How to Printf in Julia?
Last Updated :
28 Mar, 2024
In Julia, printf is not a built-in function but a macro provided by the Printf module of Julia’s standard library. This macro allows you to format strings similarly to the C programming language’s printf function. The Printf.@printf macro takes a format string followed by zero or more arguments and outputs a formatted string to the standard output.
Syntax:
@printf([io::IO], “%Fmt”, args…)
Print arguments using C style format specification string. Optionally, an IO may be passed as the first argument to redirect output.
Note:
- There is no requirement to import the Printf module in Julia like other modules.
- using Printf is necessary for using the @printf macro.
- Both the macros in the Printf module can also be used with a full path like “Printf.@printf()”.
- Ensure that ‘P’ in Printf is a capital letter and everything else is small.
First type the ‘using Printf’ function in Julia REPL so that we can use the @printf macro ahead:
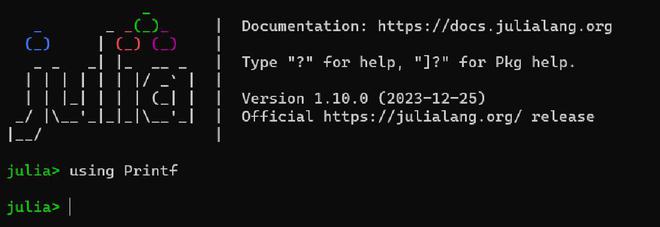
using Printf in Julia REPL
Format Specifiers in Julia
1. String Format Specifier %s
First type @printf then formatted string and format specifier in inverted commas – ” ” and then type arguments in inverted commas.
Note:
Do not use any ‘,’ – comma like C language in Julia and if you want to use comma than make sure that you also use round brackets after @printf.
Without brackets:
Julia
@printf "Geeks for %s" "Geeks"
Output:
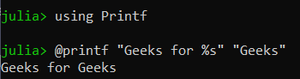
With brackets:
Julia
@printf("Geeks for %s","Geeks")
Output:

2. Character Input Format Specifier %c
Only a single character will be printed even if you provide a string as an argument.
Julia
@printf "Hello %c" "world"
Output:

Note:
Starting in Julia 1.8, %s (string) and %c (character) widths are computed using textwidth, which e.g. ignores zero-width characters (such as combining characters for diacritical marks) and treats certain “wide” characters (e.g. emoji) as width 2.
3. Floating Point Number Format Specifiers %f and %e
Below is the Julia program to implement the floating point numbers using %f:
Julia
@printf "Decimal digits: %f" 1.23456
Output:

Below is the Julia program to implement limiting float to two digits in %f:
Julia
@printf "Decimal two digits: %.2f" 1.23456
Output:

Below is the Julia program to implement the scientific notation using %e:
Julia
@printf "Scientific notation: %e" 1.234
Output:

Below is the Julia program to implement limiting scientific notation to three digits in %e:
Julia
@printf "Scientific notation three digits: %.3e" 1.23456
Output:

4. Padding to Length Format Specifier %i
Below is the Julia program for Padding to length 5 using %i:
Julia
@printf "Padded to length 5 %5i" 123
Output:

Below is the Julia program for Padding with zeros to length 6 using %i:
Julia
@printf "Padded with zeros to length 6 %06i" 123
Output:

All C language format specifiers can be similarly used in Julia.
5. Use of Dynamic Width and Precision
Dynamic width specifiers like %*s and %0*.*f can print width and precision together.
Julia
@printf "Use dynamic width and precision %*.*f" 10 2 0.12345
Output:
Use dynamic width and precision 0.12
Note:
Dynamic width specifiers like %*s and %0*.*f require Julia 1.10.
Caveats in Julia
Inf and NaN are printed consistently as Inf and NaN for flags %a, %A, %e, %E, %f, %F, %g, and %G. Furthermore, if a floating point number is equally close to the numeric values of two possible output strings, the output string further away from zero is chosen.
Julia
@printf("%f %F %f %F", Inf, Inf, NaN, NaN)
Output:

Julia
@printf "%.0f %.1f %f" 0.5 0.025 -0.0078125
Output:

sprintf in Julia
Similarly, like the @printf macro of the Printf module, Julia also has an @sprintf macro in the Printf module. @sprintf macro is used to return the formatted output of @printf as a string.
Syntax:
@sprintf(“%Fmt”, args…)
Julia
@sprintf "This is a %s %15.1f" "number using @sprintf:" 34.567
Output:

Share your thoughts in the comments
Please Login to comment...