How to Update value with put in Express/Node JS ?
Last Updated :
28 Nov, 2023
Express JS provides various HTTP methods to interact with data. Among these methods, the PUT method is commonly used to update existing resources.
Prerequisites
In this article, we are going to setup the request endpoint on the server side using Express JS and Node JS. This endpoint will be responsible for updating the existing data in your database.
Steps to create project and install required modules
Step 1: Make a root directory using following command.
mkdir root
Step 2: Initialize NPM via following command.
npm init -y
Step 3: Install Express in your project via given command of NPM.
npm i express
Dependencies:
"dependencies": {
"express": "^4.18.2",
}
Project Structure
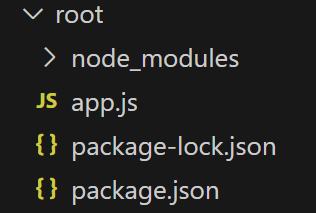
Approach
In this approach, we setup two endpoints for server-side code: one is get() and another is put(). get() will be used to display hard-coded data present in the backend, “hello world,” and put() will be used to update the database, but here we are not connecting databases; we received data in the backend and displayed that data in the console. When the user wants to save that data, they can easily save it using the dot operator.
Example: Implementation of above approach.
Javascript
const express = require( 'express' );
app = express();
app.use(express.json())
let data = "Hello Word!" ;
app.get( "/" , (req, res) => {
console.log(data);
res.send(data);
})
app.put( "/" , (req, res) => {
console.log(req.body);
res.send( "Data Recieved Successfully!" );
})
app.listen(3000, () => { console.log( "Server Connected!" ) });
|
Output:
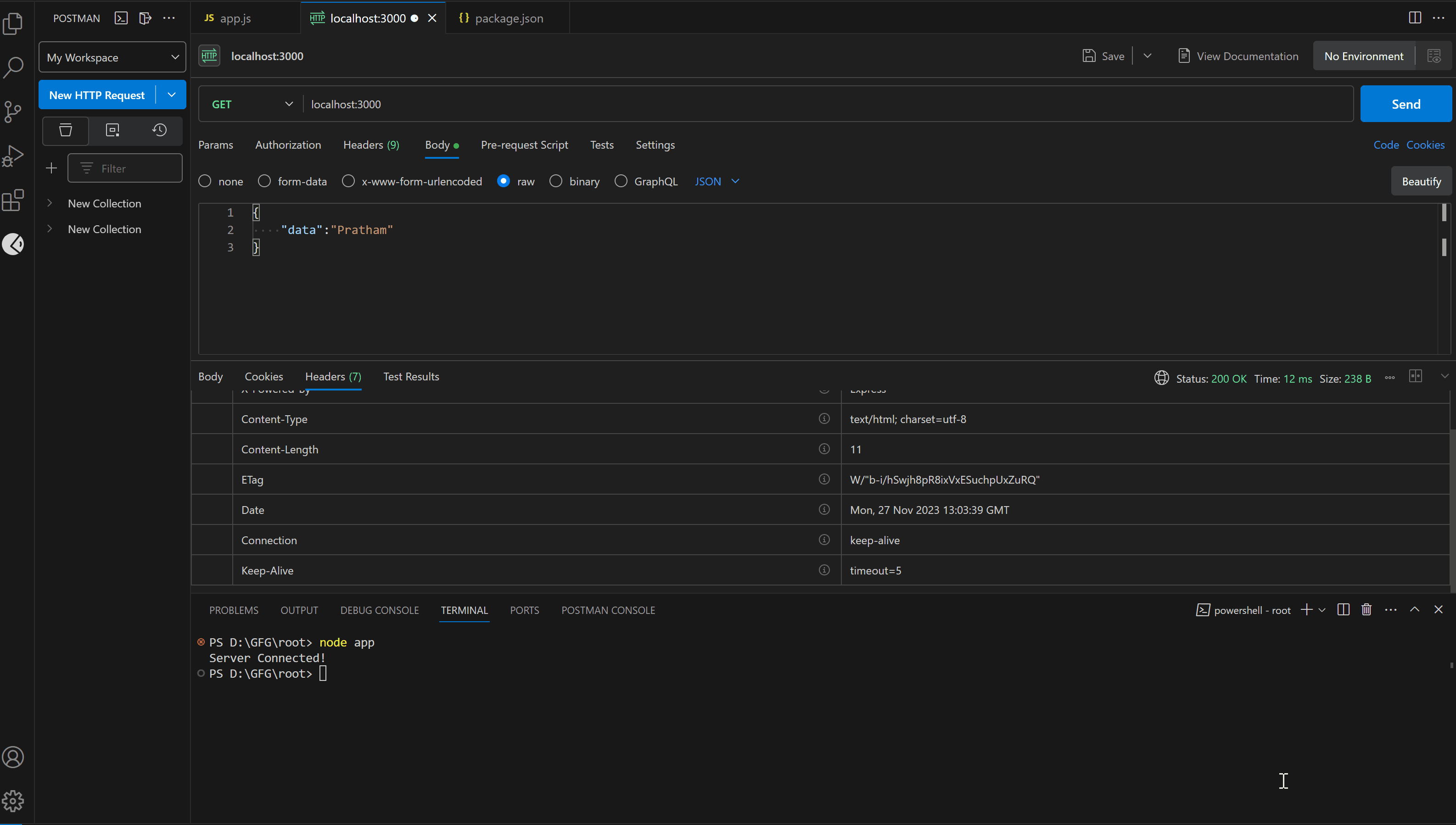
Conclusion
The HTTP PUT method in Express.js allows you to update the existing resources within the application. Using this, you can modify specific data based on unique identifiers. This is very important part of building RESTful APIs and enables applications to perform CRUD (Create, Read, Update, Delete) operations.
Share your thoughts in the comments
Please Login to comment...