How to Set Height and Width of a Chart in Chart.js ?
Last Updated :
09 Jan, 2024
Chart.js is a popular JavaScript library that allows developers to create interactive and visually appealing charts for web applications. One of the key aspects of chart customization is controlling its dimensions. In this article, we’ll explore how to set the height and width of a Chart.js chart.
Chart.js CDN link
<script src="https://cdnjs.cloudflare.com/ajax/libs/Chart.js/3.7.0/chart.min.js"></script>
Approach 1: By using the style attribute
We can set the height and the width as CSS properties for the container containing the canvas of our chart inside the style attribute.
Syntax:
<div style=" height:40vh; width:80vw">
<canvas id="myChart"></canvas>
</div>
Example: The below code will explain the use of the style attribute to set the height and the width of the chart.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Chart.js - Set Height and Width</ title >
< script src =
</ script >
</ head >
< body >
< div >
< div style="height:40vh;
width:80vw">
< canvas id = "myChart" >
</ canvas >
</ div >
</ div >
< script >
var ctx = document.
getElementById('myChart').
getContext('2d');
new Chart(ctx, {
type: 'bar',
data: {
labels:
['January', 'February', 'March', 'April', 'May', 'June', 'July'],
datasets: [{
label: 'My Dataset',
data:
[65, 71, 62, 81, 34, 55, 47],
backgroundColor:
'rgba(75, 192, 192, 0.2)',
borderColor:
'rgba(75, 192, 192, 1)',
borderWidth: 1
}]
},
options: {
scales: {
y: {
beginAtZero: true
}
}
}
});
</ script >
</ body >
</ html >
|
Output:
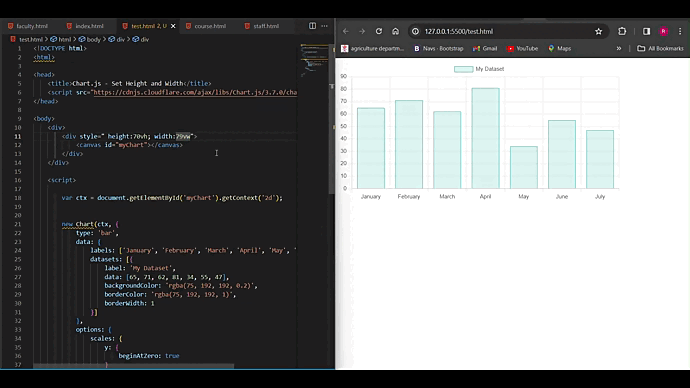
Approach 2: By setting height and width as attribuutes
We can set the height and the width by passing them as attributes directly to the canvas of our chart. We also have to set responsive to false within options for width to work correctly.
Syntax:
<canvas id="myChart" height="200" width="500"></canvas>
// In Options
options: {
responsive: false,
}
Example: The below example will show how you can set height nad width of the chart using the attributes.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Chart.js - Set Height and Width</ title >
< script src =
</ script >
</ head >
< body >
< div >
< div >
< canvas id = "myChart"
height = "300"
width = "600" >
</ canvas >
</ div >
</ div >
< script >
const ctx = document.
getElementById('myChart').
getContext('2d');
new Chart(ctx, {
type: 'bar',
data: {
labels:
['January', 'February', 'March', 'April', 'May', 'June', 'July'],
datasets: [{
label: 'My Dataset',
data:
[65, 71, 62, 81, 34, 55, 47],
backgroundColor:
'rgba(75, 192, 192, 0.2)',
borderColor:
'rgba(75, 192, 192, 1)',
borderWidth: 1
}]
},
options: {
responsive: false,
scales: {
y: {
beginAtZero: true
}
}
}
});
</ script >
</ body >
</ html >
|
Output:
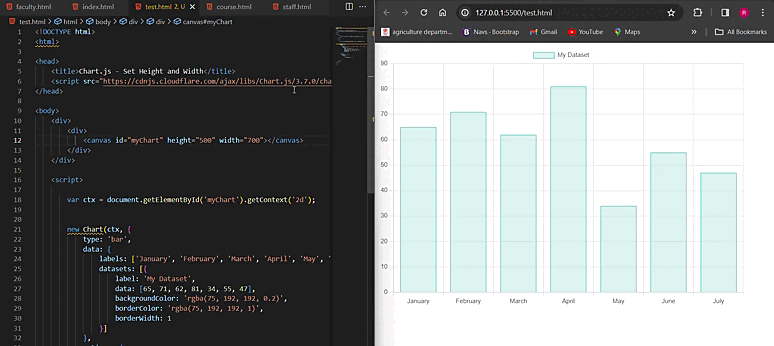
Appraoch 3: Using .resize() method
we can use .resize(width, height) method to resize our chart by invoking it using the two parameters as height and the width.
Syntax:
function resize() {
myChart.resize(700, 450);
};
Example: The below code example uses the resize() method to set height and width of the chart in chart.js.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >
Chart.js Example
</ title >
< script src =
</ script >
</ head >
< body >
< canvas id = "myChart" >
</ canvas >
< script >
var ctx = document.
getElementById('myChart');
var myChart = new Chart(ctx, {
type: 'bar',
data: {
labels:
['Red', 'Blue', 'Yellow', 'Green'],
datasets: [{
label: '# of Votes',
data: [12, 19, 3, 5]
}]
},
options: {
responsive: false,
}
});
function resize() {
myChart.resize(600, 450);
};
resize();
</ script >
</ body >
</ html >
|
Output:
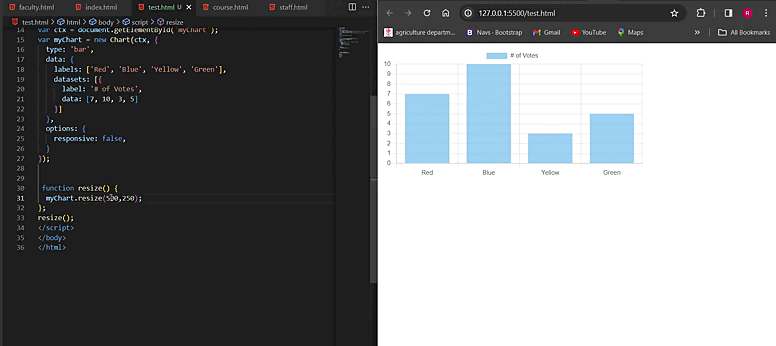
Share your thoughts in the comments
Please Login to comment...