Set the value of an input field in JavaScript
Last Updated :
24 Nov, 2023
In this article, we are going to learn how can we Set the value of an input field in JavaScript. We need to set the given input, in which the input will be provided by the input field and we need to display that input on the screen.
These are the following methods by using we can Set the value of an input field in JavaScript:
By using the innerHTML property
- In this approach, we are going to call a function when the user clicks the button.
- The click event will occur and a function will be invoked.
- The function will get the input value from the input field and add that value using the “innerHTML” property provided by JavaScript.
- At last, that value will be displayed on the screen.
Example: This example is the implementation of the above-explained approach.
html
<!DOCTYPE html>
< html >
< head >
< title >
Set the value of an input field.
</ title >
</ head >
< body style = "text-align:center;" id = "body" >
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< input type = 'text' id = 'id1' />
< br >
< br >
< button onclick = "gfg_Run()" >
click to set
</ button >
< p id = "GFG_DOWN" style="color:green;
font-size: 20px;
font-weight: bold;">
</ p >
< script >
let el_down = document.getElementById("GFG_DOWN");
let inputF = document.getElementById("id1");
function gfg_Run() {
inputF.value = "textValue";
el_down.innerHTML =
"Value = " + "'" + inputF.value + "'";
}
</ script >
</ body >
</ html >
|
Output:
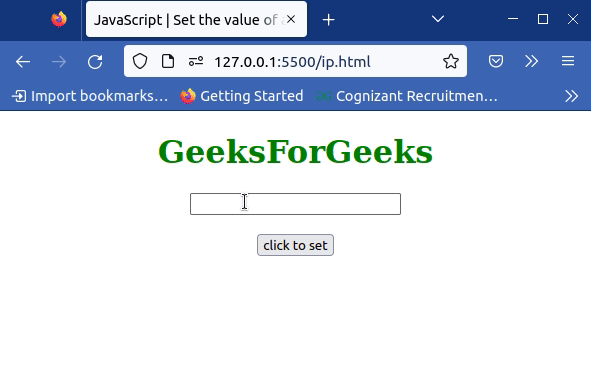
Set the value of an input field
- In this approach, we are going to call a function when the user clicks the button.
- The click event will occur and a function will be invoked.
- The function will get the input value from the input field and set that value using the setAttribute() method.
- And add that value using the “innerHTML” property provided by JavaScript.
- At last, that value will be displayed on the screen.
Example: This example is the implementation of the above-explained approach.
html
<!DOCTYPE html>
< html >
< head >
< title >
Set the value of an input field.
</ title >
</ head >
< body style = "text-align:center;" id = "body" >
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< input type = 'text' id = 'id1' />
< br >
< br >
< button onclick = "gfg_Run()" >
click to set
</ button >
< p id = "GFG_DOWN" style="color:green;
font-size: 20px;
font-weight: bold;">
</ p >
< script >
let el_down = document.getElementById("GFG_DOWN");
let inputF = document.getElementById("id1");
function gfg_Run() {
inputF.setAttribute('value', 'defaultValue');
el_down.innerHTML =
"Value = " + "'" + inputF.value + "'";
}
</ script >
</ body >
</ html >
|
Output:
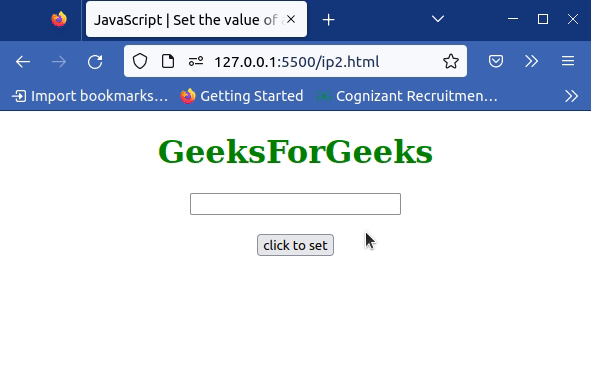
Set the value of an input field
Share your thoughts in the comments
Please Login to comment...