How to zoom-in and zoom-out image using JavaScript ?
Last Updated :
13 Dec, 2023
Given an image, the task is to increase and decrease the image size using JavaScript. Use the following property to increase and decrease the image.
These are the following ways:
Using Width property
- It is used to change the new values to resize the width of the element.
- Get the selector of the required image using .getElementById(selector).
- Store the current width value in the variable using .clientWidth.
- Now change the width value to new using .style.width.
- It will proportionally increase and decrease the dimension of an image.
Syntax:
object.style.width = "auto|length|%|initial|inherit";
Example:
html
<!DOCTYPE html>
< html >
< head >
< title >
How to zoom-in and zoom-out
image using JavaScript ?
</ title >
< script src =
</ script >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< h3 >
JavaScript | Increase and
Decrease image size
</ h3 >
< hr >
< div class = "box" >
< img src =
id = "geeks" GFG = "250"
alt = "Geeksforgeeks" >
</ div >
< hr >
< button type = "button" onclick = "zoomin()" >
Zoom-In
</ button >
< button type = "button" onclick = "zoomout()" >
Zoom-Out
</ button >
< script type = "text/javascript" >
function zoomin() {
let GFG = document.getElementById("geeks");
let currWidth = GFG.clientWidth;
GFG.style.width = (currWidth + 100) + "px";
}
function zoomout() {
let GFG = document.getElementById("geeks");
let currWidth = GFG.clientWidth;
GFG.style.width = (currWidth - 100) + "px";
}
</ script >
</ body >
</ html >
|
Output:
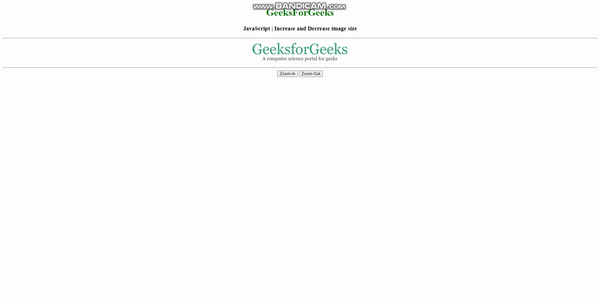
Output
Using Height property
- It is used to change the new values to resize the height of the element.
- Get the selector of the required image using .getElementById(selector).
- Store the current height value in the variable using .clientHeight.
- Now change the width value to new using .style.height.
- It will proportionally increase and decrease the dimension of an image.
Syntax:
object.style.height = "auto|length|%|initial|inherit"
Example:
html
<!DOCTYPE html>
< html >
< head >
< title >
How to zoom-in and zoom-out
image using JavaScript ?
</ title >
< script src =
</ script >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< h3 >
JavaScript | Increase and
Decrease image size
</ h3 >
< hr >
< div class = "box" >
< img src =
id = "geeks" GFG = "250"
alt = "Geeksforgeeks" >
</ div >
< hr >
< button type = "button" onclick = "zoomin()" >
Zoom-In
</ button >
< button type = "button" onclick = "zoomout()" >
Zoom-Out
</ button >
< script type = "text/javascript" >
function zoomin() {
let GFG = document.getElementById("geeks");
let currHeight = GFG.clientHeight;
GFG.style.height = (currHeight + 40) + "px";
}
function zoomout() {
let GFG = document.getElementById("geeks");
let currHeight = GFG.clientHeight;
GFG.style.height = (currHeight - 40) + "px";
}
</ script >
</ body >
</ html >
|
Output:
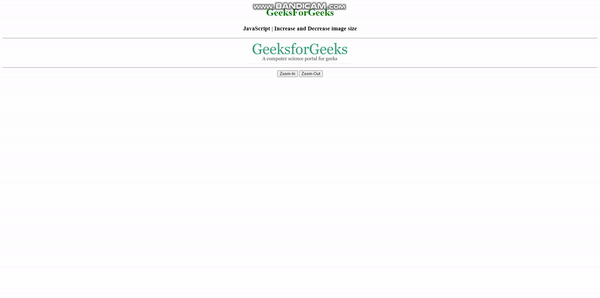
Output
Share your thoughts in the comments
Please Login to comment...