How to Add Express to React App using Gulp ?
Last Updated :
10 Nov, 2023
When building a web application with ReactJS, you may need to include a backend server for handling server-side logic and API requests. Express is a popular choice for creating backend servers in Node.js. In this tutorial, we’ll explore how to integrate Express with a React app using Gulp as the build tool. Gulp will allow us to run both the React development server and the Express server concurrently.
Prerequisites:
Gulp
Gulp is a task runner and build system for JavaScript projects. It helps automate repetitive tasks such as file minification, compilation, testing, and more. It uses a simple and intuitive syntax, allowing developers to define tasks and dependencies easily. Gulp simplifies the development process by automating common workflows, making it a popular tool among web developers.
Steps to Configure Express to a React App using Gulp
Step 1: Initialize a new React project using Create React App by running the following command in your terminal:
npx create-react-app gfg
Step 2: Move to the project directory:
cd gfg
Step 3: Install Express and Gulp by running the following command in your terminal:
npm install express gulp
Project Structure:
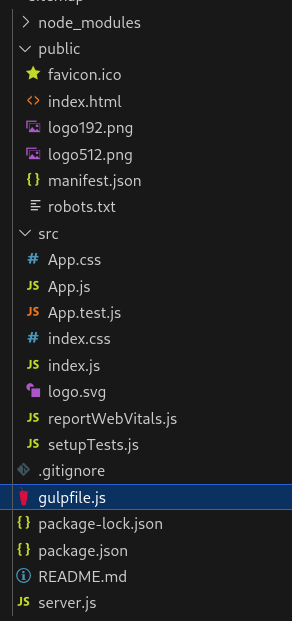
The updated dependencies after installing required modules
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"express": "^4.18.2",
"gulp": "^4.0.2",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: This example implements backend using gulp and express.
Javascript
const gulp = require( "gulp" );
const { spawn } = require( "child_process" );
gulp.task( "start-server" , () => {
const serverProcess = spawn( "node" , [ "server.js" ], {
stdio: "inherit" ,
});
serverProcess.on( "close" , (code) => {
if (code === 8) {
console.error(
"Error detected, waiting for changes..."
);
}
});
});
gulp.task(
"default" ,
gulp.series( "start-server" , () => {
const reactProcess = spawn( "npm" , [ "start" ], {
stdio: "inherit" ,
});
reactProcess.on( "close" , (code) => {
if (code === 8) {
console.error(
"Error detected, waiting for changes..."
);
}
});
})
);
|
Javascript
const express = require( "express" );
const app = express();
const port = 3001;
app.get( "/api/data" , (req, res) => {
res.json({ message: "Hello from the server!" });
});
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
|
Step to run server using gulp:
Step 1: Update the scripts: First update the “scripts” section to include a new script for running Gulp:
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject",
"gulp": "gulp"
},
Step 2 Start the backend server: Use this command in the terminal inside project directory to start the server
npm run gulp
Start frontend server: Use this command in the terminal inside project directory to start the server
npm start
Output: The React app will be available at http://localhost:3000, and you can access the Express API endpoint at http://localhost:3001/api/data. When you visit the API endpoint in your browser, you should see the JSON response { “message”: “Hello from the server!” }.
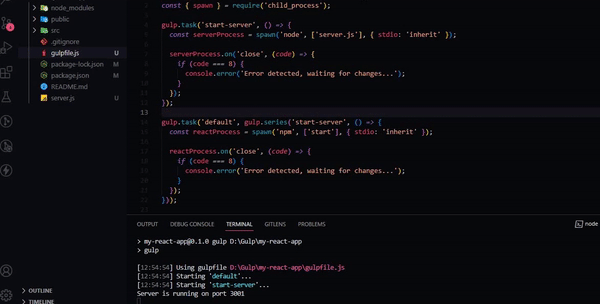
Demo
By following these steps, you’ve successfully added Express to your React app using Gulp. The React development server will run on the default port 3000, and the Express server will run on port 3001. You can modify these ports as needed in the server.js and gulpfile.js files.
Share your thoughts in the comments
Please Login to comment...