Break vs Continue Statement in Programming
Last Updated :
24 Apr, 2024
Break and Continue statements are the keywords that are used always within a loop. The purpose of a break and continue statement is to stop a running loop or to continue a particular iteration. In this article we will see what are the break and continue statements, what is their use and what are the differences between them.
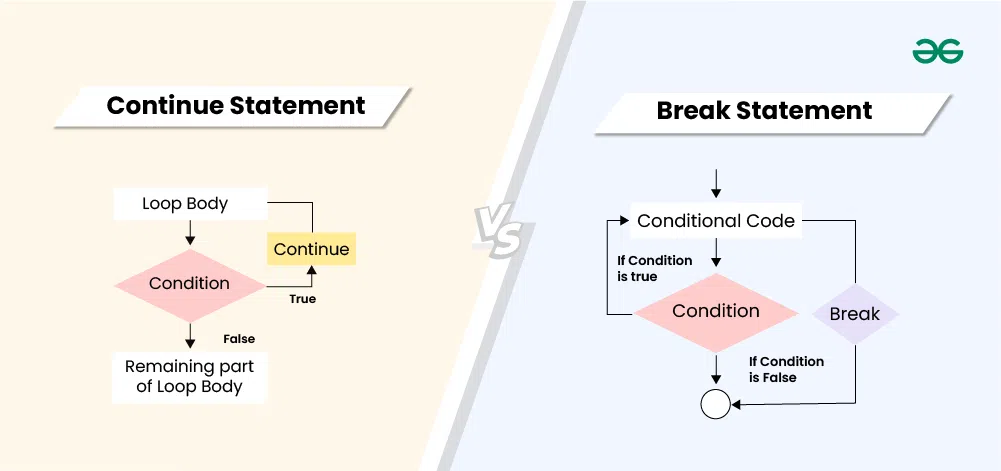
Break vs Continue Statement
Break Statement:
A break statement is used when we want to terminate the running loop whenever any particular condition occurs. Whenever a break statement occurs loop breaks and stops executing.
For example: If we have a variable curr=0 and in a while loop we are incrementing it by one each time and printing it. Now we want to stop whenever we get curr=5. So we can write a break statement when we get curr=5.
Below is the implementation of the above idea:
C++
#include <iostream>
using namespace std;
int main()
{
int curr = 0;
// Loop till curr < 10
while (curr < 10) {
cout << curr << endl;
curr++;
// If curr = 5, break out of the loop
if (curr == 5) {
break;
}
}
return 0;
}
Java
public class Main {
public static void main(String[] args)
{
int curr = 0;
// Loop till curr < 10
while (curr < 10) {
System.out.println(curr);
curr++;
// If curr = 5, break out of the loop
if (curr == 5) {
break;
}
}
}
}
Python
curr = 0
# Loop till curr < 10
while curr < 10:
print(curr)
curr += 1
# If curr = 5, break out of the loop
if curr == 5:
break
JavaScript
let curr = 0;
// Loop till curr < 10
while (curr < 10) {
console.log(curr);
curr++;
// If curr = 5, break out of the loop
if (curr === 5) {
break;
}
}
Continue Statement:
On the other side continue statement is used when we have to skip a particular iteration. Whenever we write continue statement the whole code after that statement is skipped and loop will go for next iteration.
For example: We have to print numbers form 1 to 5 but skip 3 . So we can use a for loop which will print the number and when i=3 we will continue.
Below is the implementation of the above idea:
C++
#include <iostream>
using namespace std;
int main()
{
// Loop till i <= 5
for (int i = 0; i <= 5; ++i) {
// If i = 3, then continue to the next iteration
if (i == 3) {
continue;
}
cout << i << endl;
}
return 0;
}
Java
public class Main {
public static void main(String[] args)
{
// Loop till i <= 5
for (int i = 0; i <= 5; ++i) {
// If i = 3, then continue to the next iteration
if (i == 3) {
continue;
}
System.out.println(i);
}
}
}
Python
# Loop till i <= 5
for i in range(0, 6):
# If i = 3, then continue to the next iteration
if i == 3:
continue
print(i)
JavaScript
// Loop till i <= 5
for (let i = 0; i <= 5; ++i) {
// If i = 3, then continue to the next iteration
if (i === 3) {
continue;
}
console.log(i);
}
Break vs Continue Statement:
Break Statement | Continue Statement |
---|
The Break statement is used to exit from the loop constructs. | The continue statement is not used to exit from the loop constructs. |
The break statement is usually used with the switch statement, and it can also use it within the while loop, do-while loop, or the for-loop. | The continue statement is not used with the switch statement, but it can be used within the while loop, do-while loop, or for-loop. |
When a break statement is encountered then the control is exited from the loop construct immediately. | When the continue statement is encountered then the control automatically passed to the beginning of the loop statement. |
Syntax: break; | Syntax: continue; |
Break statements uses switch and label statements. | It does not use switch and label statements. |
Leftover iterations are not executed after the break statement. | Leftover iterations can be executed even if the continue keyword appears in a loop. |
Share your thoughts in the comments
Please Login to comment...