How to Optimize Memory Usage and Performance for large HashSet Datasets in Java?
Last Updated :
05 Apr, 2024
HashSet is mainly used for the data structures in Java offers fast access and prevents duplicate elements. So, the size of the dataset will be improved and it can raise memory consumption and performance issues. Now we are discussing exploring the various strategies to optimize memory usage and enhance performance.
When we work with the large HashSet datasets in Java, we will explore the technology like load factor, select the appropriate hash functions, and leverage the solutions of the external memory. By improving the above strategies, developers can manage the memory resources effectively and improve the overall performance of the Java applications that are handling the large HashSet datasets.
Program to Optimize the Performance for Large HashSet Datasets and Memory Usage in Java
Below is the code implementation and its steps to optimize memory usage and performance for large HashSet datasets in Java.
Step 1: Create a Java Project
Open Eclipse IDE, and create a Java Project name it as HashSetOptimization.
Step 2: Create a class file in Java Project
After creating the java project, create a class and name it as HashSetExample. The below figure shows the path for the HashSetExample.java file.
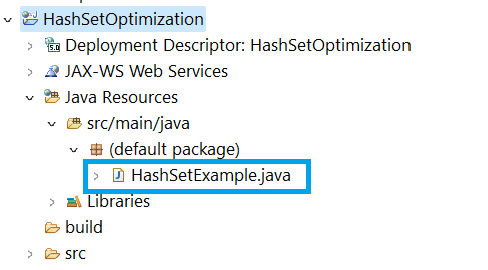
Step 3: Implement the code in java file
Once you create the .java file, open the file and write the below code for execution.
Java
import java.util.HashSet;
public class HashSetExample {
public static void main(String[] args) {
int initialCapacity = 1000000; // Initial capacity for HashSet
float loadFactor = 0.75f; // Default load factor for HashSet
// create HashSet with Initial Capacity and Load Factor
HashSet<Integer> largeSet = new HashSet<>(initialCapacity, loadFactor);
// add Elements to HashSet
for (int i = 0; i < initialCapacity; i++)
{
largeSet.add(i);
}
// Check if an element exists
int elementToCheck = 500000;
boolean containsElement = largeSet.contains(elementToCheck);
System.out.println("HashSet contains element " + elementToCheck + ": " + containsElement);
// Remove an element
int elementToRemove = 999999;
boolean removed = largeSet.remove(elementToRemove);
System.out.println("Element " + elementToRemove + " removed: " + removed);
// Check the size of the HashSet after operations
System.out.println("Size of HashSet after operations: " + largeSet.size());
}
}
Explanation of the above Program:
- In the above code, first we prepare a HashSet to hold the lot of numbers in the HashSet container.
- After that, we initialize the container to make 1,000,000 elements initially.
- If the HashSet will fill up to the 75% then it will make the more space automatically.
- Add the numbers from the 0 to 999,999 into the HashSet container.
- After adding, perform the operations such as checking, removing and counting.
Step 4: Run the Code
- After writing the code in the file, we need to run the program in Run As > Java Application.
- After that we can see the output in our console window as shown below.
Output:
After running the program, we can be able to see the below output in console.
Share your thoughts in the comments
Please Login to comment...