How to Remove Whitespace in Ruby?
Last Updated :
08 Apr, 2024
In this article, we will learn how to remove whitespace in Ruby. Removing whitespace in Ruby eliminates spaces, tabs, carriage returns, and newlines from a string.
Using gsub Method with a Regular Expression
- In this approach, we are using the gsub method with a regular expression \s+ in Ruby.
- This regular expression matches one or more whitespace characters.
- Replacing these matches with an empty string effectively removes all whitespace from the input string uInput. The result is then printed as the space-removed string.
In the below example, the gsub method with a regular expression is used to remove whitespace in ruby.
Ruby
uInput = " Hello, world! "
res = uInput.gsub(/\s+/, "")
puts "Original String: #{uInput}"
puts "Space Removed String: #{res}"
Output:
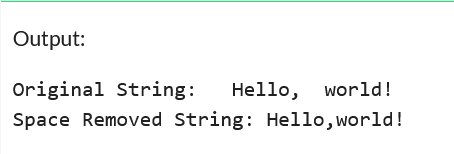
Using Delete Method with Specific Characters
- In this approach, we are using the delete method in Ruby to remove specific characters from a string.
- The characters specified within the delete method (” \t\r\n”) represent whitespace characters such as spaces, tabs, carriage returns, and newlines.
- The resulting string res contains the original string uInput with these specified characters removed.
In the below example the delete method with specific characters is used to remove whitespace in ruby.
Ruby
uInput = " Hello, GeeksforGeeks! "
res = uInput.delete(" \t\r\n")
puts "Original String: #{uInput.inspect}"
puts "Space Removed String: #{res.inspect}"
Output:
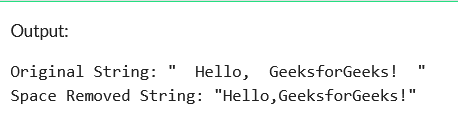
Using the Split and Join Methods
- In this approach, we are using the split method to break the input string uInput into an array of words based on whitespace.
- Then, we use the join method to concatenate the array elements back together without any spaces, creating the space-removed string res.
In the below example the split and join methods are used to remove whitespace in ruby.
Ruby
uInput = " Hello, GFG! "
res = uInput.split.join("")
puts "Original String: #{uInput.inspect}"
puts "Space Removed String: #{res.inspect}"
Output:
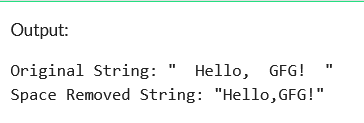
Using Strip Method
- In this approach, we are using the strip method in Ruby, which removes leading and trailing whitespace from the input string.
- By applying uInput.strip, we eliminate the spaces at the beginning and end of the string ” Hello, GFG! “, resulting in the modified string “Hello, GFG!” without leading or trailing spaces.
In the below example the strip method is used to remove whitespace in ruby.
Ruby
uInput = " Hello, GFG! "
res = uInput.strip
puts "Original String: #{uInput.inspect}"
puts "Space Removed String: #{res.inspect}"
Output:
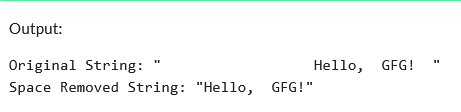
Share your thoughts in the comments
Please Login to comment...