How to set a Maximum Value less than a Number on a Decimal based Input Field ?
Last Updated :
01 Feb, 2024
Setting a maximum value of less than a specific number on a decimal-based input field is important to control and limit the range of acceptable inputs. It also provides users with clear guidance on the acceptable range of values for the input field. We can set a maximum value with different methods, including HTML max Attribute and Javascript.
Syntax:
// Using Max Attribute
<input type="number"
class="dec"
step="0.1"
id="decimalInput"
name="decimalInput"
max="5.00" required>
Using Max Attribute
Setting a maximum value less than a number can be done by adding a max attribute to the input field to set a range for the input. And a step value of 0.1 or 0.01 to make it accept Decimal values. When the form is submitted, It is accepted only when the input is given according to the specified range. If we set the max = “5.00” then the form will not be submitted with a value greater than 5.00.
Example: Illustration of Setting a maximum value less than a number on a decimal-based input field.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Set a maximum value less than a number</ title >
< style >
@import url(
* {
padding: 0;
margin: 0;
font-family: 'Open Sans', sans-serif;
}
body {
display: flex;
height: 100vh;
gap: 1rem;
justify-content: center;
align-items: center;
flex-direction: column;
}
h1, h3 {
color: green;
}
label {
font-size: 1.4rem;
}
.dec {
width: 10rem;
height: 2rem;
text-align: center;
margin: 2rem;
}
.submit {
padding: .6rem;
width: 6rem;
background-color: green;
color: #fff;
border: 2px solid transparent;
transition: all .2s ease;
}
.submit:hover {
color: green;
background-color: #fff;
border: 2px solid green;
cursor: pointer;
}
form {
text-align: center;
padding: 2rem;
box-shadow: rgba(100, 100, 111, 0.2) 0px 7px 29px 0px;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< h3 >Set a maximum value less than a
number on a decimal based input field
</ h3 >
< form >
< label for = "decimalInput" >
Enter a decimal number:
</ label >
< input type = "number"
class = "dec"
step = "0.1"
id = "decimalInput"
name = "decimalInput"
max = "5.00"
required>< br >
< input type = "submit"
value = "Submit"
class = "submit" >
</ form >
</ body >
</ html >
|
Output:
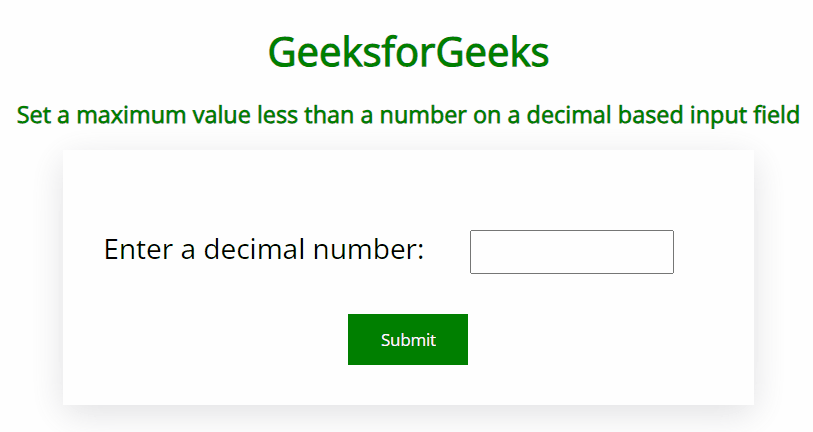
Using JavaScript
For Setting a maximum value less than a number on a decimal-based input field, we are going to restrict input values using Javascript. The JavaScript code, linked to an HTML form, checks if the entered value by the user in the input field is greater than a predefined maximum limit. If the condition holds true, it prevents the form submission and displays a warning message for enhancing user input validation.
Example: Illustration of Setting a maximum value less than a number on a decimal-based input field.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< link rel = "stylesheet" href = "style.css" >
< title >Set a maximum value less than a number</ title >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< h3 >
Maximum value less than a
number on a decimal based input field
using JavaScript
</ h3 >
< form id = "form" >
< p id = "warning" >Values should be less than 20 </ p >
< label for = "decimalInput" >Enter a decimal number:</ label >
< input type = "number"
id = "decimal"
step = "0.01" required>< br >
< input type = "submit"
value = "Submit"
class = "submit" >
</ form >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
@import url (
* {
padding : 0 ;
margin : 0 ;
}
body {
display : flex;
height : 100 vh;
gap: 1 rem;
justify- content : center ;
align-items: center ;
flex- direction : column;
font-family : 'Open Sans' , sans-serif ;
}
h 1 {
color : green ;
}
h 3 {
color : green ;
}
label {
font-size : 1.4 rem;
}
#dec imal {
width : 10 rem;
height : 2 rem;
text-align : center ;
margin : 2 rem;
}
.submit {
padding : . 6 rem;
width : 6 rem;
background-color : green ;
color : #fff ;
border : 2px solid transparent ;
transition: all . 2 s ease;
}
.submit:hover {
color : green ;
background-color : #fff ;
border : 2px solid green ;
cursor : pointer ;
}
form {
text-align : center ;
padding : 2 rem;
box-shadow: rgba( 100 , 100 , 111 , 0.2 ) 0px 7px 29px 0px ;
}
p {
display : none ;
font-size : 1.4 rem;
color : red ;
padding : 1 rem;
border : 2px solid red ;
}
|
Javascript
let form = document.getElementById( "form" );
form.addEventListener( 'submit' , submitForm);
let input = document.getElementById( 'decimal' );
let warning = document.getElementById( 'warning' );
let max = 20.00;
function submitForm(event) {
if (parseFloat(input.value) > max) {
event.preventDefault();
warning.style.display = "block" ;
}
}
|
Output:
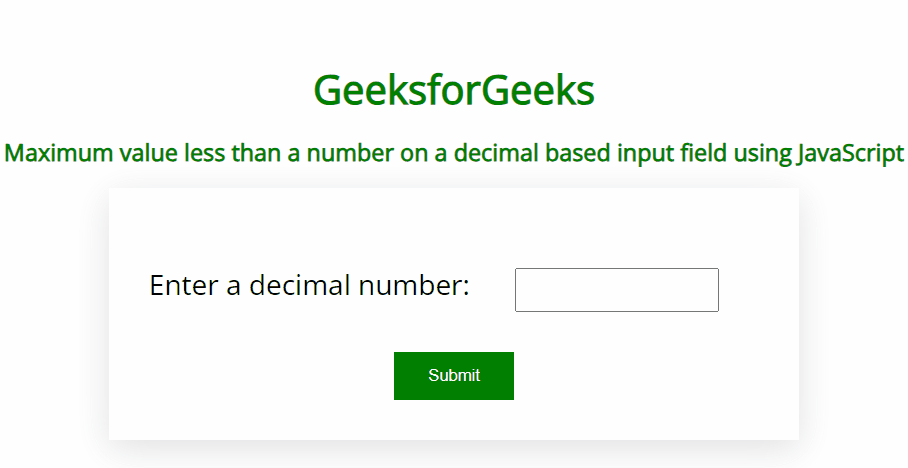
Share your thoughts in the comments
Please Login to comment...