How to Manipulate DOM using Service Worker ?
Last Updated :
21 Sep, 2023
Using a service worker, indirectly manipulates the DOM through communication with the client page it controls.
DOM (Document Object Model)
In JavaScript, the DOM (document object model) is a programming interface that represents the structure of HTML or XML files as a hierarchical tree shape. It provides a manner to efficaciously access, control, and update the content material and structure of net pages. Read More – DOM.
Service Workers
These are JavaScript scripts that run in the background, separate from web pages, and allow high-performance net applications. They act as a proxy among web pages and the network, allowing community request management, caching, and background processing. Service Workers provide functions like offline assist, push notifications, and background sync is an important part of innovative web packages (PWAs). Read More – Service Workers.
Using Service Workers for DOM Manipulation
Servers are designed to handle network requests and perform background tasks, they do not have direct access to the DOM (Data Structure) like JavaScript running in the content of a web page. Therefore, it is not possible to manipulate the DOM directly using Operators. The most common service providers are events, caching, and push notifications.
However, you can use service workers to intercept network requests made by web pages and modify the responses before they reach the web page. This can indirectly affect the DOM by modifying the data that the web page receives.
Syntax and Approach
Here’s a general outline of how you can achieve this –
- Register the service worker: In your web page’s JavaScript code, register the service worker using the navigator.serviceWorker.register() method. This tells the browser to start using the service worker for certain URLs or routes.
navigator.serviceWorker.register('service-worker.js')
.then(registration => {
console.log('Service worker registered!');
})
.catch(error => {
console.error('Failed to register service worker:', error);
});
- Implement the service worker: Create a JavaScript file (e.g., service-worker.js) and define the service worker logic inside it. The main event you’ll use is the fetch event, which allows you to intercept and modify network requests and responses.
self.addEventListener('fetch', event => {
event.respondWith(
fetch(event.request)
.then(response => {
// Modify the response here if needed
// For example, you can modify the
// response body or headers
return response;
})
.catch(error => {
console.error('Failed to fetch:', error);
return new Response('An error occurred.');
})
);
});
- Modify the response: Inside the fetch event handler, you can modify the response before it’s returned to the web page. For example, you can modify the response body or headers, or inject additional JavaScript code. Note that manipulating the response can be complex and may require knowledge of the specific data format (e.g., JSON, HTML) and how the web page consumes it.
- Install and activate the service worker: Load your web page in the browser, and the service worker will be installed and activated. Once activated, the service worker can intercept network requests and modify responses according to your logic.
It’s important to note that service workers have certain limitations and security restrictions to prevent abuse. They are designed to enhance performance and offline capabilities rather than directly manipulating the DOM.
Example 1: Updating the Title of the Page.
Javascript
self.addEventListener( 'fetch' , (event) => {
event.respondWith(
fetch(event.request).then((response) => {
self.clients.matchAll().then((clients) => {
clients.forEach((client) => {
client.postMessage({
type: 'updateTitle' ,
data: 'New Title'
})
})
})
return response
})
)
})
|
Javascript
if ( 'serviceWorker' in navigator) {
navigator.serviceWorker
.register( '/serviceWorker.js' )
.then( function (registration) {
console.log(
'You have successfully registered your service worker : ' ,
registration
)
})
. catch ( function (error) {
console.log(
'Your service worker registration failed: ' , error)
})
}
navigator.serviceWorker.addEventListener( 'message' ,
(event) => {
if (event.data && event.data.type === 'updateTitle' ) {
document.title = event.data.data
}
})
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" />
</ head >
< body class = "" >
< h1 >Geek For Geeks</ h1 >
</ body >
< script src = "script.js" ></ script >
</ html >
|
Output: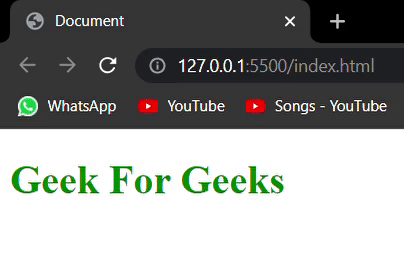
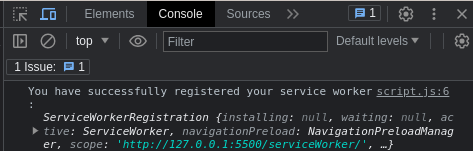
Service worker console response
Example 2: Changing the Background Color of the Body.
Javascript
if ( 'serviceWorker' in navigator) {
navigator.serviceWorker
.register( '/serviceWorker.js' )
.then((registration) => {
console.log( 'Service worker registered!' )
})
. catch ((error) => {
console.error(
'Service worker registration failed:' , error)
})
}
document.getElementById( 'button' )
.addEventListener( 'click' , () => {
navigator.serviceWorker.controller
.postMessage({ type: 'changeDOM' })
})
navigator.serviceWorker.addEventListener( 'message' ,
(event) => {
if (event.data && event.data.type === 'domChanged' ) {
document.getElementById( 'myElement' ).textContent
= event.data.data
}
})
|
Javascript
self.addEventListener( 'message' , (event) => {
if (event.data && event.data.type === 'changeDOM' ) {
const newContent = 'New content after button click'
self.clients.matchAll().then((clients) => {
clients.forEach((client) => {
client.postMessage({
type: 'domChanged' ,
data: newContent
})
})
})
}
})
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" />
< link rel = "stylesheet" href = "style.css" />
</ head >
< body class = "" >
< h1 id = "h1" >Geek For Geeks</ h1 >
< h3 id = "myElement" ></ h3 >
< button id = "button" >Click Me</ button >
< script src = "script.js" ></ script >
</ body >
</ html >
|
Output: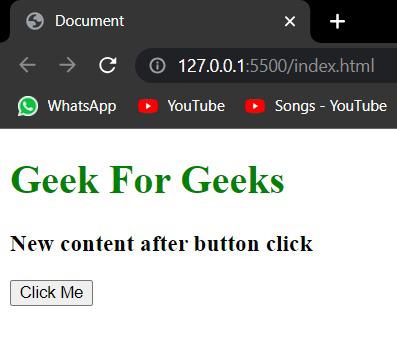
Share your thoughts in the comments
Please Login to comment...