If statement in Programming
Last Updated :
09 Mar, 2024
An if statement is a fundamental control structure in programming languages that allows you to execute specific code blocks based on whether a condition is true or false. It is used to make decisions and control the flow of execution in your program.
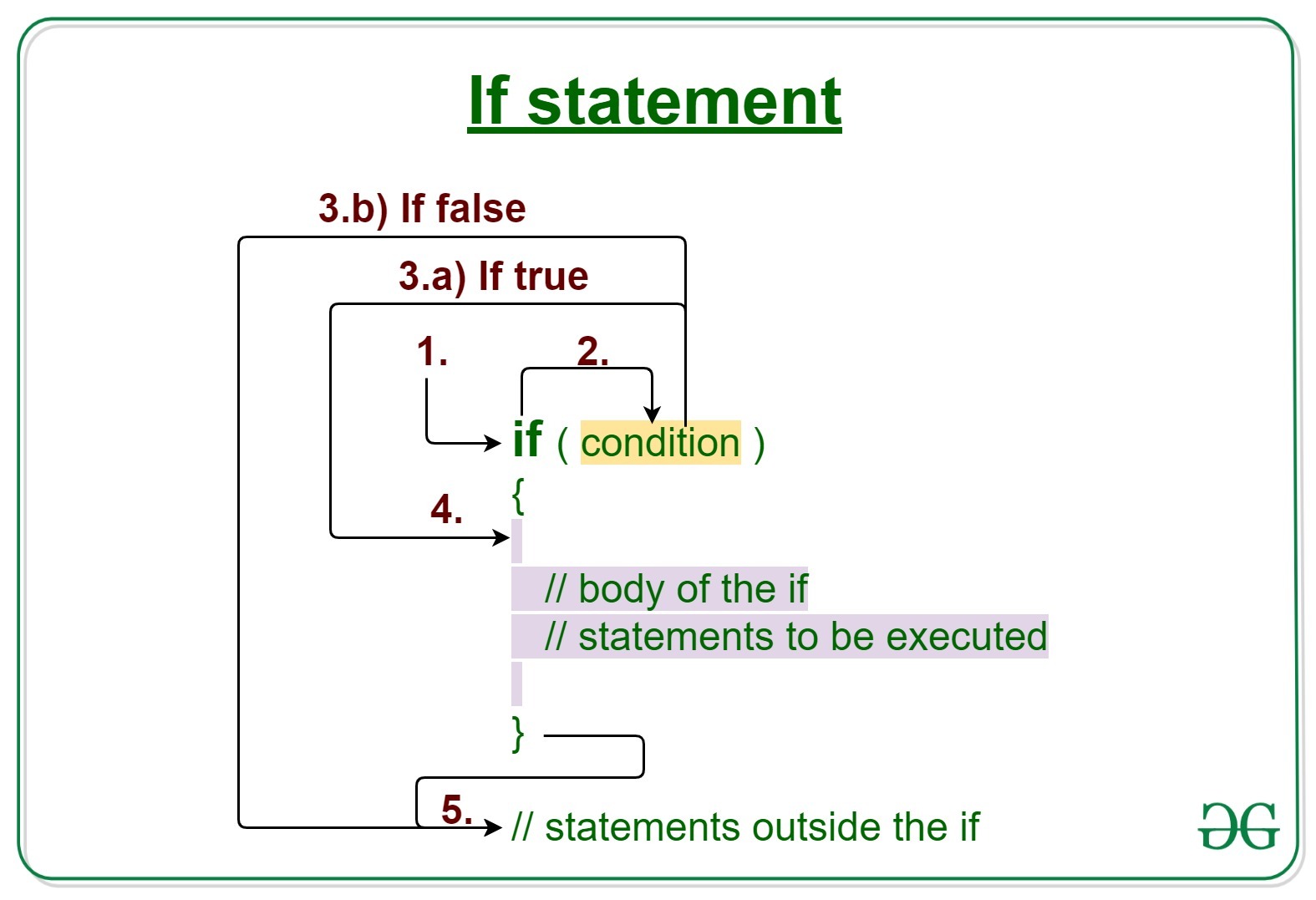
If statement in Programming
What is an if Statement?
The if statement is the most simple decision-making statement. It is used to decide whether a certain statement or block of statements will be executed or not i.e if a certain condition is true then a block of statement is executed otherwise not.
An if statement consists of two main parts:
- Condition: This is an expression that evaluates to either true or false.
- Code block: This is the code that will be executed if the condition is true.
if Statement Syntax:
The syntax of the “if” statement varies slightly across different languages, but the general syntax of an if statement in most programming languages is as follows:
Syntax
if (condition) {
/ / Code to execute if condition is true
}
|
Example of if Statement in Programming:
Let’s explore examples of “if” statements in various programming languages:
1. if Statement in C:
C
#include <stdio.h>
int main()
{
int x = 5;
if (x > 0) {
printf ("x is positive\n");
}
return 0;
}
|
Explanation: In this example, the condition x > 0 is evaluated to true because x is greater than 0. Therefore, the code inside the if block is executed, which prints “x is positive” to the console.
2. if Statement in C++:
C++
#include <iostream>
using namespace std;
int main()
{
int x = 5;
if (x > 0) {
printf ("x is positive\n");
}
return 0;
}
|
Explanation: In this example, the condition x > 0 is evaluated to true because x is greater than 0. Therefore, the code inside the if block is executed, which prints “x is positive” to the console.
3. if Statement in Java:
Java
public class Main {
public static void main(String[] args)
{
int x = 5 ;
if (x > 0 ) {
System.out.println("x is positive");
}
}
}
|
Explanation: In this example, the condition x > 0 is evaluated to true because x is greater than 0. Therefore, the code inside the first if block is executed, which prints “x is positive” to the console.
4. if Statement in Python:
Python3
x = 5
if x > 0 :
print ("x is positive")
|
Explanation: In this example, the condition x > 0 is evaluated to true because x is greater than 0. Therefore, the code inside the first if block is executed, which prints “x is positive” to the console.
5. if Statement in Javascript:
Javascript
let x = 5;
if (x > 0) {
console.log("x is positive");
}
|
Explanation: In this example, the condition x > 0 is evaluated to true because x is greater than 0. Therefore, the code inside the first if block is executed, which prints “x is positive” to the console.
6. if Statement in C#:
C#
using System;
class Program {
static void Main()
{
int x = 5;
if (x > 0) {
Console.WriteLine("x is positive");
}
}
}
|
Explanation: In this example, the condition x > 0 is evaluated to true because x is greater than 0. Therefore, the code inside the first if block is executed, which prints “x is positive” to the console.
Conditional Operators in if Statements
Conditional operators are used to compare two values and return a boolean value (true or false). The most common conditional operators are:
- == (equal to)
- != (not equal to)
- > (greater than)
- < (less than)
- >= (greater than or equal to)
- <= (less than or equal to)
Example:
C++
#include <iostream>
using namespace std;
int main()
{
int a = 5, b = 10;
if (a == b) {
cout << "a is equal to b" << endl;
}
if (a != b) {
cout << "a is not equal to b" << endl;
}
if (a > b) {
cout << "a is greater than b" << endl;
}
if (a < b) {
cout << "a is less than b" << endl;
}
if (a >= b) {
cout << "a is greater than or equal to b" << endl;
}
if (a <= b) {
cout << "a is less than or equal to b" << endl;
}
return 0;
}
|
C
#include <stdio.h>
int main()
{
int a = 5, b = 10;
if (a == b) {
printf ( "a is equal to b\n" );
}
if (a != b) {
printf ( "a is not equal to b\n" );
}
if (a > b) {
printf ( "a is greater than b\n" );
}
if (a < b) {
printf ( "a is less than b\n" );
}
if (a >= b) {
printf ( "a is greater than or equal to b\n" );
}
if (a <= b) {
printf ( "a is less than or equal to b\n" );
}
return 0;
}
|
Java
public class Main {
public static void main(String[] args)
{
int a = 5 , b = 10 ;
if (a == b) {
System.out.println( "a is equal to b" );
}
if (a != b) {
System.out.println( "a is not equal to b" );
}
if (a > b) {
System.out.println( "a is greater than b" );
}
if (a < b) {
System.out.println( "a is less than b" );
}
if (a >= b) {
System.out.println(
"a is greater than or equal to b" );
}
if (a <= b) {
System.out.println(
"a is less than or equal to b" );
}
}
}
|
Python
a = 5
b = 10
if a = = b:
print ( "a is equal to b" )
if a ! = b:
print ( "a is not equal to b" )
if a > b:
print ( "a is greater than b" )
if a < b:
print ( "a is less than b" )
if a > = b:
print ( "a is greater than or equal to b" )
if a < = b:
print ( "a is less than or equal to b" )
|
C#
using System;
class Program {
static void Main()
{
int a = 5, b = 10;
if (a == b) {
Console.WriteLine( "a is equal to b" );
}
if (a != b) {
Console.WriteLine( "a is not equal to b" );
}
if (a > b) {
Console.WriteLine( "a is greater than b" );
}
if (a < b) {
Console.WriteLine( "a is less than b" );
}
if (a >= b) {
Console.WriteLine(
"a is greater than or equal to b" );
}
if (a <= b) {
Console.WriteLine(
"a is less than or equal to b" );
}
}
}
|
Javascript
let a = 5, b = 10;
if (a === b) {
console.log( "a is equal to b" );
}
if (a !== b) {
console.log( "a is not equal to b" );
}
if (a > b) {
console.log( "a is greater than b" );
}
if (a < b) {
console.log( "a is less than b" );
}
if (a >= b) {
console.log( "a is greater than or equal to b" );
}
if (a <= b) {
console.log( "a is less than or equal to b" );
}
|
Output
a is not equal to b
a is less than b
a is less than or equal to b
Common Mistakes to Avoid:
- Missing Parentheses: Always put conditions inside parentheses when using if statements. Forgetting them can make your code confusing or cause errors.
- Mixing up Assignment and Comparison operator: Don’t use the single equals sign
=
in conditions. Use ==
to check if things are equal. Mixing them up can make your program behave unexpectedly.
- Forgetting Curly Braces: Even for short if statements, it’s safer to use curly braces
{}
. Forgetting them might cause issues, especially when you add more lines of code later.
- Too Many Nested Ifs: Avoid putting if statements inside other if statements too much. It can make your code hard to understand. Try to simplify by using logical operators like
&&
and ||
.
- Forgetting Special Cases: Make sure your if statements cover all possible situations. Test them with different values to be sure they work correctly.
Best Practices for Using if Statements:
- Clear Conditions: Write if statements that are easy to understand. Use clear variable names and comments to explain what each condition does.
- Consistent Formatting: Keep your if statements looking the same throughout your code. Use the same amount of space and curly braces to make it easier to read.
- Avoid Redundancy: Don’t repeat conditions that don’t add anything new. Keep your code simple and easy to follow.
- Logical Operators: Use
&&
, ||
, and !
to combine conditions when it makes sense. It helps make your code shorter and easier to understand.
- Hardcoding Values: Instead of putting specific numbers directly into if statements, use variables or constants. It makes your code easier to change later.
Share your thoughts in the comments
Please Login to comment...