How to make a Todo App using PHP & MySQL ?
Last Updated :
11 Mar, 2024
To create a Todo App using PHP and MySQL, you’ll first need to set up a MySQL database to store the tasks. Then, use PHP to create a web interface where users can add, edit, and delete tasks. PHP will handle the backend logic, such as connecting to the database and performing CRUD operations. Finally, use HTML and CSS to design the frontend interface, making it user-friendly and visually appealing.
Prerequisites
Note: First, you need to install a local server like XAMPP to run PHP scripts on your device. After setting up the server, follow the steps below.
Setup the Database
Run the local server and create a New Database namely “todo” in it. Once the database is created, create a table named “task” in this database. Use the code mentioned below to create this table.
CREATE TABLE `task` (
`task_id` int(10) PRIMARY KEY,
`task` varchar(250) NOT NULL,
`status` varchar(30) NOT NULL
);
INSERT INTO `task` VALUES
(1, 'Read an article on React.js', 'Done'),
(2, 'Organize a meeting', 'Pending');
ALTER TABLE `task`
MODIFY `task_id` int(10) AUTO_INCREMENT, AUTO_INCREMENT=3;
Create a connection with Database
Inside the folder, create a file named – “config.php”. Inside this file used the below mentioned code to establish a connection with the database. So that you can access the database directly from the application.
- mysqli_connect( ) function is used to create the connection. It takes 4 parameter – hostname, username, password and database name, in the same order as mentioned.
- die( ) function terminates the execution if there an error while connecting to the database.
<?php
$db = mysqli_connect("localhost", "root", "", "todo")
or
die("Connection failed: " . mysqli_connect_error());
?>
Example: Implementation to create a todo app.
CSS
// style.css
* {
margin : 0 ;
padding : 0 ;
font-family : monospace ;
}
body {
background-color : olivedrab;
}
nav {
background-color : rgb ( 0 , 90 , 0 );
margin-bottom : 50px ;
}
.heading {
text-decoration : none ;
color : white ;
letter-spacing : 1px ;
font-size : 30px ;
font-weight : 900 ;
display : flex;
justify- content : center ;
padding : 10px ;
}
.container {
display : flex;
flex- direction : column;
justify- content : center ;
align-items: center ;
}
.input-area {
margin-bottom : 30px ;
}
input {
width : 500px ;
height : 30px ;
border : none ;
border-radius: 10px ;
padding : 5px ;
}
.btn {
width : 100px ;
height : 40px ;
border-radius: 50px ;
border : 1px solid rgb ( 0 , 90 , 0 );
color : white ;
font-weight : 900 ;
background-color : rgb ( 0 , 90 , 0 );
}
table {
border : 0.5px solid black ;
width : 700px ;
padding : 10px ;
border-radius: 5px ;
background-color : whitesmoke;
}
th {
font-size : 20px ;
text-align : start;
padding-top : 10px ;
padding-bottom : 10px ;
}
th:nth-child( 4 ) {
text-align : center ;
}
tr.border- bottom td {
border-bottom : 1pt dashed black ;
}
tbody {
padding : 3px ;
font-size : 15px ;
}
td {
padding : 5px ;
}
.action {
display : flex;
flex- direction : row;
justify- content : center ;
align-items: center ;
gap: 20px ;
}
.btn-completed {
text-decoration : none ;
}
.btn-remove {
text-decoration : none ;
}
|
PHP
<!DOCTYPE html>
<html lang= "en" >
<head>
<title>Todo List</title>
<link rel= "stylesheet"
type= "text/css" href= "style.css" />
</head>
<body>
<nav>
<a class = "heading" href= "#" >ToDo App</a>
</nav>
<div class = "container" >
<div class = "input-area" >
<form method= "POST" action= "add_task.php" >
<input type= "text" name= "task"
placeholder= "write your tasks here..." required />
<button class = "btn" name= "add" >
Add Task
</button>
</form>
</div>
<table class = "table" >
<thead>
<tr>
<th>#</th>
<th>Tasks</th>
<th>Status</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<?php
require 'config.php' ;
$fetchingtasks =
mysqli_query( $db , "SELECT * FROM `task` ORDER BY `task_id` ASC" )
or die (mysqli_error( $db ));
$count = 1;
while ( $fetch = $fetchingtasks ->fetch_array()) {
?>
<tr class = "border-bottom" >
<td>
<?php echo $count ++ ?>
</td>
<td>
<?php echo $fetch [ 'task' ] ?>
</td>
<td>
<?php echo $fetch [ 'status' ] ?>
</td>
<td colspan= "2" class = "action" >
<?php
if ( $fetch [ 'status' ] != "Done" )
{
echo
'<a href="update_task.php?task_id=' . $fetch [ 'task_id' ] . '"
class = "btn-completed" >✅</a>';
}
?>
<a href=
"delete_task.php?task_id=<?php echo $fetch['task_id'] ?>"
class = "btn-remove" >
❌
</a>
</td>
</tr>
<?php
}
?>
</tbody>
</table>
</div>
</body>
</html>
|
PHP
<?php
require_once 'config.php' ;
if (isset( $_POST [ 'add' ])) {
if ( $_POST [ 'task' ] != "" ) {
$task = $_POST [ 'task' ];
$addtasks = mysqli_query( $db ,
"INSERT INTO `task` VALUES('', '$task', 'Pending')" )
or
die (mysqli_error( $db ));
header( 'location:index.php' );
}
}
?>
|
PHP
<?php
require_once 'config.php' ;
if ( $_GET [ 'task_id' ]) {
$task_id = $_GET [ 'task_id' ];
$deletingtasks = mysqli_query( $db ,
"DELETE FROM `task` WHERE `task_id` = $task_id" )
or
die (mysqli_error( $db ));
header( "location: index.php" );
}
?>
|
PHP
<?php
require_once 'config.php' ;
if ( $_GET [ 'task_id' ] != "" ) {
$task_id = $_GET [ 'task_id' ];
$updatingtasks =
mysqli_query( $db ,
"UPDATE `task` SET `status` = 'Done' WHERE `task_id` = $task_id" )
or
die (mysqli_error( $db ));
header( 'location: index.php' );
}
?>
|
Output:
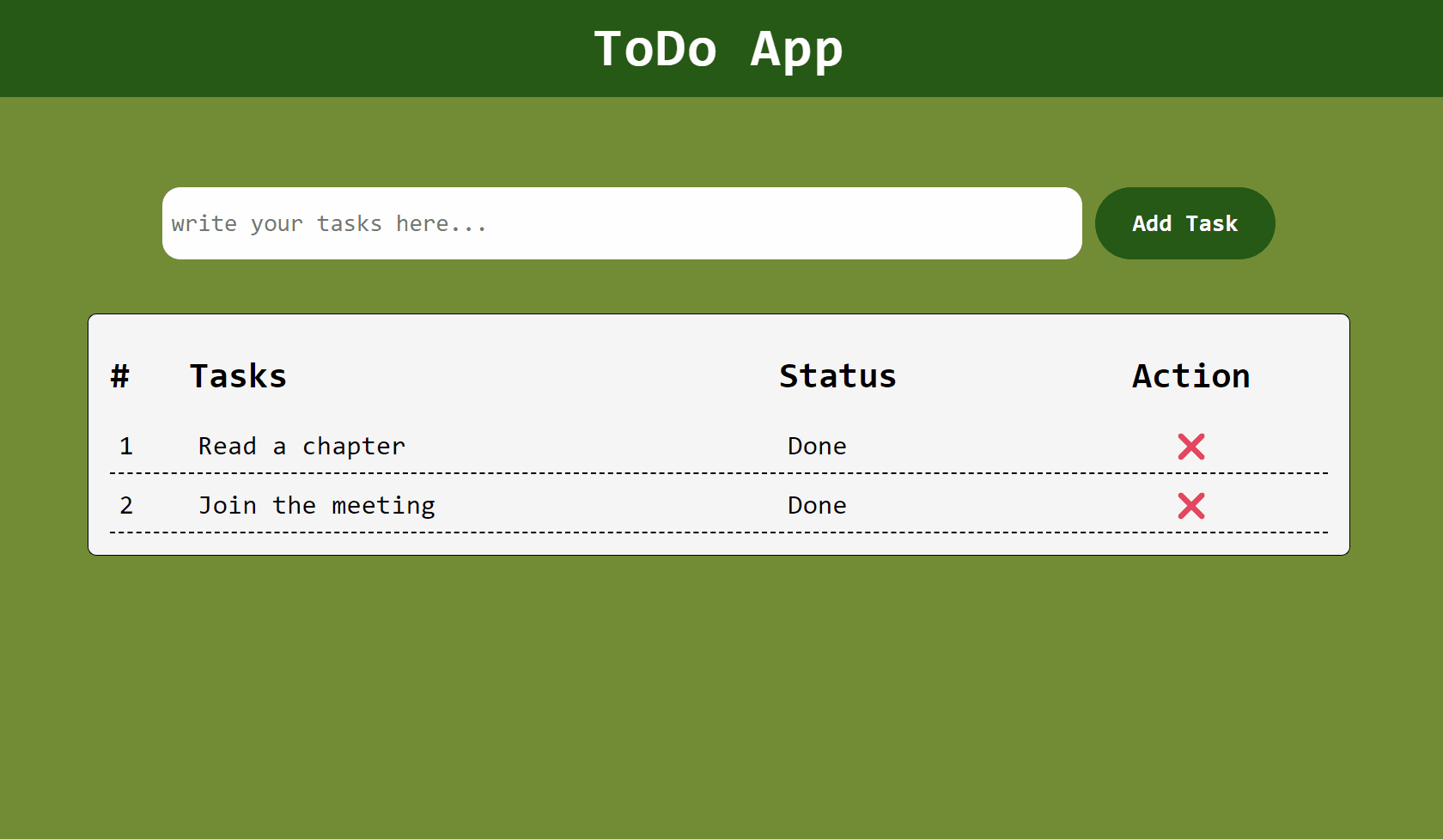
ToDo App using PHP and MySQL
Share your thoughts in the comments
Please Login to comment...