How to Set Chart.js Y-axis Title ?
Last Updated :
02 Jan, 2024
Chart.js is the JavaScript library to represent the data in visual forms in terms of various charts like Bar, Pies, etc. We can set and customize the axes of the chart by setting the Y-axis title. This provides better information about the vertical axis of the Chart. In this article, we will see how we can set the Chart.js Y-axis title by using two different approaches.
Below are the possible approaches:
CDN link
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.9.4/Chart.min.js"></script>
Approach 1: Using Chart.js Global Options
- In this approach, we are using the options object which is from Chart.js Global.
- There is a scale configuration in which we can customize the y-axis by setting its title.
- For the below example, we have set the title as “More Popular” which is displayed on the y-axis of the Bar chart.
Syntax:
const options = {
scales: {
y: {
title: {
display: true,
text: 'Title'
}
}
}
};
Example: Below is the implementation of the above-discussed approach.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Example 1</ title >
< script src =
</ script >
</ head >
< body >
< div >
< h1 style = "color:green;" >GeeksforGeeks</ h1 >
< h3 >Approach 1: Using Chart.js Global Options </ h3 >
< div style = "width:90%;" >
< canvas id = "chart1" ></ canvas >
</ div >
</ div >
< script >
const data = {
labels: ['JavaScript', 'Python', 'Java', 'C#', 'C++'],
datasets: [{
label: 'Popularity',
data: [70, 60, 50, 40, 30],
backgroundColor: 'rgba(255,0,0)',
borderColor: 'rgba(11,156,49)',
borderWidth: 1
}]
};
const options = {
scales: {
y: {
title: {
display: true,
text: 'More Popular'
}
}
}
};
const ctx = document.getElementById('chart1').getContext('2d');
const chart1 = new Chart(ctx, {
type: 'bar',
data: data,
options: options
});
</ script >
</ body >
</ html >
|
Output:
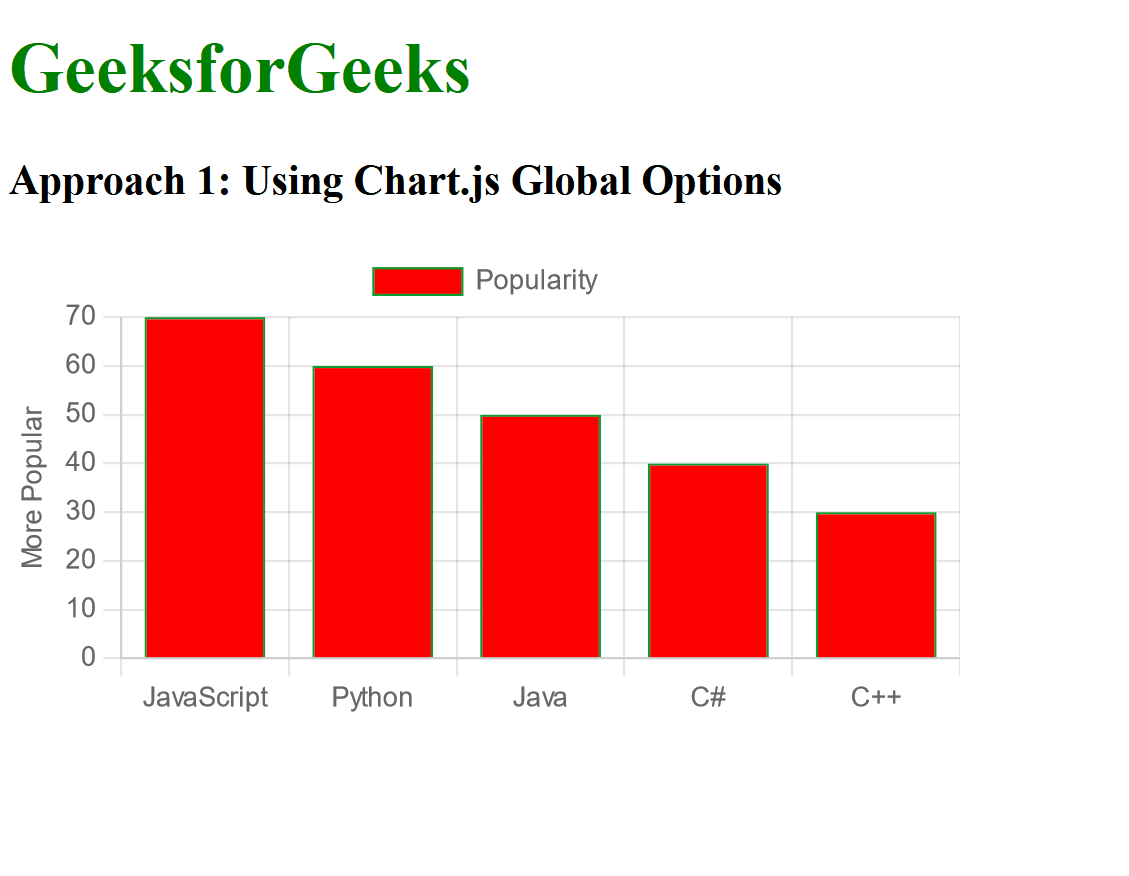
Approach 2: Using yAxes Option
- In this approach, we are using the yAxes option in Chart.js which configures the scale property to include the yAxes array.
- By using this array, the scaleLabel property is used to set the Y-axis title.
- We have given the title “Number of Visitors“.
- This option is mainly used with Chart.js Version 2.
Syntax:
scales: {
yAxes: [{
scaleLabel: {
display: true,
labelString: 'Y-axis Title'
}
}]
}
Example: Below is the implementation of the above-discussed approach.
HTML
<!DOCTYPE html>
< html >
< head >
< script src =
</ script >
< title >Example 2</ title >
</ head >
< body >
< h1 style = "color:green;" >GeeksforGeeks</ h1 >
< h3 >Approach 2: Using yAxes Option </ h3 >
< canvas id = "myChart" width = "400" height = "200" ></ canvas >
< script >
let ctx = document.getElementById('myChart').getContext('2d');
let myChart = new Chart(ctx, {
type: 'bar',
data: {
labels: ['January', 'February', 'March', 'April', 'May'],
datasets: [{
label: 'GeeksforGeeks Data',
data: [12, 19, 3, 5, 2],
backgroundColor: 'rgba(75, 192, 192, 0.2)',
borderColor: 'rgba(75, 192, 192, 1)',
borderWidth: 1
}]
},
options: {
scales: {
yAxes: [{
scaleLabel: {
display: true,
labelString: 'Number of Views'
}
}]
},
legend: {
display: true,
position: 'top'
}
}
});
</ script >
</ body >
</ html >
|
Output:
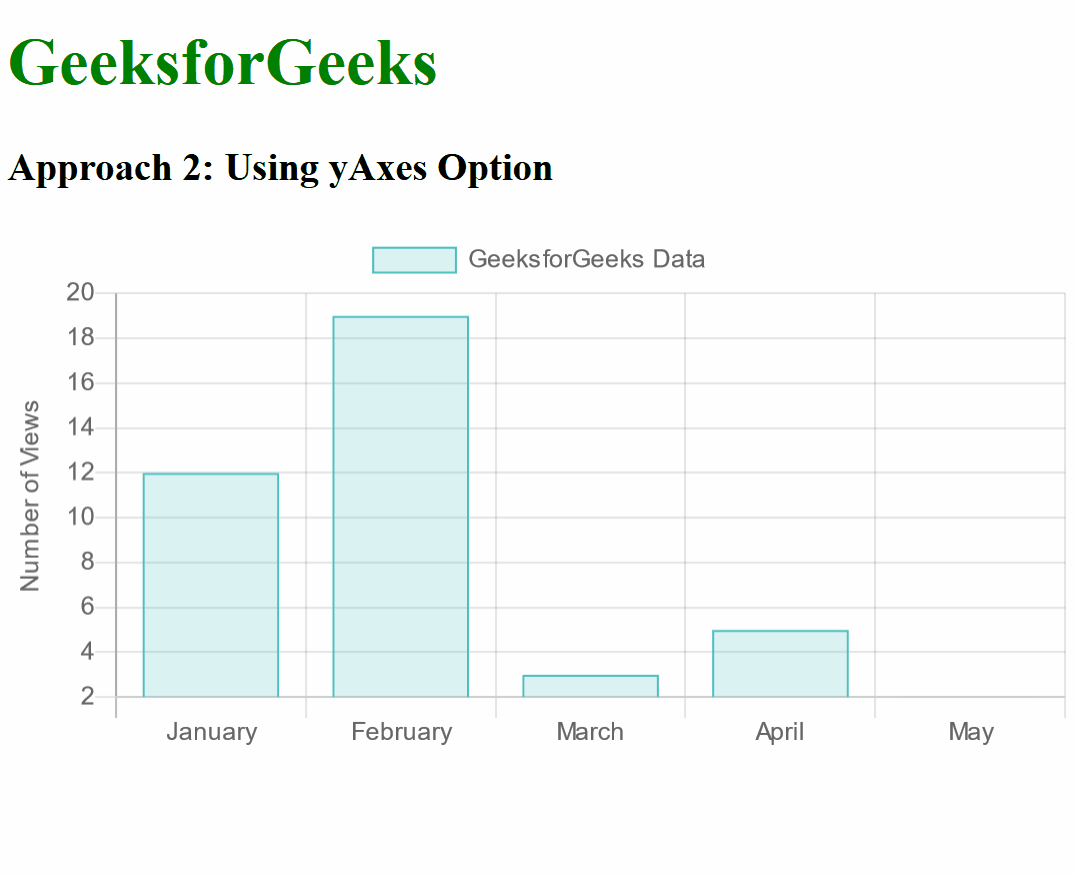
Share your thoughts in the comments
Please Login to comment...