How to use Underscore.js Templates in Conjunction with EJS ?
Last Updated :
15 Apr, 2024
Underscore.js is one of the most famous javascript libraries that provides a set of template literals, which allow you to create reusable HTML templates using a simple syntax. EJS (Embedded JavaScript) is a server-side templating language that allows you to embed JavaScript code in HTML templates. Using Underscore.js templates in conjunction with EJS can be useful when you want the presentation logic from the business logic of your application.
Steps to Create NodeJS App & Installing Module:
Step 1: Create a new directory for your project:
mkdir ejs-underscore
Step 2: Navigate into the project directory:
cd ejs-underscore
Step 3: Initialize npm (Node Package Manager) to create a package.json file:
npm init -y
Step 4: Install required modules (Express and EJS) using npm:
npm install express ejs
Project Structure:
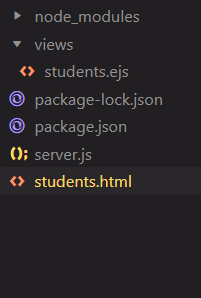
project_structure
The updated dependencies in package.json file will look like:
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.2"
}
Example: Below is an example of using underscore.js templates in conjunction with EJS.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Students Table</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
margin: 0;
padding: 0;
}
.container {
max-width: 800px;
margin: 50px auto;
padding: 20px;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h1 {
color: green;
text-align: center;
margin-bottom: 20px;
}
table {
width: 100%;
border-collapse: collapse;
margin-top: 20px;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
}
</style>
</head>
<body>
<h1>GeeksForGeeks Students Data</h1>
<table>
<thead>
<tr>
<th>No:</th>
<th>Name</th>
<th>Email</th>
<th>Enrolled Course</th>
</tr>
</thead>
<tbody>
<% students.forEach(function(student, index) { %>
<tr>
<td><%= index + 1 %></td>
<td><%= student.name %></td>
<td><%= student.email %></td>
<td><%= student.enrolledCourse %></td>
</tr>
<% }); %>
</tbody>
</table>
</body>
</html>
HTML
<!-- students.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Students Table</title>
</head>
<body>
<h1>Students Table</h1>
<table>
<thead>
<tr>
<th>Name</th>
<th>Email</th>
<th>Enrolled Course</th>
</tr>
</thead>
<tbody>
<!-- Student data will be inserted dynamically here -->
</tbody>
</table>
</body>
</html>
JavaScript
// server.js
const express = require("express");
const app = express();
const ejs = require("ejs");
const path = require("path"); // Import the path module
const port = 3000;
// Sample data
const students = [
{
name: "Aman Shrey",
email: "aman@gmail.com",
enrolledCourse: "Python",
},
{
name: "Shreya Gupta",
email: "shreya@example.com",
enrolledCourse: "Web Development",
},
{
name: "Rahul Mishra",
email: "rahul@example.com",
enrolledCourse: "DSA in Java",
},
];
app.set("view engine", "ejs");
app.listen(port, () => {
console.log(`Server is listening at http://localhost:${port}`);
});
JavaScript
// Serve static files from the 'public' directory
app.use(express.static(path.join(__dirname, "public")));
// Route to render the students.ejs template
app.get("/", (req, res) => {
res.render("students", { students: students });
});
Output:
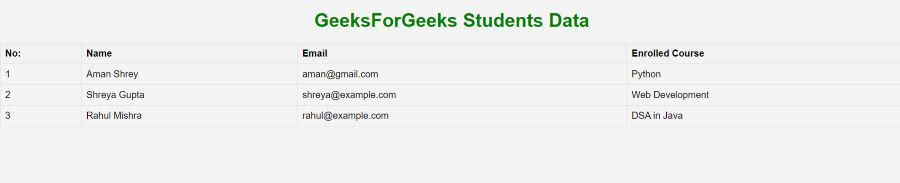
output
Share your thoughts in the comments
Please Login to comment...