How to Sort List by Date Filter in AngularJS ?
Last Updated :
10 Oct, 2023
AngularJS is a feature-based JavaScript framework that uses various tools to create dynamic single-page web applications. While developing the application we have the requirement to play with the data in AngularJS and sort this list of data by a date properly in descending order. This can be done using the AngularJS filters, as these filters enable us to format, transform, and manipulate the data before it is displayed in our application. In this article, we will see two different approaches through which we can perform descending order-by-date filtering in AngularJS.
Steps for Configuring AngularJS Application
The below steps will be followed to configure the AngularJS Application:
- Create a new folder for the project. We are using the VSCode IDE to execute the command in the integrated terminal of VSCode.
mkdir desc-date
cd desc-date
- Create the index.html file in the newly created folder, we will have all our logic and styling code in this file. We can also create separate files for HTML, CSS, and JS:
We will explore the Descending order-by-date filter in AngularJS with the help of suitable approaches & will understand its implementation through the illustration.
Using orderBy filter with ng-repeat Directive
In this approach, we are implementing the descending and ascending order of the list of students and their join dates. We have used the ‘orderBy‘ filter with the ng-repeat directive that will enable us to dynamically sort the student information by allowing us to select the desired sorting order (Ascending or Descending) using a simple drop-down menu. Data is initially sorted in ascending order by join date, but we can sort it in descending order by selecting the descending order option from the drop-down list.
Example: Below is an example that demonstrates performing the Descending order by date filter in AngularJS using the orderBy filter with ng-repeat Directive.
HTML
<!doctype html>
< html ng-app = "myApp" >
< head >
< title >
Descending Order by Date Filter
</ title >
< script src =
</ script >
< style >
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
margin: 0;
padding: 0;
}
h1 {
color: green;
margin-top: 20px;
}
h3 {
color: #333;
}
.container {
max-width: 800px;
margin: 0 auto;
padding: 20px;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
ul {
list-style-type: none;
padding: 0;
}
li {
margin: 10px 0;
padding: 10px;
border: 1px solid #ddd;
background-color: #f9f9f9;
border-radius: 5px;
}
input[type="text"] {
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</ style >
</ head >
< body >
< div class = "container"
ng-controller = "StudentController" >
< center >
< h1 >GeeksforGeeks</ h1 >
</ center >
< center >
< h3 >
Apporach 1: Using orderBy
filter with ng-repeat
</ h3 >
</ center >
< label for = "dateFilter" >
Select a Date Filter:
</ label >
< select id = "dateFilter"
ng-model = "dateOrder" >
< option value = "asc" >
Ascending
</ option >
< option value = "desc" >
Descending
</ option >
</ select >
< h3 >Students:</ h3 >
< ul >
< li ng-repeat="student in filteredStudents =
(students | orderBy:
(dateOrder === 'desc' ? '-joinDate' : 'joinDate'))">
< strong >{{ student.name }}</ strong > -
{{ student.joinDate | date: "dd/MM/yyyy" }}
(Age: {{ student.age }})
</ li >
</ ul >
</ div >
< script >
var app = angular.module("myApp", []);
app.controller("StudentController", function ($scope) {
$scope.students = [
{ name: "Ramesh",
joinDate: new Date("05/17/2015"),
age: 21 },
{ name: "Sandeep",
joinDate: new Date("03/25/2016"),
age: 22 },
{ name: "Suresh",
joinDate: new Date("09/11/2015"),
age: 20 },
{ name: "Deepika",
joinDate: new Date("01/07/2016"),
age: 21 },
{ name: "Esha",
joinDate: new Date("03/09/2014"),
age: 23 },
];
$scope.dateOrder = "asc";
});
</ script >
</ body >
</ html >
|
Output:
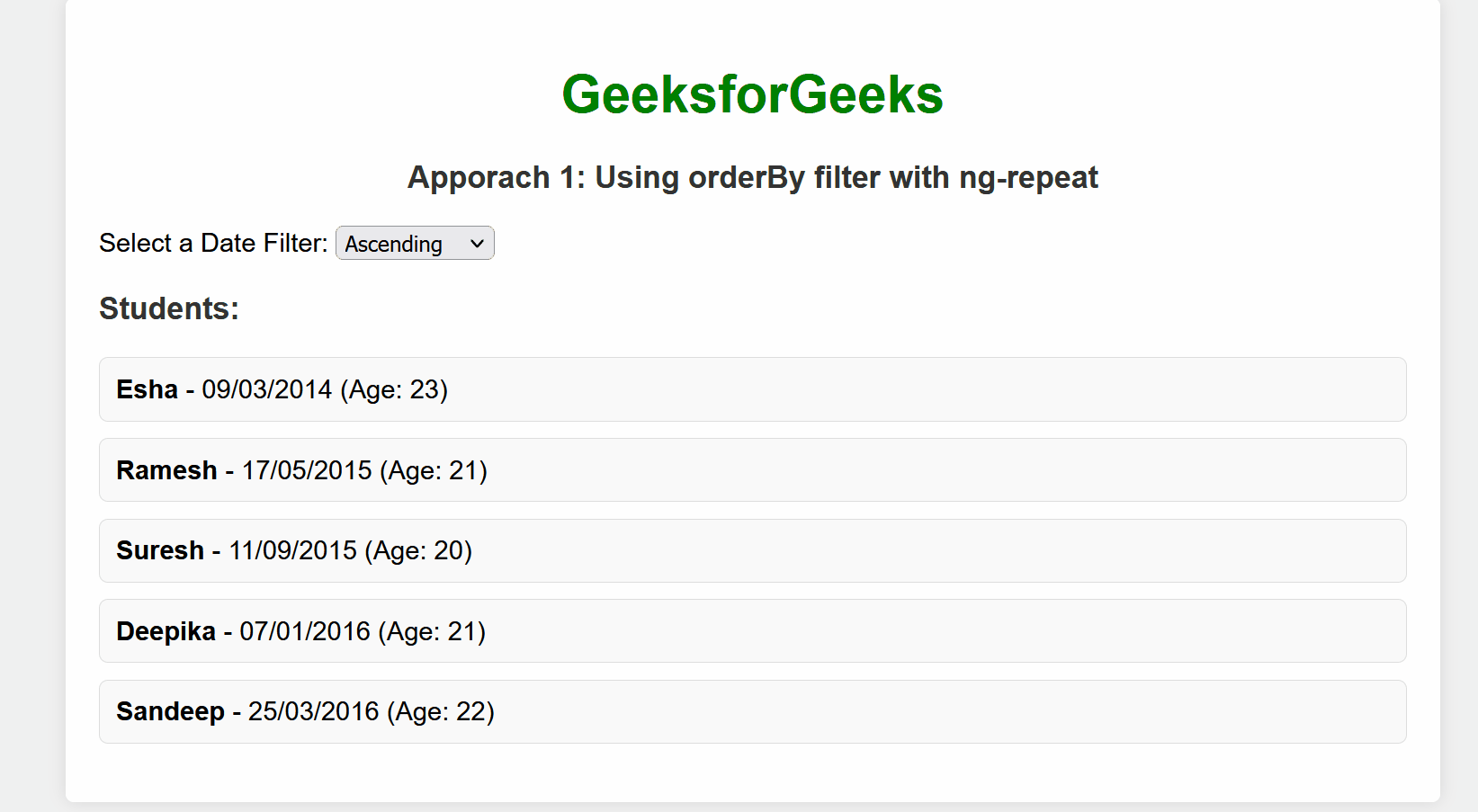
Using reverse flag with ng-click Directive
In this approach, we are sorting the sample list of data that contains the payment dates. We have used the button that sorts the data in ascending and descending order by use of the ng-click directive which updates the reverse flag. the reverse flag in AngularJS mainly reverses the order of the payment list by using the ‘orderBy‘ filter and allows us to switch from the ascending and descending sorting type. When the user clicks on the descending option, the reverse flag is been updated and all the dates are sorted in descending order.
Example: Below is an example that showcases performing the Descending order by date filter in AngularJS using the reverse flag with the ng-click directive.
HTML
<!doctype html>
< html ng-app = "myApp" >
< head >
< title >
Descending Order by Payment
Date Filter - Approach 2
</ title >
< script src =
</ script >
< style >
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
margin: 0;
padding: 0;
}
.header {
background-color: transparent;
text-align: center;
padding: 20px;
}
.container {
max-width: 800px;
margin: 0 auto;
padding: 20px;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h1 {
color: green;
}
h3 {
margin-top: 20px;
}
ul {
list-style-type: none;
padding: 0;
}
li {
margin: 10px 0;
padding: 10px;
border: 1px solid #ddd;
background-color: #f9f9f9;
border-radius: 5px;
}
input[type="text"],
select {
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
.toggle-button {
background-color: green;
color: white;
border: none;
padding: 10px 20px;
border-radius: 5px;
cursor: pointer;
}
</ style >
</ head >
< body >
< div class = "header" >
< h1 >GeeksforGeeks</ h1 >
</ div >
< div class = "container"
ng-controller = "PaymentController" >
< h3 >
Approach 2: Sort Payments by Payment Date
</ h3 >
< label for = "dateFilter" >
Select Payment Date Order:
</ label >
< button class = "toggle-button"
ng-click = "toggleOrder()" >
{{ reverse ? "Sort Ascending" : "Sort Descending" }}
</ button >
< h3 >Payments:</ h3 >
< ul >
< li ng-repeat =
"payment in payments | orderBy:'date':reverse" >
< strong >{{ payment.name }}</ strong >
- {{ payment.date | date: "dd/MM/yyyy" }}
</ li >
</ ul >
</ div >
< script >
var app = angular.module("myApp", []);
app.controller("PaymentController", function ($scope) {
$scope.payments = [
{ name: "Payment A",
date: new Date("2019-09-25") },
{ name: "Payment B",
date: new Date("2020-03-15") },
{ name: "Payment C",
date: new Date("2021-06-12") },
{ name: "Payment D",
date: new Date("2022-12-07") },
];
$scope.reverse = false;
$scope.toggleOrder = function () {
$scope.reverse = !$scope.reverse;
};
});
</ script >
</ body >
</ html >
|
Output:
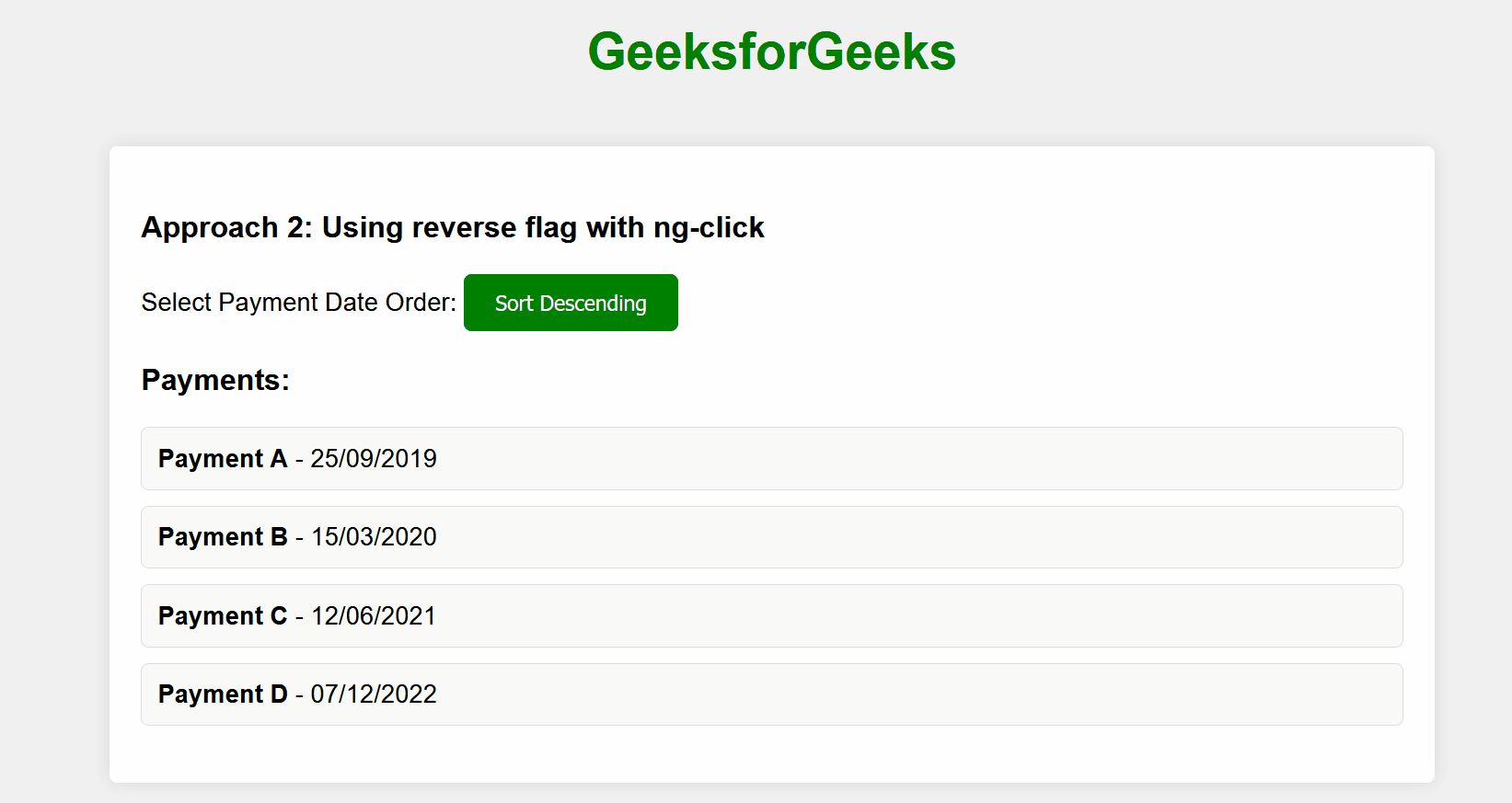
Share your thoughts in the comments
Please Login to comment...