In the world of MongoDB and Node.js development, Mongoose serves as a powerful tool for interacting with MongoDB databases. One of Mongoose’s key methods, findOneAndUpdate, allows developers to easily find a single document in a MongoDB collection, update it, and optionally return either the original or the modified document.
In this article, We will learn about how to Update documents which not returned by Mongoose’s findOneAndUpdate method, through the various methods along with the examples and so on.
How to Use findOneAndUpdate()
in Mongoose?
The findOneAndUpdate is a method provided by Mongoose, a MongoDB object modeling tool for Node.js. This method is specifically designed to atomically find a single document in a MongoDB collection based on a query (the query is not required, if we do not pass any query then it will find the first item), update it, and optionally return either the original or the modified document.
This operation is commonly used in scenarios where we want to update a specific document and retrieve the results in one database call, ensuring consistency. The function takes a filter (query) and updates objects, and options as parameters.
Syntax:
Model.findOneAndUpdate(filter, update, options, callback)
Here, “filter” is the query condition to find the document to update, update specifies the modifications to apply, options provide additional configurations, and callback is an optional parameter for handling the result asynchronously. Below is the method which helps us to Update a document not returned by Mongoose’s findOneAndUpdate easily are as follows:
- Using new: true
- Using upsert: true
1. Using new: true
The new: true
option in Mongoose’s findOneAndUpdate
method ensures that the method returns the modified document instead of the original document. This is useful when we want to retrieve the document after it has been updated.
By default, findOneAndUpdate returns the original document before the update operation. However, by using { new: true }, it will instead return the updated document.
Syntax:
Model.findOneAndUpdate(query, update, { new: true });
Example: The below code uses new: true to update the document and fetch the updated document at the time of update.
// importing all requirements
const User = require('../models/User');
// to fetch update the user data
const updateUser = async (req, res) => {
//Find any user and return
const user = await User.findOne({ email: 'gfg@gmail.com' });
// now, we want to update the name of the user
const name = "Geeks For Geeks";
// to get the updated user's data, we have to set the {new: true}
const updatedUser = await User.findOneAndUpdate(
{ email: 'gfg@gmail.com' },
{ $set: { fullName: name }},
{ new: true }
);
// here we will return the user
return res.status(200).json({ oldUser: user, updatedUser });
}
Output:
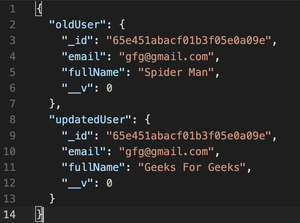
User , With {new: true} and without
Explanation: This code snippet defines an asynchronous function updateUser responsible for updating a user’s data in a MongoDB database using Mongoose. Initially, it fetches the user data based on the email address ‘gfg@gmail.com’ using the findOne method. Then, it sets a new name for the user as ‘Geeks For Geeks‘.
Subsequently, it utilizes findOneAndUpdate to update the user’s full name to the new value. By setting the option { new: true }, it ensures that the updated user data is returned. Finally, the function responds with a JSON object containing both the original user data (oldUser) fetched before the update and the updated user data (updatedUser).
2. Using upsert: true
The upsert: true
option in Mongoose’s findOneAndUpdate
method instructs MongoDB to create a new document if the query criteria do not match any existing documents. This is useful when we want to update a document if it exists but also create it if it doesn’t exist.
Syntax:
Model.findOneAndUpdate(query, update, { upsert: true });
Example: The below code uses `upsert: true` to update the document and fetch the updated document at the time of update.
// importing all requirements
const User = require('../models/User');
// to fetch update the user data
const updateUser = async (req, res) => {
//Find any user and return
const user = await User.findOne({ email: 'gfg@dev.com' });
// now, we want to update the name of the user
const name = "GeeksForGeeks";
// to get the updated user's data, we have to set the {new: true}
let updatedUser = await User.findOneAndUpdate(
{ email: 'gfg@dev.com' },
{ $set: { fullName: name }},
{ new: true, upsert: true }
);
// here we will return the user
return res.status(200).json({ updatedUser, oldUser: user });
}
Output:
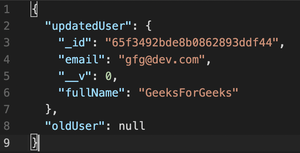
User when {upsert: true}
Explanation: In this code snippet, the updateUser function attempts to update a user’s data in a MongoDB database. Initially, it searches for a user with the email ‘gfg@dev.com’ with the help of findOne method.
If no user is found with this email, MongoDB will create a new document based on the query criteria due to the { upsert: true } option specified in the subsequent findOneAndUpdate call. The findOneAndUpdate method updates the user’s full name to the new value and returns the updated user’s data.
Conclusion
Overall, the findOneAndUpdate method in Mongoose provides developers with a powerful tool for updating documents in MongoDB collections. By understanding and utilizing the new: true
and upsert: true
options, developers can efficiently update documents not returned by findOneAndUpdate, streamlining database operations in their Node.js applications. Whether it’s updating existing documents or creating new ones on the fly, findOneAndUpdate proves to be an invaluable method in the toolkit of any MongoDB developer.
Share your thoughts in the comments
Please Login to comment...