HTML | DOM Style borderTopWidth Property
Last Updated :
09 Aug, 2022
The Style borderTopWidth property in HTML DOM is used to set or return the width of the top border of an element.
Syntax:
- To get the borderTopWidth Property
object.style.borderTopWidth
- To set the borderTopWidth Property
object.style.borderTopWidth = "thin | medium | thick | length |
initial | inherit"
Return Values: It returns a string value, which representing the width of an element’s top border.
Property Values:
1. thin: This is used to define a thin top border.
Example:
html
<!DOCTYPE html>
< html lang="en">
< head >
< title >
DOM Style BorderTopWidth
</ title >
< style >
.elem {
border-style: solid;
padding: 10px;
}
</ style >
</ head >
< body >
< h1 style="color: green">
GeeksforGeeks
</ h1 >
< b >
DOM Style BorderTopWidth
</ b >
< p class="elem">
GeeksforGeeks is a computer science
portal with a huge variety of well
written and explained computer
science and programming articles,
quizzes and interview questions.
</ p >
< button onclick="changeWidth()">
Change borderTopWidth
</ button >
< script >
function changeWidth() {
elem = document.querySelector('.elem');
elem.style.borderTopWidth = 'thin';
}
</ script >
</ body >
</ html >
|
Output:
Before clicking the button:
After clicking the button:
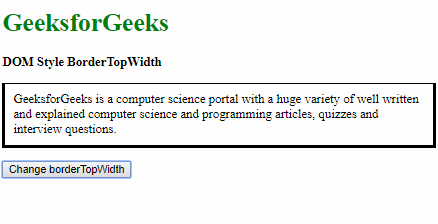
2. medium: This is used to define a medium top border. This is the default value.
Example:
html
<!DOCTYPE html>
< html lang="en">
< head >
< title >
DOM Style BorderTopWidth
</ title >
< style >
.elem {
border: thick solid;
padding: 10px;
}
</ style >
</ head >
< body >
< h1 style="color: green">
GeeksforGeeks
</ h1 >
< b >
DOM Style BorderTopWidth
</ b >
< p class="elem">
GeeksforGeeks is a computer
science portal with a huge
variety of well written and
explained computer science
and programming articles,
quizzes and interview questions.
</ p >
< button onclick="changeWidth()">
Change borderTopWidth
</ button >
< script >
function changeWidth() {
elem = document.querySelector('.elem');
elem.style.borderTopWidth = 'medium';
}
</ script >
</ body >
</ html >
|
Output:
Before clicking the button:
After clicking the button:
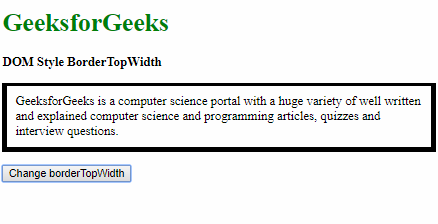
3. thick: This is used to define a thick top border.
Example-3:
html
<!DOCTYPE html>
< html lang="en">
< head >
< title >
DOM Style BorderTopWidth
</ title >
< style >
.elem {
border-style: solid;
padding: 10px;
}
</ style >
</ head >
< body >
< h1 style="color: green">
GeeksforGeeks
</ h1 >
< b >
DOM Style BorderTopWidth
</ b >
< p class="elem">
GeeksforGeeks is a computer
science portal with a huge
variety of well written and
explained computer science
and programming articles,
quizzes and interview questions.
</ p >
< button onclick="changeWidth()">
Change borderTopWidth
</ button >
< script >
function changeWidth() {
elem = document.querySelector('.elem');
elem.style.borderTopWidth = 'thick';
}
</ script >
</ body >
</ html >
|
Output:
Before clicking the button:
After clicking the button:
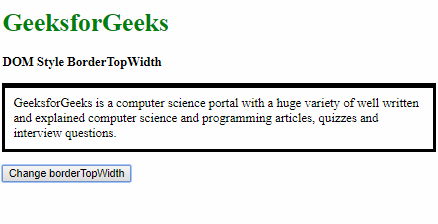
4. length: This is used to define the top border width in terms of length units.
Example-4:
html
<!DOCTYPE html>
< html lang="en">
< head >
< title >
DOM Style BorderTopWidth
</ title >
< style >
.elem {
border-style: solid;
padding: 10px;
}
</ style >
</ head >
< body >
< h1 style="color: green">
GeeksforGeeks
</ h1 >
< b >
DOM Style BorderTopWidth
</ b >
< p class="elem">
GeeksforGeeks is a computer science
portal with a huge variety of well
written and explained computer science
and programming articles, quizzes and
interview questions.
</ p >
< button onclick="changeWidth()">
Change borderTopWidth
</ button >
< script >
function changeWidth() {
elem = document.querySelector('.elem');
elem.style.borderTopWidth = '10px';
}
</ script >
</ body >
</ html >
|
Output:
Before clicking the button:
After clicking the button:
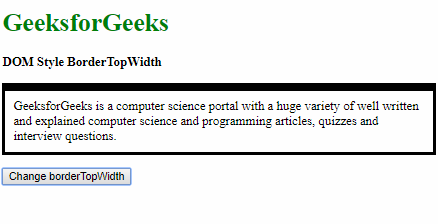
5. initial: This is used to set this property to its default value.
Example-5:
html
<!DOCTYPE html>
< html lang="en">
< head >
< title >
DOM Style BorderTopWidth
</ title >
< style >
.elem {
border-style: solid;
padding: 10px;
border-top-width: 2px;
}
</ style >
</ head >
< body >
< h1 style="color: green">
GeeksforGeeks
</ h1 >
< b >
DOM Style BorderTopWidth
</ b >
< p class="elem">
GeeksforGeeks is a computer science
portal with a huge variety of well
written and explained computer
science and programming articles,
quizzes and interview questions.
</ p >
< button onclick="changeWidth()">
Change borderTopWidth
</ button >
< script >
function changeWidth() {
elem = document.querySelector('.elem');
elem.style.borderTopWidth = 'initial';
}
</ script >
</ body >
</ html >
|
Output:
Before clicking the button:
After clicking the button:
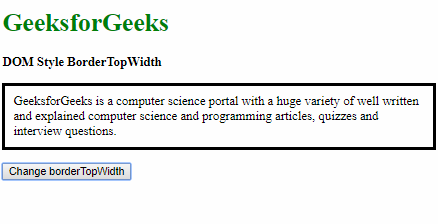
6. inherit: This inherits the property from its parent.
Example-6:
html
<!DOCTYPE html>
< html lang="en">
< head >
< title >
DOM Style BorderTopWidth
</ title >
< style >
#parent {
padding: 10px;
border-style: solid;
/* Setting the borderTopWidth
of the parent */
border-top-width: 15px;
}
.elem {
border-style: solid;
padding: 10px;
}
</ style >
</ head >
< body >
< h1 style="color: green">
GeeksforGeeks
</ h1 >
< b >
DOM Style BorderTopWidth
</ b >
< br >
< br >
< div id="parent">
< p class="elem">
GeeksforGeeks is a computer science
portal with a huge variety of well
written and explained computer science
and programming articles, quizzes and
interview questions.
</ p >
</ div >
< br >
< button onclick="changeWidth()">
Change borderTopWidth
</ button >
< script >
function changeWidth() {
elem = document.querySelector('.elem');
elem.style.borderTopWidth = 'inherit';
}
</ script >
</ body >
</ html >
|
Output:
Before clicking the button:
After clicking the button:
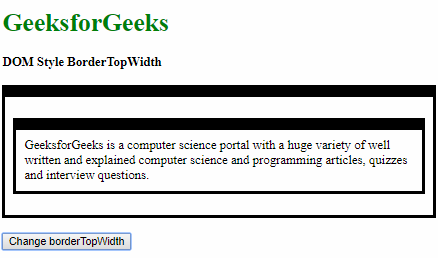
Supported Browsers: The browser supported by borderTopWidth property are listed below:
- Google Chrome 1
- Edge 12
- Internet Explorer 4
- Firefox 1
- Opera 3.5
- Apple Safari 1
Share your thoughts in the comments
Please Login to comment...