="Transmission"
name="Transmission"
value={formData.Transmission}
onChange={handleChange}
required
>
<option value="" disabled>
Select
</option>
<option value="0">Manual</option>
<option value="1">Automatic</option>
</select>
</div>
<div className="form-group">
<label>
<b>Enter the Number of Owners:</b>
</label>
<br />
<input
type="text"
className="form-control"
id="Owner"
name="Owner"
value={formData.Owner}
onChange={handleChange}
placeholder="Enter the number of Owner "
/>
</div>
<div className="form-group mt-3">
<button
className="btn btn-primary form-control"
disabled={isLoading}
onClick={!isLoading ? handlePredictClick : null}
>
Predict Selling Price
</button>
</div>
</form>
<br />
<div className="text-center">
<h4>
{showSpan && (
<span id="prediction">
{result && Object.keys(result).length !== 0 ? (
<p>The Predicted Price is {result} Lakhs</p>
) : (
<p>Please fill out each field in the form completely</p>
)}
</span>
)}
</h4>
</div>
</div>
</div>
</>
);
};
export default Form;
App.js : The `App` component in this React application renders the main interface by including the `Form` component, which handles user inputs and predictions for car prices.
Javascript
import React from 'react'
import Form from './components/Form';
const App=()=>{
return (
<div>
<Form/>
</div>
)
}
export default App;
Deployement of the Project
Run the server with the help of following command:
python3 app.py
npm start
Output
.jpg)
Car Price Prediction Web Application using React and Flask
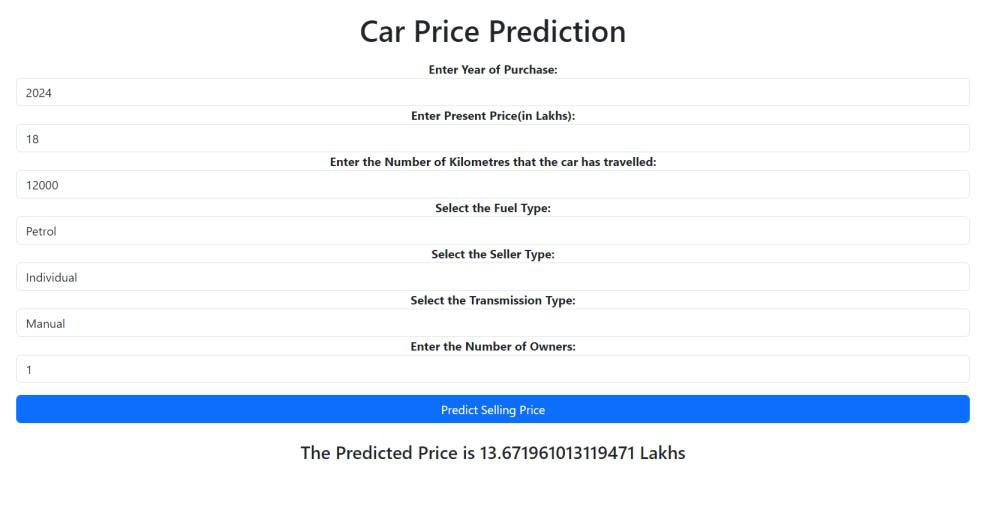
Car Price Prediction Web Application using React and Flask
Video Demonstration
Share your thoughts in the comments
Please Login to comment...