How to use the app object in Express ?
Last Updated :
03 Jan, 2024
In Node and Express, the app
object has an important role as it is used to define routes and middleware, and handling HTTP requests and responses. In this article, we’ll explore the various aspects of the `app` object and how it can be effectively used in Express applications.
Prerequisites
The app
object represents the Express application. It can be created by calling the express()
function and is used to set up and configure various aspects of your server.
Syntax:
const express = require('express');
const app = express();
Different functions of the app object:
1. Defining Routes with “app”
The `app` object is to define routes for handling different HTTP methods and paths. Routes are defined using methods like GET, POST, PUT and DELETE.
Here’s a simple example:
// Handling GET requests to the root path "/"
app.get('/', (req, res) => {
res.send('Hello, World!');
});
2. Middleware with “app”
Middleware functions are functions that have access to the request (`req`), response (`res`), and the next middleware function in the application’s request-response cycle. The `app` object is used to apply middleware to your application.
Syntax:
// Logger middleware
const loggerMiddleware = (req, res, next) => {
console.log(`Received a ${req.method} request to ${req.path}`);
next();
};
// Applying the logger middleware to all routes
app.use(loggerMiddleware);
3. Setting Configuration with “app”
The `app` object allows you to configure various settings for your application, such as the view engine, port number, and more.
Syntax:
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Steps to create a sample Express app.
Step 1: Create a directory for the application
mkdir app-object
cd app-object
Step 2: Initializing the app and install the required dependencies.
npm init -y
npm i express
Example: In this sample code we have used various app objects.
Javascript
const express = require( "express" );
const app = express();
app.get( "/" , (req, res) => {
res.send( "Hello, World!" );
});
app.post( "/login" , (req, res) => {
res.send( "Login successful!" );
});
const loggerMiddleware = (req, res, next) => {
console.log(`Received a ${req.method} request to ${req.path}`);
next();
};
app.use(loggerMiddleware);
app.get( "/about" , (req, res) => {
res.send( "About Us" );
});
const PORT = process.env.PORT || 3001;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
|
Output(Browser):
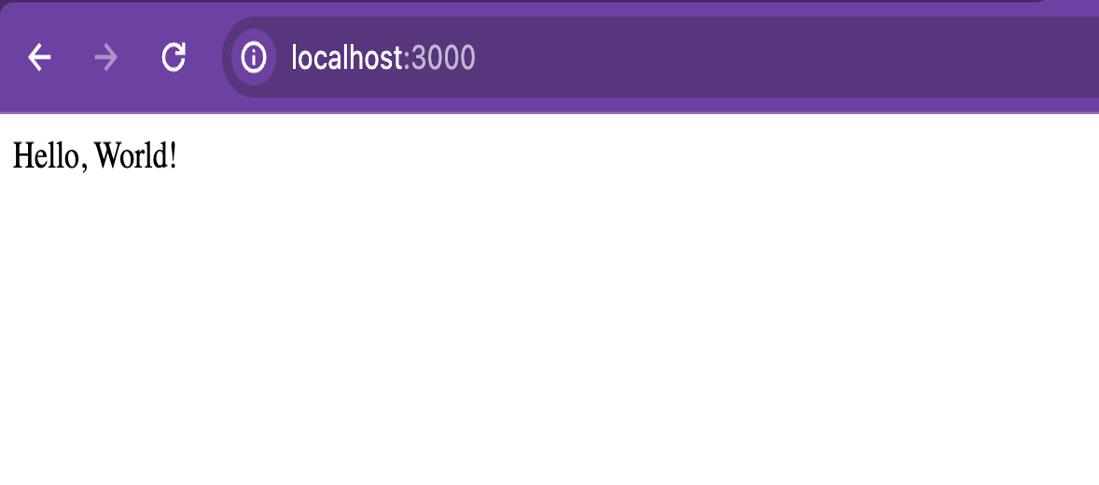
output when the “localhost:3000/” endpoint is hitted.
Output(Console):
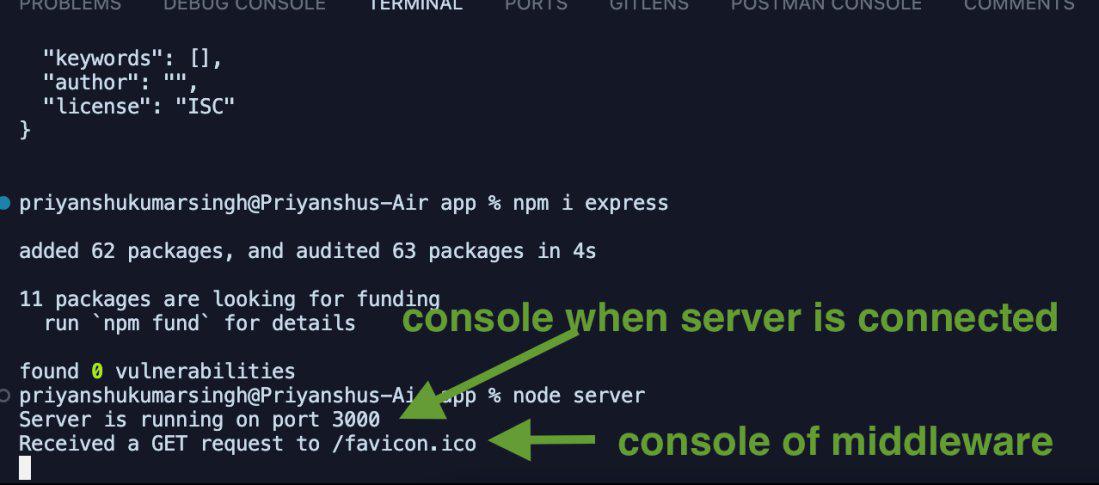
console when server is connected and when the middleware is called
Share your thoughts in the comments
Please Login to comment...