How to Lock a File in Java?
Last Updated :
03 Apr, 2024
In Java, file locking involves stopping processes or threads from changing a file. It comes in handy for threaded applications that require simultaneous access, to a file or to safeguard a file from modifications while it’s being used by our application.
When reading or writing files we need to make sure proper file locking mechanisms are in place. This ensures data integrity in concurrent I/O-based applications.
Approach to lock a File in Java:
- In Java, to lock a file, we need to get a FileChannel for the file we want to lock and use the lock() method of the FileChannel to acquire a lock on the file.
- This lock() method blocks until it can retrieve the lock.
- If we want to try to acquire the lock without blocking, we can use the lock() method instead.
- This method returns a null or throws an exception if the file is already locked.
- Release the lock using the release() method of the FileLock Object.
- Then we need to close the file channel.
Program to Lock a File in Java
Here we can see one example for lock a file by using lock() method and Filechannel.
Java
// Java program to lock a file using
// lock() method and FileChannel
import java.io.IOException;
import java.io.RandomAccessFile;
import java.nio.channels.FileChannel;
import java.nio.channels.FileLock;
public class FileLock {
public static void main(String[] args) {
String filePath = "C:\\Users\\Geeks Author\\Downloads\\geeksforgeeks.txt";
try (RandomAccessFile file = new RandomAccessFile(filePath, "rw");
FileChannel channel = file.getChannel()) {
// Acquiring an exclusive lock on the file
FileLock fileLock = channel.lock();
System.out.println("File locked successfully");
// Release the lock when done
fileLock.release();
System.out.println("File lock released.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
File locked successfully
File lock released.
Below we can refer the console output.
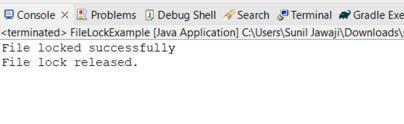
Explanation of the Program:
- We have given one String filePath, here we can give the filePath in String quotes whatever in your local system and replace the actual path of the file we want to lock.
- RandomAccessfile using and obtains a FileChannel from it and then acquires an exclusive lock on the file using channel.lock() method.
- After that, we perform the operations of the locked files, and it releases the lock using fileLock.release() method.
- Once all code is running successfully, we can get output for this File locked successfully and File lock released as shown in the output.
Share your thoughts in the comments
Please Login to comment...