How to use HOCs to reuse Component Logic in React ?
Last Updated :
27 Mar, 2024
In React, making reusable components keeps your code neat. Higher-order components (HOCs) are a smart way to bundle and reuse component logic. HOCs are like magic functions that take a component and give you back an upgraded version with extra powers or information.
HOCs can be implemented in a few different ways, depending on the requirements in the following ways:
1. Function that Returns a Function: This is the most common approach where the HOC is a function that accepts a component and returns a new component that renders the original component with enhanced props.
Syntax:
const withData = (WrappedComponent) => (props) => {
// Enhanced logic here
return <WrappedComponent {...props} />;
};
2.Using ES6 Classes: Sometimes, you might need the HOC to maintain its own state or lifecycle methods. In such cases, extending React.Component in the HOC can be beneficial.
Syntax:
const withSubscription = (WrappedComponent, selectData) => {
return class extends React.Component {
// Implement lifecycle methods and state here
render() {
return <WrappedComponent {...this.props} />;
}
};
};
Steps to Create Application (Install Required Modules)
Step 1: Initialize a new React project: If you haven’t already, create a new React app by running:
npx create-react-app my-app
Step 2: Navigate into your project:
cd my-app
Step 3: Install any additional dependencies you might need, depending on your project requirements. For general purposes, the React app setup comes with everything you need to get started.
the updated dependencies in package.json file will look like:
"dependencies": {
"@reduxjs/toolkit": "^2.2.1",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-redux": "^9.1.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
Approach 1: Function that Returns a Function
This approach is useful for adding simple enhancements or props to a component. It’s the most straightforward way to implement an HOC.
Example: Below is the code example using `withExtraProps`:
JavaScript
import React from 'react';
// withExtraProps HOC adds additional props to the wrapped component
const withExtraPrimport React from "react";
// withExtraProps HOC adds additional props to the wrapped component
const withExtraProps = (WrappedComponent) => (props) => {
const extraProps = { extraProp: "This is an extra prop!" };
return <WrappedComponent {...props} {...extraProps} />;
};
// Simple component that displays props
const DisplayPropsComponent = (props) => (
<div>
<h1>
{Object.entries(props).map(([key, value]) => (
<p key={key}>{`${key}: ${value}`}</p>
))}
</h1>
</div>
);
// Enhanced component with extra props
const EnhancedComponent = withExtraProps(DisplayPropsComponent);
// Usage in App component
function App() {
return <EnhancedComponent someProp="This is some prop" />;
}
export default App;
ops = (WrappedComponent) => (props) => {
const extraProps = {extraProp: 'This is an extra prop!'};
return <WrappedComponent {...props} {...extraProps} />;
};
// Simple component that displays props
const DisplayPropsComponent = (props) => (
<div>
<h1>
{Object.entries(props).map(([key, value]) => (
<p key={key}>{`${key}: ${value}`}</p>
))}
</h1>
</div>
);
// Enhanced component with extra props
const EnhancedComponent = withExtraProps(DisplayPropsComponent);
// Usage in App component
function App() {
return <EnhancedComponent someProp="This is some prop" />;
}
export default App;
Output:
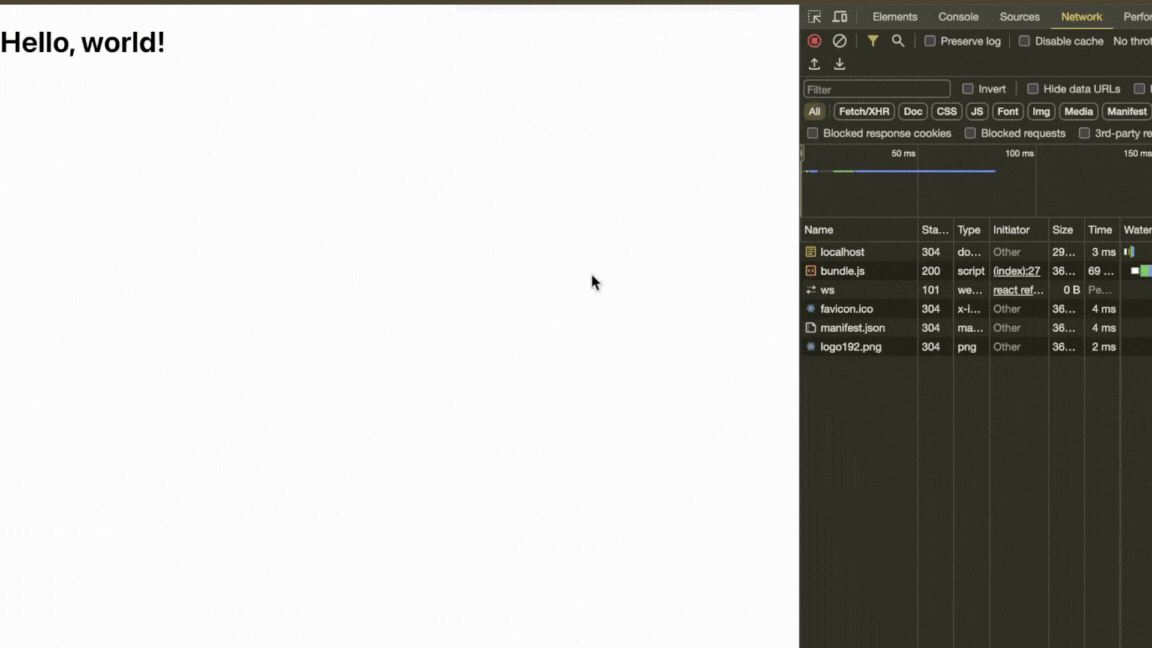
Approach 1 Output
Approach 2: Using ES6 Classes
When you need the HOC to have its own state or lifecycle methods, using ES6 classes is the preferred approach.
Example: Below is the code example `withLoadingIndicator`
JavaScript
import React from "react";
// withLoadingIndicator HOC shows a loading
// indicator based on its own state
const withLoadingIndicator = (WrappedComponent) => {
return class extends React.Component {
constructor(props) {
super(props);
this.state = { isLoading: true };
}
componentDidMount() {
// Mock loading time
setTimeout(() => this.setState({ isLoading: false }),1000);
}
render() {
const { isLoading } = this.state;
return isLoading ? (
<div>Loading...</div>
) : (
<WrappedComponent {...this.props} />
);
}
};
};
// Component to be enhanced
const HelloWorld = () => <div>Hello, world!</div>;
// Enhanced component with loading indicator
const HelloWorldWithLoading = withLoadingIndicator(HelloWorld);
// Usage in App component
function App() {
return <HelloWorldWithLoading />;
}
export default App;
Output:
.gif)
Approach 2 Output
Share your thoughts in the comments
Please Login to comment...