How to Update JSON Object Value Dynamically in jQuery?
Last Updated :
17 Apr, 2024
In jQuery, we can update JSON object values dynamically by manipulating the object directly or using utility functions. We will explore two approaches to update JSON object values dynamically in jQuery.
Below are the approaches to update JSON object values dynamically in jQuery.
Using each() Method
In this approach, we use each() method in jQuery to iterate through the keys of the JSON object. When the input key matches an existing key in the object, we dynamically update its corresponding value and display the updated JSON object.
Example: The example below uses each() Method to update the JSON object value in jQuery dynamically.
HTML
<!DOCTYPE html>
<html>
<head>
<title>each() Method</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js">
</script>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
label {
display: block;
margin-bottom: 10px;
}
input[type="text"] {
width: 30%;
padding: 8px;
margin-bottom: 15px;
border-radius: 5px;
border: 1px solid #ccc;
box-sizing: border-box;
}
#output {
border: 1px solid #ccc;
width: 50%;
padding: 10px;
border-radius: 5px;
margin-bottom: 15px;
}
#updateBtn {
padding: 10px 10px;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
}
#updateBtn:hover {
background-color: #0056b3;
}
</style>
</head>
<body>
<h1>Using each() Method</h1>
<div>
<label for="keyInput">Enter Key:</label>
<input type="text" id="keyInput">
<label for="valueInput">Enter Value:</label>
<input type="text" id="valueInput">
<button id="updateBtn">Update Value</button>
</div>
<div id="output"></div>
<script>
let jsonObject = {
"JavaScript": "Programming Language",
"PHP": "Server Side Language"
};
$("#output").text(JSON.stringify(jsonObject));
$("#updateBtn").click(function () {
const key = $("#keyInput").val();
const value = $("#valueInput").val();
$.each(jsonObject,
function (objKey, objValue) {
if (objKey === key) {
jsonObject[objKey] = value;
}
});
$("#output").text(JSON.stringify(jsonObject));
$("#keyInput").val('');
$("#valueInput").val('');
});
</script>
</body>
</html>
Output:
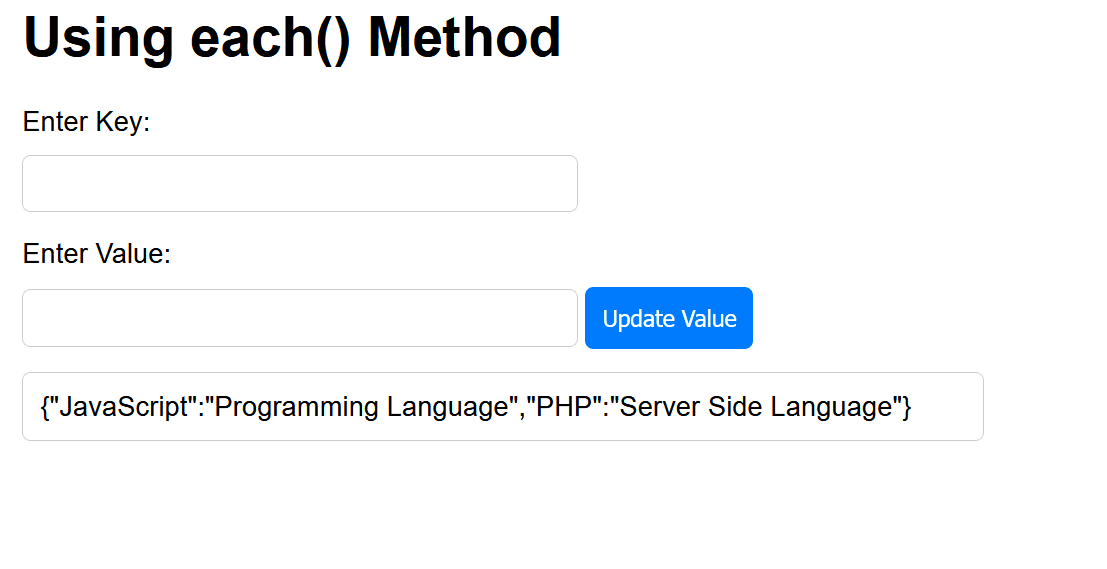
Using hasOwnProperty() Method
In this approach, we use the hasOwnProperty() method in JavaScript to check if a key exists in the JSON object before updating its value dynamically in jQuery. If the key exists, the value is updated otherwise an error alert is shown.
Example: The below example uses hasOwnProperty() to update the Json object value dynamically in jQuery.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Using hasOwnProperty() method</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
label {
display: block;
margin-bottom: 10px;
}
input[type="text"] {
width: 30%;
padding: 8px;
margin-bottom: 15px;
border-radius: 5px;
border: 1px solid #ccc;
box-sizing: border-box;
}
#output {
border: 1px solid #ccc;
width: 50%;
padding: 10px;
border-radius: 5px;
margin-bottom: 15px;
}
#updateBtn {
padding: 10px 10px;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
}
#updateBtn:hover {
background-color: #0056b3;
}
</style>
</head>
<body>
<h1>Using hasOwnProperty() method</h1>
<div>
<label for="updatesInput">
Enter Updates:
</label>
<input type="text" id="updatesInput"
placeholder="key1:value1,key2:value2,...">
<button id="updateBtn">
Update Values
</button>
</div>
<div id="output"></div>
<script>
let jsonObject = {
"JavaScript": "Programming Language",
"PHP": "Server Side Language"
};
$("#output").text(JSON.stringify(jsonObject));
$("#updateBtn").click(function () {
const updatesInput = $("#updatesInput").val();
const updates = updatesInput.split(",")
.reduce(function (obj, update) {
const parts = update.split(":");
const key = parts[0];
const value = parts[1];
if (jsonObject.hasOwnProperty(key)) {
jsonObject[key] = value;
} else {
alert("Error: Key '" +
key + "not found in the object.");
}
return obj;
}, {});
$("#output").text(JSON.stringify(jsonObject));
$("#updatesInput").val('');
});
</script>
</body>
</html>
Output:
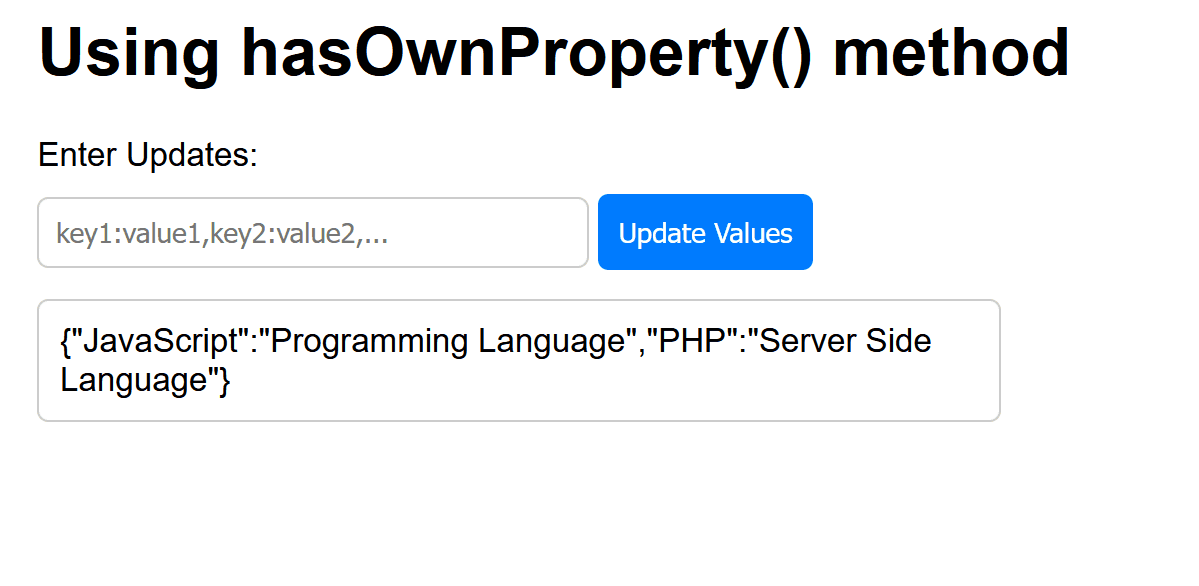
Share your thoughts in the comments
Please Login to comment...