How to mock object in Scala?
Last Updated :
08 May, 2024
In software development, mocking is a technique used to isolate the unit under test by replacing external dependencies with simulated objects or “mocks”. Mocking is particularly useful when working with objects that are difficult to set up or have side effects, such as databases, web services, or file systems.
Scala provides several libraries for mocking objects, including ScalaMock, MockFactory, and EasyMock. In this article, we’ll focus on using ScalaMock, which is a popular and lightweight mocking library for Scala.
Prerequisites to mock object in Scala
1. Add the ScalaMock dependency to your project:
If you’re using SBT, add the following line to your build.sbt file:
libraryDependencies += “org.scalamock” % “scalamock” % “5.1.0” % Test
This will add ScalaMock as a test dependency in your project.
2. Import required classes:
In your test suite file, you need to import the following classes:
import org.scalamock.scalatest.MockFactory
import org.scalatest.flatspec.AnyFlatSpec
- MockFactory provides utility methods to create mock objects.
- AnyFlatSpec is a trait from ScalaTest that provides the testing framework. You can use any other test suite trait from ScalaTest as per your preference.
3. Extend your test suite with MockFactory:
Your test suite class should extend AnyFlatSpec and MockFactory.
For example:
class MyServiceSpec extends AnyFlatSpec with MockFactory {
Your tests go here
}
Extending MockFactory allows you to use the mock method to create mock objects.
4. Define the trait or class you want to mock:
You need to have a trait or class that you want to mock.
For example:
scalaCopy codetrait MyService {
def doSomething(arg: String): String
}
With these prerequisites in place, you can start mocking objects in your tests using ScalaMock.
Example to demonstrate how to mock object in Scala
Step 1: Set up the SBT Build File
Add ScalaMock as a dependency in your SBT build file (build.sbt).
Scala
libraryDependencies += "org.scalamock" %% "scalamock" % "5.1.0" % Test
Step 2: Define the Service Trait and its Implementation
Create a trait and a concrete implementation representing the service you want to mock.
Scala
// DataService.scala
trait DataService {
def fetchData(id: Int): String
}
class DataServiceImpl extends DataService {
override def fetchData(id: Int): String = {
// Implementation to fetch data
s"Actual data for ID $id"
}
}
Step 3: Create a Mock Object using ScalaMock
Create a test class where you’ll mock the behavior of the service using ScalaMock.
Scala
// MockingDataServiceTest.scala
import org.scalamock.scalatest.MockFactory
import org.scalatest.funsuite.AnyFunSuite
class MockingDataServiceTest extends AnyFunSuite with MockFactory {
test("Mocking fetchData") {
// Create a mock instance of the DataService trait
val mockDataService = mock[DataService]
// Stub the behavior of the fetchData method
(mockDataService.fetchData _)
.expects(42)
.returning("Mocked data for ID 42")
// Call the fetchData method on the mock object
val result = mockDataService.fetchData(42)
// Verify the result matches the expected mocked data
assert(result == "Mocked data for ID 42")
// Optionally, verify method invocations
(mockDataService.fetchData _).verify(42).once()
}
}
Output:
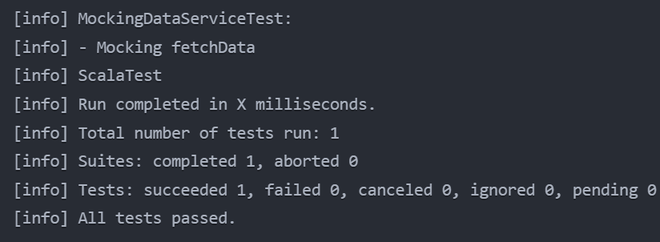
Conclusion
Mocking is an essential technique in software development, particularly when working with unit tests and external dependencies. ScalaMock is a powerful and lightweight library that makes it easy to create mock objects and stub their behavior in Scala. By following the examples in this article, you should be able to start using ScalaMock in your Scala projects and write more robust and maintainable tests.
Share your thoughts in the comments
Please Login to comment...