Java AWT or Abstract Window Toolkit is an API used for developing GUI(Graphic User Interfaces) or Window-Based Applications in Java. Java AWT is part of the Java Foundation Classes (JFC) that provides a way to build platform-independent graphical applications.
In this AWT tutorial, you will learn the basics of the AWT, including how to create windows, buttons, labels, and text fields. We will also learn how to add event listeners to components so that they can respond to user input.
By the end of this tutorial, you will have a good understanding of the AWT and be able to create simple GUIs in Java.
Java AWT Basics
Java AWT (Abstract Window Toolkit) is an API used to create Graphical User Interface (GUI) or Windows-based Java programs and Java AWT components are platform-dependent, which means they are shown in accordance with the operating system’s view. AWT is heavyweight, which means that its components consume resources from the underlying operating system (OS). The java.awt package contains AWT API classes such as TextField, Label, TextArea, RadioButton, CheckBox, Choice, List, and so on.
Points about Java AWT components
i. Components of AWT are heavy and platform dependent
ii. AWT has less control as the result can differ because of components are platform dependent.
Why AWT is Platform Independent?
The Java AWT utilizes the native platform subroutine to create API components such as TextField, CheckBox, and buttons. This results in a different visual format for these components on different platforms such as Windows, MAC OS, and Unix. The reason for this is that each platform has a distinct view of its native components. AWT directly calls this native subroutine to create the components, resulting in an AWT application resembling a Windows application on Windows OS, and a Mac application on the MAC OS. In simpler terms, the AWT application’s appearance adapts to the platform it is running on.
AWT is platform independent even after the AWT components are platform dependent because of the points mentioned below:
1. JVM (Java Virtual Machine):
As Java Virtual Machine is platform dependent
2. Abstract APIs:
AWT provides an abstract layer for GUI. Java applications interact with AWT through Abstract API which are platform independent. Abstract API allows Java to isolate platform-specific details, making code portable across different systems.
3. Platform-Independent Libraries:
The Libraries of AWT are written in Java which they are totally platform-independent. Because of this, it ensures that AWT functionality remains consistent across different environments.
Java AWT Hierarchy
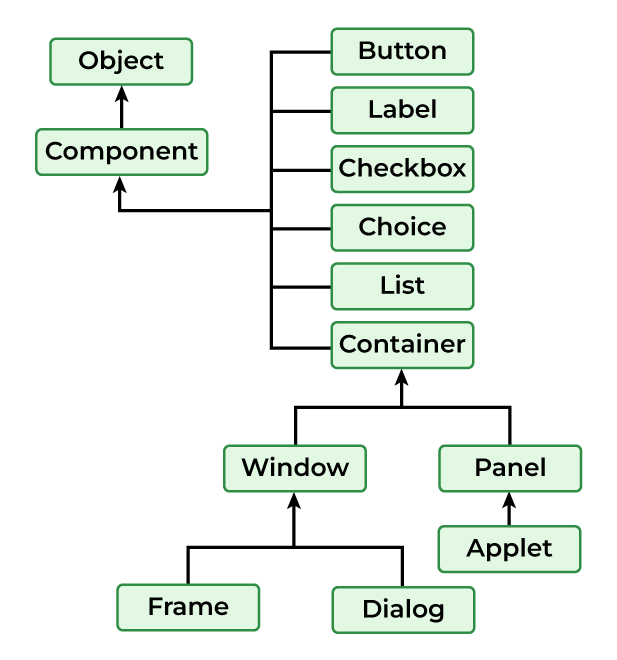
- Components: AWT provides various components such as buttons, labels, text fields, checkboxes, etc used for creating GUI elements for Java Applications.
- Containers: AWT provides containers like panels, frames, and dialogues to organize and group components in the Application.
- Layout Managers: Layout Managers are responsible for arranging data in the containers some of the layout managers are BorderLayout, FlowLayout, etc.
- Event Handling: AWT allows the user to handle the events like mouse clicks, key presses, etc. using event listeners and adapters.
- Graphics and Drawing: It is the feature of AWT that helps to draw shapes, insert images and write text in the components of a Java Application.
Note: Container can be added inside another container as it is type of component.
Types of Containers in Java AWT
There are four types of containers in Java AWT:
- Window: Window is a top-level container that represents a graphical window or dialog box. The Window class extends the Container class, which means it can contain other components, such as buttons, labels, and text fields.
- Panel: Panel is a container class in Java. It is a lightweight container that can be used for grouping other components together within a window or a frame.
- Frame: The Frame is the container that contains the title bar and border and can have menu bars.
- Dialog: A dialog box is a temporary window an application creates to retrieve user input.
Java AWT Tutorial for Beginner & Experienced
Learn the basics of the Abstract Window Toolkit (AWT) in Java, for both beginners and experienced developers.
1. Java AWT Label
Syntax of AWT Label
public class Label extends Component implements Accessible Â
AWT Label Class Constructors
There are three types of Java AWT Label Class
1. Label():
Creates Empty Label.
2. Label(String str):
Constructs a Label with str as its name.
3. Label(String str, int x):
Constructs a label with the specified string and x as the specified alignment
AWT Button is a control component with a label that generates an event when clicked on. Button Class is used for creating a labeled button that is platform-independent.
Syntax of AWT Button
public class Button extends Component implements Accessible Â
Java AWT Button Class Constructors
There are two types of Button class constructors as mentioned below:
1. Button( ):
Creates a Button with no label i.e. showing an empty box as a button.
2. Button(String str):Â
Creates a Button with String str as a label. For example if str=”Click Here” button with show click here as the value.
3. Java AWT TextField
Syntax of AWT TextField:
public class TextField extends TextComponentÂ
TextField Class constructors
There are TextField class constructors are mentioned below:
1. TextField():
Constructs a TextField component.
2. TextField(String text):
Constructs a new text field initialized with the given string str to be displayed.
3. TextField(int col):
Creates a new text field(empty) with the given number of columns (col).
4. TextField(String str, int columns):
Creates a new text field(with String str in the display) with the given number of columns (col).
4. Java AWT Checkbox
Syntax of AWT Checkbox:
public class Checkbox extends Component implements ItemSelectable, Accessible Â
Checkbox Class Constructors
There are certain constructors in the AWT Checkbox class as mentioned below:
1. Checkbox():
Creates a checkbox with no label.
2. Checkbox(String str):
Creates a checkbox with a str label.
3. Checkbox(String str, boolean state, CheckboxGroup group):
Creates a checkbox with the str label, and sets the state in the mentioned group.
5. Java AWT CheckboxGroup
CheckboxGroup Class is used to group together a set of Checkbox.
Syntax of AWT CheckboxGroup:
public class CheckboxGroup extends Object implements Serializable Â
Note: CheckboxGroup enables the use of radio buttons in AWT.
6. Java AWT Choice
The object of the Choice class is used to show a popup menu of choices.
Syntax of AWT Choice:
public class Choice extends Component implements ItemSelectable, AccessibleÂ
AWT Choice Class constructor
Choice(): It creates a new choice menu.
7. Java AWT List
The object of the AWT List class represents a list of text items.
Syntax of Java AWT List:
public class List extends Component implements ItemSelectable, AccessibleÂ
AWT List Class Constructors
The List of class constructors is defined below:
1. List():
Creates a new list.
2. List(int row):
Creates lists for a given number of rows(row).
3. List(int row, Boolean Mode)
Ceates new list initialized that displays the given number of rows.
8. Java AWT Canvas
Syntax of AWT Canvas:
public class Canvas extends Component implements Accessible Â
Canvas Class Constructors
1. Canvas():
Creates new Canvas.
2. Canvas(GraphicConfiguration config):
It creates a new Canvas with the given Graphic configuration.
Syntax of AWT Scrollbar:
public class Scrollbar extends Component implements Adjustable, AccessibleÂ
Java AWT Scrollbar Class Constructors
There are three constructor classes in Java mentioned below:
1. Scrollbar():
  It Creates a new vertical Scrollbar in the Application.
2. Scrollbar(int orientation):
  Creates a new vertical Scrollbar with the given orientation.
3. Scrollbar(int orientation, int value, int visible, int mini, int maxi):
   Creates a new scrollbar with the orientation mentioned with value as the default value and [mini, maxi] as the lower and higher limit.
MenuItem class adds a simple labeled menu item on the menu. The MenuItem class allows you to create individual items that can be added to menus. And Menu is a component used to create a dropdown menu that can contain a list of MenuItem components.
 Syntax of Java AWT MenuItem
public class MenuItem extends MenuComponent implements Accessible Â
Syntax of Java AWT Menu
public class Menu extends MenuItem implements MenuContainer, Accessible Â
Java AWT PopupMenu is a component that is used for dynamically popping up a menu that appears when the user right-clicks or performs any other action on a component.
Syntax of AWT PopupMenu
public class PopupMenu extends Menu implements MenuContainer, Accessible Â
12. Java AWT Panel
Java AWT Panel is a container class used to hold and organize graphical components in a Java Application.
Syntax of Java AWT Panel:
public class Panel extends Container implements Accessible Â
Java AWT Toolkit class provides us with a platform-independent way to access various system resources and functionalities. Subclasses of Toolkit are used to bind various components.
Syntax of Java AWT Toolkit
public abstract class Toolkit extends Object Â
Event Handling Components – Java AWT
Here are some of the event handling components in Java:
1. Java ActionListener
Java ActionListner is a interface which responds to the actions performed by the components like buttons, menu items ,etc.
Syntax of Java ActionListener:
public class ActionListenerExample Implements ActionListener Â
There is only methods associated with ActionListner class that is actionPerformed().
Syntax of actionPerformed() method:
public abstract void actionPerformed(ActionEvent e); Â
2. Java MouseListener
Java MouseListner is a interface that responds to the actions performed by mouse events generated by the user. Example: mouse clicks , mouse movements, etc.
There are 5 Methods associated with MouseListner:
1. mouseClicked(MouseEvent e):
Responds to mouse buttons when clicked on a component in the Application.
2. mousePressed(MouseEvent e):
Responds to mouse button is Pressed on a component in the Application.
3. mouseReleased(MouseEvent e):
Responds to Mouse button released after being pressed over a component in the Application.
4. mouseEntered(MouseEvent e):
Responds to the situation when a Mouse cursor enters the bounds of a component in an Application.
5. mouseExited(MouseEvent e):
Responds to the situation when a Mouse cursor exits a component’s bounds.
3. Java MouseMotionListener
Java MouseMotionListner is a interface which is notified when mouse is moved or dragged.
It contains two Methods mentioned below:
1. mouseDragged(MouseEvent e):
Responds when the mouse is dragged with mouse button clicked over a component in Application.
2. mouseMoved(MouseEvent e):
Responds when the mouse is moved over a component in Application.
4. Java ItemListener
Java ItemListner is an interface which handles events related to item selection and deselection those that occur with checkboxes, radio buttons, etc. There is only one Method associated with ItemListner that is itemStateChanged(). This method provides information about the event, i.e. source of the event and the changed state.
Syntax of itemStateChanged() method:
itemStateChanged(ItemEvent e)
5. Java KeyListener
Java KeyListner is an interface in Java notified whenever you change the state of key or can be said for key related events.
Syntax of KeyListener:
public interface KeyListener extends EventListenerÂ
There are three methods associated with KeyListner as mentioned below:
1. keyPressed (KeyEvent e):
Responds to the event when key is pressed.
2. keyReleased (KeyEvent e):
Responds to the event when the key is released.
3. keyTyped (KeyEvent e):
Responds to the key has been typed.
6. Java WindowListener
Java WindowListener is a interface used for handling events related to window actions. Events like opening , closing, minimizing, etc are handled using WindowListener.
Syntax of WindowListener
public interface WindowListener extends EventListenerÂ
There are seven methods associated with WindowListener as mentioned below:
1. windowActivated (WindowEvent e):
Responds when window is first opened
2. windowClosed (WindowEvent e):
Responds when the user attempts to close the window
3. windowClosing (WindowEvent e):
Responds after a window has been closed
4. windowDeactivated (WindowEvent e):
Responds when a window is minimized
5. windowDeiconified (WindowEvent e):
Responds when a window is restored from a minimized state
6. windowIconified (WindowEvent e):
Responds when a window is activated
7. windowOpened (WindowEvent e):
Responds when a window loses focus
7. Java Adapter classes
Java adapter classes provide the default implementation of listener interfaces.
8. Close AWT Window
At the end we will need to Close AWT Window, So to perform this task we will use dispose() method. This method releases the resources associated with the window and also removes it from the screen.
Java AWT Examples
Here are some Java AWT examples:
1. Hello World in Java AWT
Hello, World is was the first step in learning Java. So, let us program our first Program in Java AWT as Hello World using Labels and Frames.
Below is the implementation of the above method:
Java
// Java AWT Program for Hello World
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
// Driver Class
public class AWT_Example {
// main function
public static void main(String[] args)
{
// Declaring a Frame and Label
Frame frame = new Frame("Basic Program");
Label label = new Label("Hello World!");
// Aligning the label to CENTER
label.setAlignment(Label.CENTER);
// Adding Label and Setting
// the Size of the Frame
frame.add(label);
frame.setSize(300, 300);
// Making the Frame visible
frame.setVisible(true);
// Using WindowListener for closing the window
frame.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e)
{
System.exit(0);
}
});
}
}
Running:
javac AWT_Example.java
java AWT_Example
Output:
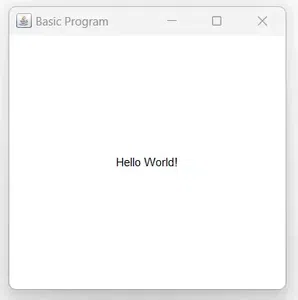
2. Java AWT Program to create Button
Below is the implementation of the Java AWT Program to create a Button:
Java
// Java AWT Program for Button
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
// Driver Class
public class Button_Example {
// main function
public static void main(String[] args)
{
// Creating instance of frame with the label
Frame frame = new Frame("Example 2");
// Creating instance of button with label
Button button = new Button("Click Here");
// Setting the position for the button in frame
button.setBounds(80, 100, 64, 30);
// Adding button to the frame
frame.add(button);
// setting size, layout and visibility of frame
frame.setSize(300, 300);
frame.setLayout(null);
frame.setVisible(true);
// Using WindowListener for closing the window
frame.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e)
{
System.exit(0);
}
});
}
}
Running:
javac Button_Example.java
java Button_Example
Output:
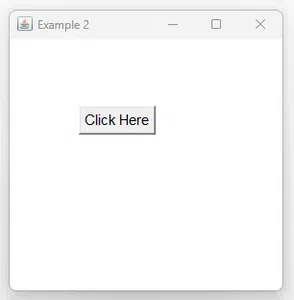
Java AWT Tutorial – FAQs
1. What is AWT in Java?Â
AWT stands for Abstract Window Toolkit, which is an API for creating graphical user interface (GUI) or windows-based applications in Java. AWT provides various components like buttons, labels, text fields, etc. that can be used as objects in a Java program
2. What are the advantages of AWT?Â
Some of the advantages of AWT are:
- It is easy to use and learn for beginners.
- It is compatible with all platforms that support Java.
- It provides a native look and feel for the components.
3. What are the types of containers in AWT?Â
A container is a component that can contain other components. There are four types of containers in AWT:
- Window: a container that has no borders and menu bars. It requires a frame, dialog, or another window to create it.
- Panel: a container that has no title bar, border, or menu bar. It is a generic container for holding components.
- Frame: a container that has a title bar, and border, and can have menu bars. It is the most widely used container for developing AWT applications.
- Dialog: a container that has a title bar, and border, and can have buttons. It is used to display messages or get user input.
4. How to create and use components in AWT?Â
To create and use components in AWT, we need to follow these steps:
- Import the java.awt package, which contains the classes for AWT components.
- Create an instance of the component class, such as Button, Label, TextField, etc.
- Set the properties of the component, such as size, location, text, color, etc.
- Add the component to a container, such as Frame, Panel, etc.
- Set the visibility of the container to true.
Share your thoughts in the comments
Please Login to comment...