How to Make Visited Link Unvisited in HTML ?
Last Updated :
01 Apr, 2024
When users interact with links on a webpage, then their browsers keep track of the state of these links. Understanding and managing these states can be a crucial task. We will focus on how to make a visited link appear unvisited again using HTML.
Approach
- First, create a basic structure with different HTML elements including <h1>, <p>, <a>, and <button>.
- The HTML defines a link with the ID “link” pointing to “geeksforgeeks.org” and an onclick event calling the function “changeLinkColor()”.
- The link element with the ID “link” directs to “geeksforgeeks.org” and triggers the function “changeLinkColor()” upon click.
- To reset the color of a visited link, you can create another function like “resetLinkColor()”.
- This function selects the link element and changes its color from black to the default value i.e., blue.
Example: This example shows the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Change Link Color on Click</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: white;
margin: 0;
padding: 0;
}
h2 {
text-align: center;
margin-top: 20px;
}
p {
text-align: center;
}
#link {
color: blue;
text-decoration: none;
}
button {
background-color: #007bff;
border: none;
color: white;
padding: 10px 20px;
cursor: pointer;
border-radius: 5px;
margin-top: 20px;
display: block;
margin-left: auto;
margin-right: auto;
}
button:hover {
background-color: #0056b3;
}
</style>
</head>
<body>
<h2>Change Link Color on Click</h2>
<!-- Link to geeksforgeeks.org -->
<p>
<a id="link" href="https://geeksforgeeks.org"
onclick="changeLinkColor()"
target="_blank">
Visit GeeksforGeeks
</a>
</p>
<!-- Button to reset link color -->
<h2>Make visited link unvisited in HTML</h2>
<button onclick="resetLinkColor()">
Reset Color
</button>
<script>
function changeLinkColor() {
let linkElement = document.getElementById("link");
linkElement.style.color = "red";
}
function resetLinkColor() {
let reset_link = document.getElementById("link");
reset_link.style.color = "blue";
}
</script>
</body>
</html>
Output:
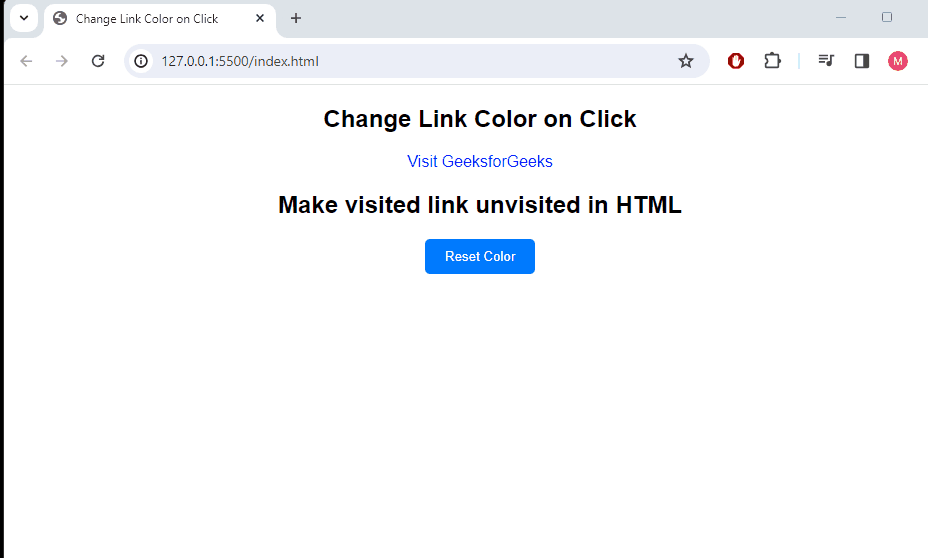
Share your thoughts in the comments
Please Login to comment...