How to Place an Image in AWT in Java
Last Updated :
15 Nov, 2023
AWT stands For Abstract Window Tool Kit. In the AWT we could place the image in the frame using the ToolKit class and MediaTracker class in the java.awt.* package. We can display image formats like GIF or JPG images in the AWT Frame with the help of the following steps in Java.
- Firstly create an Image class object and then load your convenient image into the object using the getImage(“path”) using the ToolKit class.
Loading the image into the object
For loading images into the object we can use the syntax mentioned below.
Syntax:
Image img = ToolKit.getDefaultToolKit().getImage("Path")
Now after the image is completely loaded into the Image class Object with the help of the Graphics class we use the below method to place the image in the frame
Graphics.drawImage(Image Object,int x_coordinate,int y_coordinate,int width,int height,Observer null)
To display an image on the title bar use
Frame.setIconImage(Image Object) .
Program to add an image in AWT
Here we call the getImage() Method with the help of ToolKit.getDefaultToolKit() object. But the main thing is that it takes some time to load the image into the object.
- JVM uses a separate thread for loading the image into the object.
- There is a possibility that the frame will appear without the image we mentioned in the getImage() Method as it completes its execution early.
- We use the MediaTracker class in Java and use the addImage(Image img, int id) method in the MediaTracker class in Java.
- Here the addImage() method takes two parameters which are an Image class object and an id for the following Image class object which takes values such as 0,1,2…n.
Now, JVM will be waiting until the image is completely loaded with the help of MediaTracker.waitForId(int id). The image should be in the same folder or mention the path of the Image.
Below is the implementation of Add Image in AWT:
Java
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class GFG extends Frame{
static Image img;
GFG() {
img = Toolkit.getDefaultToolkit().getImage( "Geeks.png" );
MediaTracker track = new MediaTracker( this );
this .addWindowListener( new WindowAdapter() {
public void windowClosing(WindowEvent e) {
System.exit( 0 );
}
});
track.addImage(img, 0 );
try {
track.waitForID( 0 );
}
catch (InterruptedException ae){
}
}
public void paint(Graphics g){
g.drawImage(img, 150 , 200 , 200 , 200 , null );
}
public static void main(String args[]){
GFG g = new GFG();
g.setTitle( "Geeks For Geeks" );
g.setSize( 500 , 500 );
g.setIconImage(img);
g.setVisible( true );
}
}
|
Output:
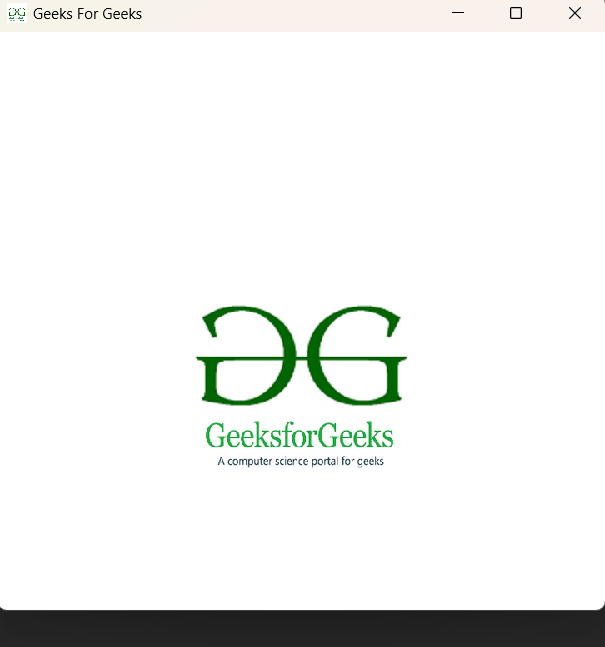
.
Here we can also observe that the image icon has also changed in the frame

The Frame.setIconImage() have also the same image on the Title Bar
This is about placing an image in the AWT Frame on Java and setting the icon image on the title bar in the AWT Frame.
Share your thoughts in the comments
Please Login to comment...