How to Remove Duplicate Elements from Array in Ruby?
Last Updated :
02 Apr, 2024
This article focuses on discussing how to remove duplicate elements from an array in Ruby. In Ruby, an array is a fundamental data structure that stores a collection of elements in a specific order. Various methods and approaches can be used to remove duplicate elements from an array.
Removing Duplicate Elements from an Array in Ruby
Below are the possible approaches to removing duplicate elements from the array in Ruby.
Approach 1: Using the Uniq Method
- In this approach, we are using the uniq method in Ruby to remove duplicate elements from an array.
- The uniq method returns a new array with unique elements, preserving the original order.
- This approach provides a simple and concise way to remove duplicates from an array without modifying the original array.
In the below example, uniq method is used to remove duplicate elements from an array in ruby.
Ruby
arr = [1, 2, 2, 3, 4, 4, 5]
arr_unique = arr.uniq
puts "Array Elements: #{arr.inspect}"
puts "Array after Removing Duplicates: #{arr_unique.inspect}"
Output:
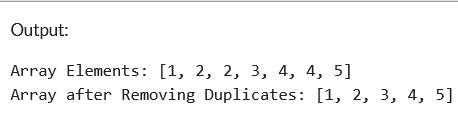
Approach 2: Using Loop
- In this approach, we are using a loop to iterate through the array and filter out duplicate elements.
- The loop checks if each element is already present in the arr_unique array and only adds it if it’s not already included.
- This method manually removes duplicates by comparing each element, resulting in an array with unique elements while preserving the original order.
In the below example, loop is used to remove duplicate elements from array in ruby.
Ruby
arr = [1, 2, 2, 3, 4, 4, 5]
arr_unique = []
arr.each { |element| arr_unique << element unless arr_unique.include?(element) }
puts "Array Elements: #{arr.inspect}"
puts "Array after Removing Duplicates: #{arr_unique.inspect}"
Output:
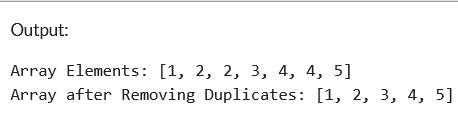
Approach 3: Using | (pipe) Operator
- In this approach, we are using the | (pipe) operator in Ruby to create a new array with unique elements by combining the original array with an empty array [].
- The | operator performs a union operation, ensuring that duplicate elements are removed, resulting in an array with unique elements while maintaining the original order.
In the below example, | (pipe) operator is used to remove duplicate elements from array in ruby.
Ruby
arr = [1, 2, 2, 3, 4, 4, 5]
arr_unique = arr | []
puts "Array Elements: #{arr.inspect}"
puts "Array after Removing Duplicates: #{arr_unique.inspect}"
Output:
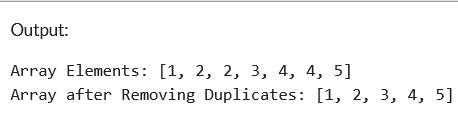
Share your thoughts in the comments
Please Login to comment...