How to Save Data in Local Storage in JavaScript ?
Last Updated :
09 Apr, 2024
LocalStorage is a client-side web storage mechanism provided by JavaScript that allows developers to save key-value pairs locally in the user’s browser. It provides a simple way to store data persistently across page reloads, making it ideal for storing user preferences, session data, and other information that needs to be retained for users to visit the website again.
Approach:
- In this approach, we are using the localStorage object in JavaScript to store user input data locally in the browser.
- The saveData function captures the input value and saves it with the key ‘userData’ using localStorage.setItem().
- The displayData function retrieves the saved data using localStorage.getItem() and displays it on the webpage.
Syntax:
// Storing data in local storage
localStorage.setItem('name', 'anyName');
// Retrieving data from local storage
const username = localStorage.getItem('name');
console.log(username);
Example: The below example uses localStorage Object to save data in local storage in JavaScript.
HTML
<!DOCTYPE html>
<head>
<title>Example 1</title>
</head>
<body>
<div>
<h1
style="color: green;">GeeksforGeeks</h1>
<h3>Using localStorage Object</h3>
<label
for="dataInput">Enter Data:</label>
<input
type="text"
id="dataInput">
<button
onclick="saveData()">Save Data</button>
</div>
<div>
<h2>Saved Data:</h2>
<div id="savedData"></div>
</div>
<script>
function saveData() {
const data = document
.getElementById('dataInput')
.value;
localStorage
.setItem('userData', data);
displayData();
}
function displayData() {
const savedData = localStorage
.getItem('userData');
document
.getElementById('savedData')
.innerText = savedData;
}
displayData();
</script>
</body>
</html>
Output:
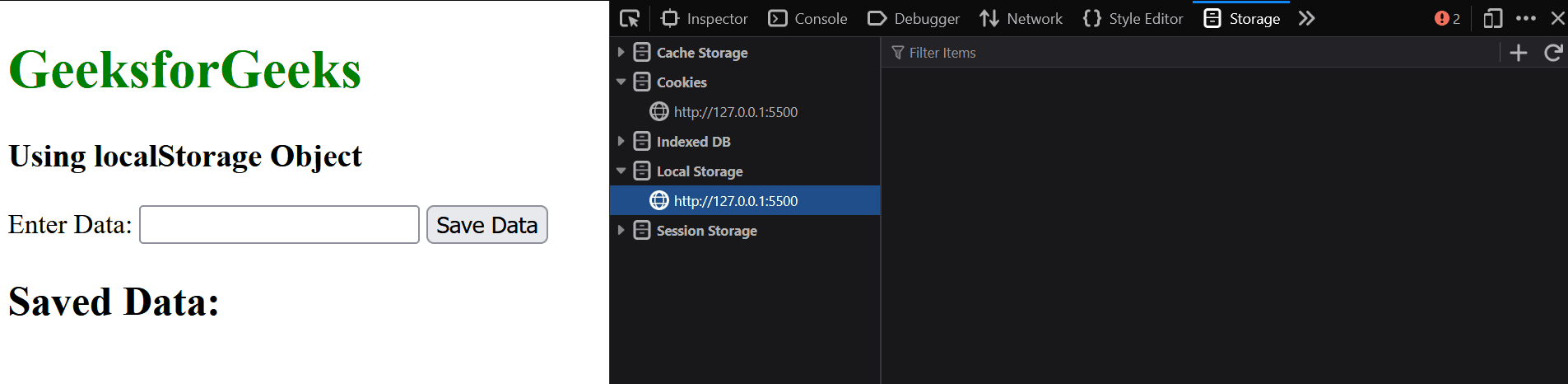
Share your thoughts in the comments
Please Login to comment...