How to Find Document with Array that Contains a Specific Value in MongoDB
Last Updated :
26 Mar, 2024
MongoDB is a popular choice for developers working with large volumes of data, due to its flexibility and powerful querying capabilities. One common task developers face is finding documents in a collection where an array field contains a specific value.
In this beginner-friendly article, we’ll explore simple yet effective methods to achieve this using MongoDB.
How to Find a Document with Array that Contains Specific Value in MongoDB?
In MongoDB, the find() method selects the documents in a collection and returns the cursor to the selected documents. A cursor means pointer and how it is pointing to a document, when we use the find() method it returns a pointer on the selected documents and returns one by one.
These find() methods can have the ability to access single or multiple documents in the collection.
The syntax is as follows:
db.Collection_name.find(selection, projection,options)
Explanation:
- selection: It is used to select required documents. To select all documents in the collection, we can remove the parameter or use collection_name.find().
- projection: It is used to specify which fields are to be returned in the documents based on our requirements. To return all fields in the matching documents, remove this parameter.
- options: It specifies some additional options for the parameter and modifies the behavior in selection.
Examples: Find Document with Array that Contains a Specific Value in MongoDB
Example 1
Let’s Create a MongoDB collection named Specificvalue
and insert sample documents into the collection. Display all documents from the collection and find a document where the FavouriteSubject
field contains the value “MongoDB“
// Inserting sample documents into the collection
db.Specificvalue.insertMany( [
{ "StudentId":1,
"StudentName":"Larry",
"FavouriteSubject":["C","C++","Java"]
} ,
{ "StudentId":2,
"StudentName":"Larry",
"FavouriteSubject":["MongoDB","MySQL","SQL Server"]
}
] );
// Display all documents from the collection
db.Specificvalue.find();
// Finding specific value
db.Specificvalue.find({FavouriteSubject: "MongoDB"});
Output:
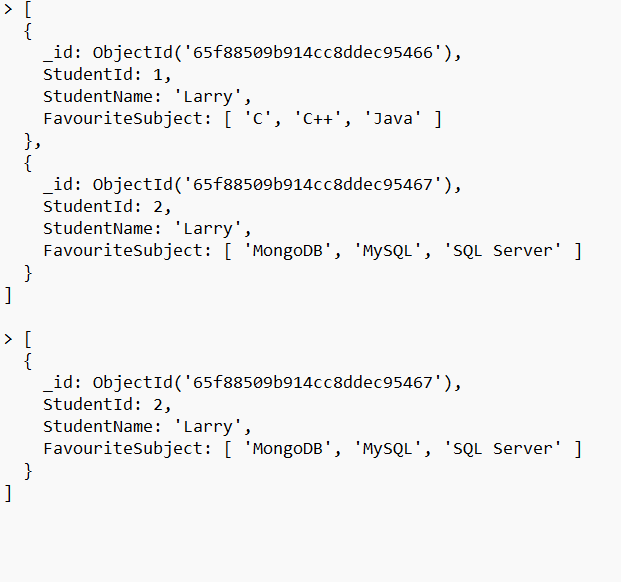
Output
Explanation: In the above output, we finded a document based on specific value is equal FavouriteSubject: “MongoDB” by using the find() function.
Example 2
Let’s Create a MongoDB collection named games
, insert the provided sample documents. Then, display all documents from the collection and find a document where the team
field contains the value “csk“.
// Inserting sample documents into the collection
db.games.insertMany([
{
player: 1,
name: "Rahul",
game: "kabaddi",
team:"Telugu titans",
age: 26,
league: "PKL",
guests: ["hero", "heroine"]
},
{
player: 2,
name: "dhoni",
game: "Cricket",
team: "csk",
age: 43,
league: "IPL",
guests: ["cm", "pm"]
}
])
// displaying all the documents
db.games.find()
//displaying specific value
db.games.find({team:"csk"})
Output:
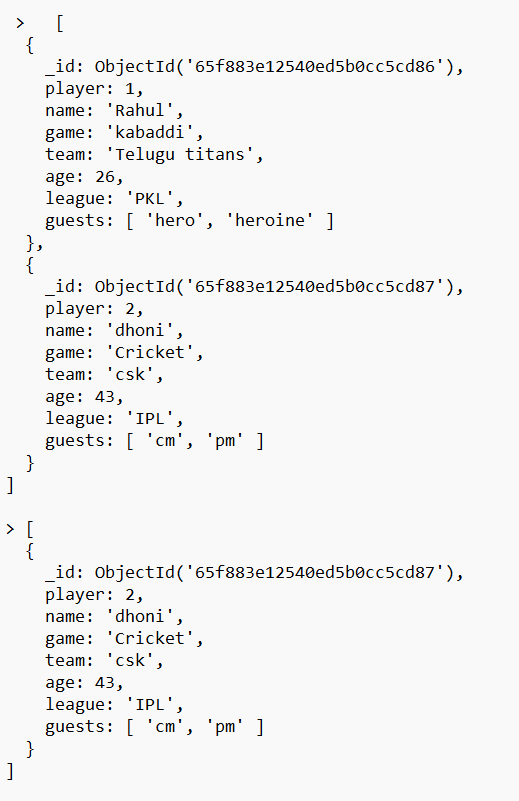
Output
Explanation: In the below output, we finded a document based on specific value which is equal to team:”csk” by using the find() function.
Example 3
Let’s Insert the provided student details into a MongoDB collection named student_details
. Display all documents from the collection and find documents where the age
field is equal to 20.
// Inserting sample documents into the collection
db.student_details.insertMany([
{
student: 1,
Name: "Bhaskar",
age: 20,
sex: "Male",
id_no: 102,
college_name: "Sri sai"
},
{
student: 2,
Name: "Bhanu priya",
age: 19,
sex: "Female",
id_no: 103,
college_name: "vishnu"
},
{
student: 3,
Name: "Gopi",
age: 20,
sex: "Male",
id_no: 104,
college_name: "Raghu"
}
]);
// Displaying all the documents
db.student_details.find();
// Displaying specific value
db.student_details.find({ age: 20 });
Output:
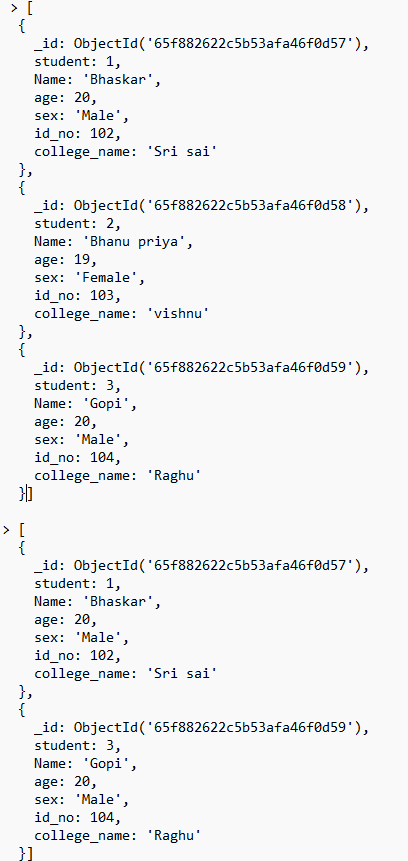
Output
Explanation: In the above output, we finded a document based on specific value is equal to “age:20” by using the find() function.
Conclusion
Overall, Finding documents in a collection where an array field contains a specific value is a common requirement.By using the find()
method with appropriate parameters, developers can easily select the required documents from a collection.
The selection
parameter allows for the selection of specific documents, while the projection
parameter specifies which fields to return in the result.In this article, we explored several examples to demonstrate how to find documents with arrays containing specific values in MongoDB.
Share your thoughts in the comments
Please Login to comment...