How to Redirect Users to Last Active Page before Logout in Angular ?
Last Updated :
01 Aug, 2023
Redirecting the users to the last active page before logout after they log in is the most common feature or functionality in web applications. This functionality enhances the user’s experience by saving their session state and also enables them to resume their activities seamlessly after they log in. In the article, we will see the practical implementation for redirecting users to the last active page before logout after login
Prerequisites:
Before we start, we need to install and configure the tools below to create the Angular application:
- Nodejs and npm
- Angular CLI
- An IDE ( VS Code )
Steps to redirect users to the last active page in Angular Application
The following steps will be implemented to redirect users to the last active page before logout after login in an Angular App:
Step 1: Create an Angular application
Let’s start by using the Angular CLI to generate our base application structure, with routing and a CSS stylesheet.
> ng new AngularApp
? Would you like to add Angular routing? Yes
? Which stylesheet format would you like to use? CSS
. . .
CREATE AngularApp/src/app/app.component.css (0 bytes)
Packages installed successfully.
Successfully initialized git.
Step 2: Creating Directory Structure
Make the directory structure according to the below-added image:
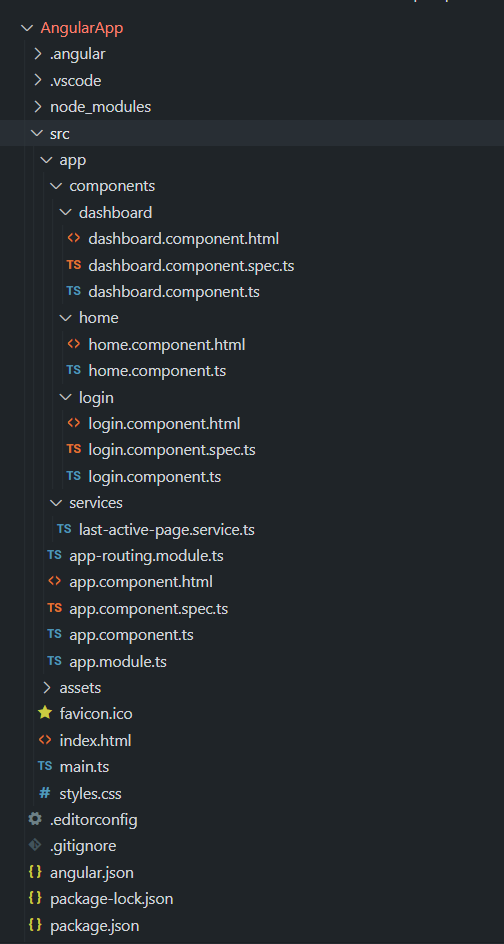
Step 3: Create a Dashboard Component
After the successful creation of the Angular App, now, create the dashboard component in the src/app/components directory by executing the below command and adding the below code in the respective files.
ng generate component dashboard
Javascript
import { Component } from '@angular/core' ;
import { Router } from '@angular/router' ;
import { LastActivePageService }
from '../../services/last-active-page.service' ;
@Component({
selector: 'app-dashboard' ,
templateUrl: './dashboard.component.html' ,
styleUrls: [ './dashboard.component.css' ]
})
export class DashboardComponent {
constructor(private router: Router,
private lastActivePageService: LastActivePageService) { }
logout(): void {
this .lastActivePageService.storeLastActivePage( this .router.url);
this .router.navigateByUrl( '/login' );
}
}
|
HTML
< h2 >Welcome to the Dashboard!</ h2 >
< p >
Click the button to simulate a user logout.
</ p >
< button (click)="logout()">
Logout
</ button >
|
Step 4: Create a Home Component
Create the home component in the src/app/components directory by executing the below command and adding the below code in the respective files.
ng generate component home
Javascript
import { Component } from '@angular/core' ;
import { Router } from '@angular/router' ;
import { LastActivePageService }
from '../../services/last-active-page.service' ;
@Component({
selector: 'app-home-page' ,
templateUrl: './home.component.html' ,
styleUrls: [ './home.component.css' ]
})
export class HomePageComponent {
constructor(private router: Router,
private lastActivePageService: LastActivePageService) { }
logout(): void {
this .lastActivePageService.storeLastActivePage( this .router.url);
this .router.navigateByUrl( '/login' );
}
}
|
HTML
< h1 >Welcome to the Home Page</ h1 >
< p >
Click the button to simulate a
user logout from the home page.
</ p >
< button (click)="logout()">Logout</ button >
|
Step 5: Create a Login Component
Create the login component in the src/app/components directory by executing the below command and adding the below code in the respective files.
ng generate component login
Javascript
import { Component } from '@angular/core' ;
import { Router } from '@angular/router' ;
import { LastActivePageService }
from '../../services/last-active-page.service' ;
@Component({
selector: 'app-login' ,
templateUrl: './login.component.html' ,
styleUrls: [ './login.component.css' ]
})
export class LoginComponent {
username: string = '' ;
password: string = '' ;
constructor(private router: Router,
private lastActivePageService: LastActivePageService) { }
login(): void {
const enteredUsername = this .username.trim();
const enteredPassword = this .password.trim();
if (enteredUsername === 'admin' && enteredPassword === 'admin' ) {
const lastActivePage =
this .lastActivePageService.getLastActivePage();
if (lastActivePage) {
this .router.navigateByUrl(lastActivePage);
} else {
this .router.navigateByUrl( '/dashboard' );
}
} else {
console.log( 'Login failed. Invalid credentials.' );
}
}
}
|
HTML
< h2 >Login</ h2 >
< form (submit)="login()">
< div >
< label for = "username" >Username:</ label >
< input type = "text"
id = "username"
[(ngModel)]="username"
name = "username" required>
</ div >
< div >
< label for = "password" >Password:</ label >
< input type = "password"
id = "password"
[(ngModel)]="password"
name = "password" required>
</ div >
< button type = "submit" >Login</ button >
</ form >
|
Step 6: Create the Last Active Page
Create the last-active-page.service.ts file in the src/app/services directory and add the below code to it.
Javascript
import { Injectable } from '@angular/core' ;
@Injectable({
providedIn: 'root'
})
export class LastActivePageService {
private lastActivePage: string | null = null ;
constructor() { }
storeLastActivePage(url: string): void {
this .lastActivePage = url;
}
getLastActivePage(): string | null {
return this .lastActivePage;
}
clearLastActivePage(): void {
this .lastActivePage = null ;
}
}
|
Step 7: Configuring App Component Files
Now, configure the app files by adding the proper route and rendering. Add the below code in the respective files.
Javascript
import { NgModule } from '@angular/core' ;
import { RouterModule, Routes }
from '@angular/router' ;
import { LoginComponent }
from './components/login/login.component' ;
import { DashboardComponent }
from './components/dashboard/dashboard.component' ;
import { HomePageComponent }
from './components/home/home.component' ;
const routes: Routes = [
{ path: 'login' , component: LoginComponent },
{ path: 'dashboard' , component: DashboardComponent },
{ path: 'home' , component: HomePageComponent },
{ path: '' , redirectTo: '/home' , pathMatch: 'full' },
{ path: '**' , redirectTo: '/home' }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { FormsModule }
from '@angular/forms' ;
import { AppRoutingModule }
from './app-routing.module' ;
import { AppComponent }
from './app.component' ;
import { LoginComponent }
from './components/login/login.component' ;
import { DashboardComponent }
from './components/dashboard/dashboard.component' ;
import { HomePageComponent }
from './components/home/home.component' ;
import { LastActivePageService }
from './services/last-active-page.service' ;
@NgModule({
declarations: [
AppComponent,
LoginComponent,
DashboardComponent,
HomePageComponent
],
imports: [
BrowserModule,
FormsModule,
AppRoutingModule
],
providers: [LastActivePageService],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Javascript
import { Component } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {}
|
HTML
< router-outlet ></ router-outlet >
|
Step 8: Main File
Javascript
import { platformBrowserDynamic }
from '@angular/platform-browser-dynamic' ;
import { AppModule } from './app/app.module' ;
platformBrowserDynamic().bootstrapModule(AppModule)
. catch (err => console.error(err));
|
HTML
<!DOCTYPE html>
< html >
< head >
< title >My Angular App</ title >
< base href = "/" >
< link rel = "stylesheet" href = "styles.css" >
</ head >
< body >
< app-root ></ app-root >
</ body >
</ html >
|
Step 9: Dependency files
{
"compileOnSave": false,
"compilerOptions": {
"baseUrl": "./",
"outDir": "./dist/out-tsc",
"forceConsistentCasingInFileNames": true,
"strict": true,
"noImplicitOverride": true,
"noPropertyAccessFromIndexSignature": true,
"noImplicitReturns": true,
"noFallthroughCasesInSwitch": true,
"sourceMap": true,
"declaration": false,
"downlevelIteration": true,
"experimentalDecorators": true,
"moduleResolution": "node",
"importHelpers": true,
"target": "ES2022",
"module": "ES2022",
"useDefineForClassFields": false,
"lib": [
"ES2022",
"dom"
]
},
"angularCompilerOptions": {
"enableI18nLegacyMessageIdFormat": false,
"strictInjectionParameters": true,
"strictInputAccessModifiers": true,
"strictTemplates": true
}
}
{
"name": "angular-app",
"version": "0.0.0",
"scripts": {
"ng": "ng",
"start": "ng serve",
"build": "ng build",
"watch": "ng build --watch --configuration development",
"test": "ng test"
},
"private": true,
"dependencies": {
"@angular/animations": "^16.1.0",
"@angular/common": "^16.1.0",
"@angular/compiler": "^16.1.0",
"@angular/core": "^16.1.0",
"@angular/forms": "^16.1.0",
"@angular/platform-browser": "^16.1.0",
"@angular/platform-browser-dynamic": "^16.1.0",
"@angular/router": "^16.1.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.13.0"
},
"devDependencies": {
"@angular-devkit/build-angular": "^16.1.5",
"@angular/cli": "~16.1.5",
"@angular/compiler-cli": "^16.1.0",
"@types/jasmine": "~4.3.0",
"jasmine-core": "~4.6.0",
"karma": "~6.4.0",
"karma-chrome-launcher": "~3.2.0",
"karma-coverage": "~2.2.0",
"karma-jasmine": "~5.1.0",
"karma-jasmine-html-reporter": "~2.1.0",
"typescript": "~5.1.3"
}
}
Step 10: Run the application
Finally, we are ready to test our application. Run the application with the command below:
> ng serve
Browser application bundle generation complete.
. . .
** Angular Live Development Server is listening on localhost:4200,
open your browser on http://localhost:4200/ **
√ Compiled successfully.
Explanation:
- After logout, the user is redirected to the login page.
- When the user enters the login credentials and clicks the “Login” button, the application checks if there is any stored last active page before logout.
- If there is a last active page, the user is redirected to that page (either home page or dashboard) as per the last visited page before logout.
- If there is no last active page (i.e., the user directly accessed the login page), the user is redirected to the default dashboard page.
Output:
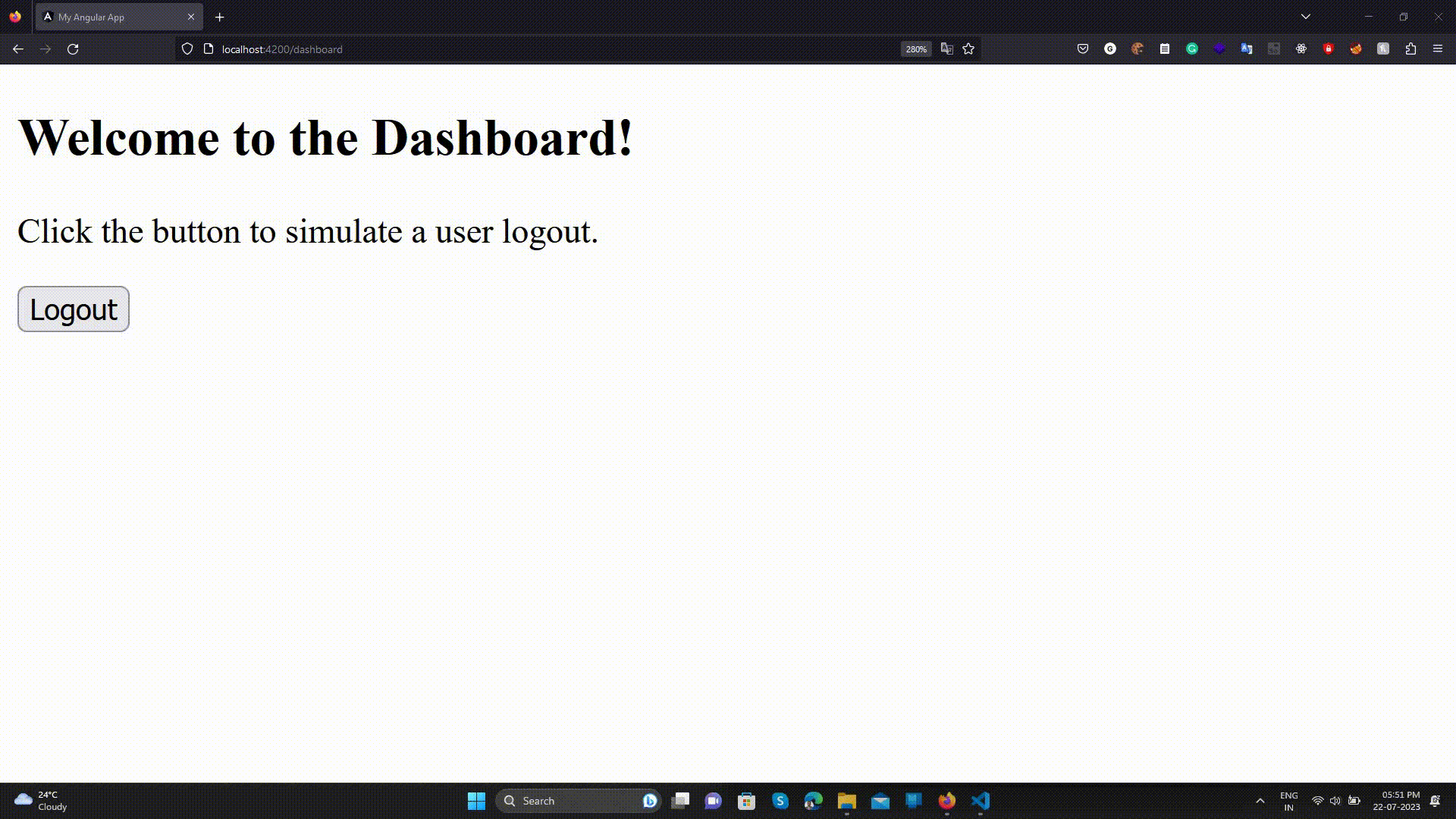
Share your thoughts in the comments
Please Login to comment...