How to use jQuery with TypeScript ?
Last Updated :
21 Dec, 2023
In this article, we will learn how we can use jQuery with TypeScript and implement the features of both languages. The below approach can be used to implement jQuery in TypeScript.
By installing jQuery using the npm command
The jQuery can be installed in your current TypeScript project folder using the below commands and can be used to make changes in the application.
npm commands:
npm install jquery
npm install --save-dev @types/jquery
Once jQuery gets installed in your project use the below command to import and use it in your TypeScript file.
import variable_name_by_which_you_want_to_import_jQuery from 'jquery'
Example 1: The below code example shows how you can use jQuery in TypeScript by installing it in your project folder.
Please add the below HTML code to your index.html file.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >Using jQuery in TypeScript</ title >
</ head >
< body >
< div style = "text-align: center;" >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< button id = "buttonId" >
Click to show text using jQuery
</ button >
< h3 id = "dialog-container" ></ h3 >
</ div >
</ body >
</ html >
|
Now, add the below code to your index.ts file.
Javascript
import $ from "jquery" ;
declare let global: any;
global.jQuery = $;
$( "#buttonId" ).click(() => {
const myHTML: string = `
<b>
This text is shown using
the jQuery in TypeScript
by installing it using
npm package.
</b>`;
$( '#dialog-container' ).html(myHTML);
});
|
Output:
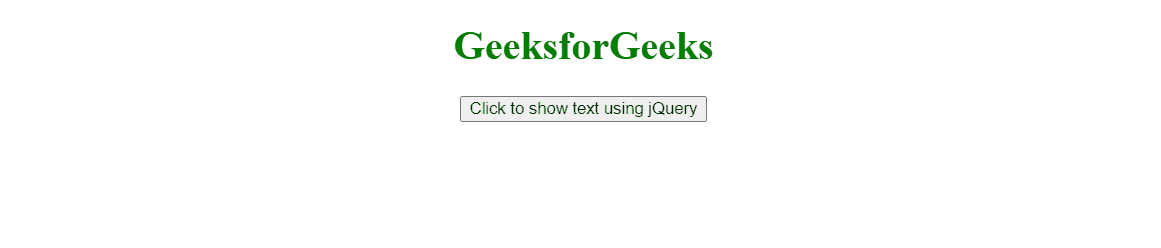
Example 2: The below example will show the advance implementation of jQuery in TypeScript.
Add the below HTML code to your index.html file.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0" />
< title >
Using jQuery in TypeScript
</ title >
</ head >
< body >
< div style = "text-align: center" >
< h1 style = "color: green" >
GeeksforGeeks
</ h1 >
< button id = "buttonId" >
Show the below text
</ button >
< h3 style = "display: none;"
id = "dialog-container" >
This text is shown using
the jQuery in TypeScript
by installing it using
npm package.
</ h3 >
</ div >
</ body >
</ html >
|
Add the below code in your index.ts file.
Javascript
import $ from 'jquery' ;
$( "#buttonId" ).click(() => {
$( '#dialog-container' ).toggle();
if ($( '#dialog-container' ).css( 'display' ) === 'none' ) {
$( '#buttonId' ).html( 'Show the below text' );
}
else {
$( '#buttonId' ).html( 'Hide the below text' );
}
});
|
Output:
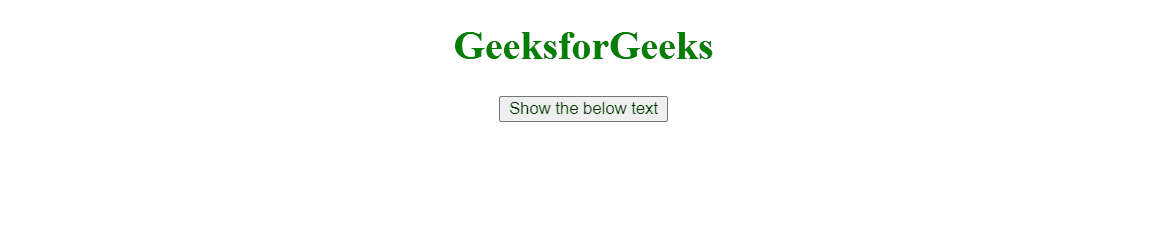
Share your thoughts in the comments
Please Login to comment...