How to Update Objects in a Document’s Array in MongoDB?
Last Updated :
26 Mar, 2024
Imagine we are managing a database with a large collection of documents, each containing arrays of nested objects. Now, here the challenge comes by updating specific objects within those arrays without altering the rest of the document. This is a common scenario in MongoDB, a powerful NoSQL database.
In this article, we’ll learn about updating objects within a document’s array, exploring multiple approaches to efficiently handle nested updating in MongoDB. Whether you’re a MongoDB novice or an experienced user, mastering these techniques will enhance your database management skills and streamline our data manipulation processes.
How to Update Objects in a Documents Array (Nested Updating) in MongoDB?
When working with MongoDB, updating objects within an array requires specific handling to ensure that only the intended objects are modified while leaving the rest of the document unchanged. MongoDB provides several methods to achieve this. Below is the method which helps us to Update Objects in a Document’s Array (Nested Updating) in MongoDB
- Using UpdateOne Method
- Using UpdateMany Method
1. UpdateOne Method
The updateOne() method is used to update a single document that matches the specified filter criteria in a collection. If multiple documents match the filter, only the first document encountered is updated.
Syntax:
db.collection.updateOne(
<filter>,
<update>,
{
arrayFilters: [ { <filterCondition> } ],
multi: true
}
)
Explanation:
- <filter>: This is the criteria to select the documents to update.
- <update>: This is the update operation to perform, including the modifications to the array elements.
- arrayFilters: This option specifies the conditions to identify the array elements to update.
- multi: true: This option ensures that all matching array elements are updated (optional).
Example: Here, We Are Updating The Value Of The HTML Course Duration From 4 Weeks To 5 Weeks Using The updateOne Method.
Suppose we have a gfg database in courses collection which stores information about various courses. Each document in the collection represents a course and contains details such as the course name, Instructor name, fees, and duration.
After inserting a record into the courses collection, Our courses look like:
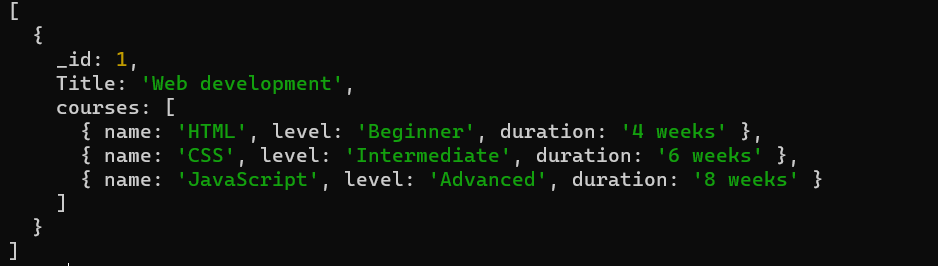
Sample Collection
Query:
Let’s Update the “duration” field of the “HTML” course in a MongoDB collection to “5 weeks” using the positional operator.
db.collection.update(
{ "_id": 1, "courses.name": "HTML" },
{ "$set": { "courses.$.duration": "5 weeks" } }
)
Output:
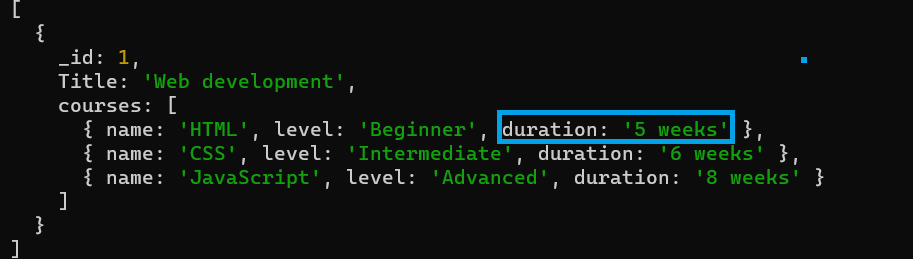
using updateOne() Method
Explanation: This query updates the “duration” field of the nested object within the “courses” array where the “name” field is “HTML” and the “_id” of the document is 1. The positional operator $
is used to update the matching element in the “courses” array.
2. UpdateMany Method
The updateMany() method is used to update all documents that match the specified filter criteria in a collection.
Syntax:
db.collection.updateMany(
<filter>,
<update>,
{
upsert: <boolean>,
writeConcern: <document>,
collation: <document>,
hint: <document|string> // Available starting in MongoDB 4.2
arrayFilters: [ ... ]
}
)
Explanation:
- db.collection.updateMany(): This is the method call for the updateMany operation on a specific collection.
- <filter>: This is a document that specifies the selection criteria for the update operation. It identifies the documents to be updated.
- <update>: This is a document that describes the modifications to be made to the selected documents.
- upsert (optional): If set to `true`, the operation creates a new document when no document matches the query criteria. The default value is false.
- writeConcern (optional): A document expressing the write concern.
- collation (optional): Specifies the collation to use for the operation.
Example: Here We Are Adding The Value Of The Fees In Every Course Using The updateMany Method.
Query:
Let’s Update the “fees” field of all courses in a MongoDB collection to “2000” using the all positional operator.
db.courses.updateMany(
{},
{ "$set": { "courses.$[].fees": "2000" } }
)
Output:
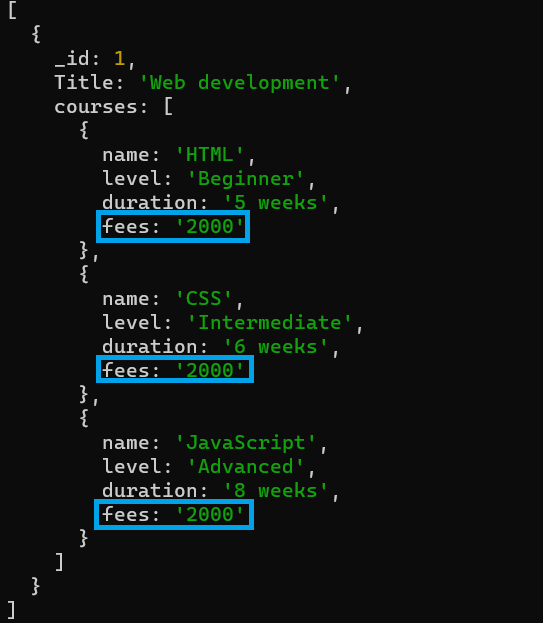
updateMany
Explanation: In the query db.courses.updateMany({}, { "$set": { "courses.$[].fees": "2000" } })
updates the “fees” field of all nested objects within the “courses” array in every document of the “courses” collection to “2000“. The positional operator $[]
is used to update all elements of the “courses” array in each document.
Conclusion
Overall, updating objects within a document’s array in MongoDB requires careful consideration to ensure that only the intended objects are modified. The updateOne method is used to update a single document that matches the specified filter criteria, while the updateMany method updates all documents that match the criteria. These methods, along with the arrayFilters option, provide powerful tools for efficiently updating nested objects in MongoDB arrays.
Share your thoughts in the comments
Please Login to comment...