How to Load XML from JavaScript ?
Last Updated :
23 Apr, 2024
Loading XML data into JavaScript is a common task, whether it’s for parsing user input or fetching data from a server.
The below-listed approaches can be used to load XML from JavaScript.
Parsing XML String Directly
This approach involves directly parsing an XML string within the JavaScript code.
- It’s suitable when you already have the XML data available as a string, such as from user input or a pre-defined source.
- It utilizes the DOM Parser object in JavaScript to parse the XML string.
- Allows for manipulation of the parsed XML document using standard DOM methods.
Example: The below code will directly parse the XML string using JavaScript.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content=
"width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<h1>
How to Load XML from JavaScript?
</h1>
<script>
const xmlString =
`<tutorials>
<tutorial>
<id>1</id>
<title>
Introduction to JavaScript
</title>
</tutorial>
<tutorial>
<id>2</id>
<title>
Advanced CSS Techniques
</title>
</tutorial>
</tutorials>`;
const parser = new DOMParser();
const xmlDoc = parser.
parseFromString(xmlString, "text/xml");
const tutorialsNode = xmlDoc.
querySelector("tutorials");
const tutorials = tutorialsNode.
querySelectorAll("tutorial");
tutorials.forEach((tutorial) => {
const id = tutorial.
querySelector("id").textContent;
const title = tutorial.
querySelector("title").textContent;
console.log(
`Tutorial ID: ${id}, Title: ${title}`);
});
</script>
</body>
</html>
Output:

Fetching XML Data from an External Source
In this approach, XML data is fetched from an external source, typically a server, using the fetch API.
- Suitable for scenarios where XML data needs to be dynamically retrieved from a server.
- Handles the response from the server using promises and interprets the response as text.
- Proceeds with parsing the XML string similar to the direct parsing method after receiving the data from the server.
Example: The below code fetches XML data from external resource and parses it using JavaScript.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content=
"width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<h1>
How to Load XML from JavaScript?
</h1>
<script>
fetch("tutorials.xml")
.then((response) => response.text())
.then((xmlString) => {
const parser = new DOMParser();
const xmlDoc = parser.
parseFromString(xmlString, "text/xml");
const tutorials = xmlDoc.
querySelectorAll("tutorial");
tutorials.forEach((tutorial) => {
const id = tutorial.
querySelector("id").textContent;
const title = tutorial.
querySelector("title").textContent;
console.log(
`Tutorial ID: ${id}, Title: ${title}`);
});
});
</script>
</body>
</html>
XML
<!-- tutorials.xml -->
<tutorials>
<tutorial>
<id>1</id>
<title>Introduction to JavaScript</title>
</tutorial>
<tutorial>
<id>2</id>
<title>Advanced CSS Techniques</title>
</tutorial>
</tutorials>
Output:

Parsing XML in Node.js using xml2js
In this approach, we utilize the xml2js module, which is specifically designed for parsing XML in a Node.js environment.
- We use the fs module to read the XML file from the file system.
- After reading the XML data, we use xml2js.parseString() to parse the XML data into a JavaScript object.
- We then access and process the parsed XML data as required.
Steps to setup xml2js using nodejs to parse XML:
Step 1: Create a new directory for your project.
mkdir xml-parser
cd xml-parser
Step 2: Initialize a new Node.js project.
npm init -y
Step 3: Install the required dependencies.
npm install xml2js
Step 4: Create a xml file named tutorials.xml in your project directory and add the XML content you provided in your example.
Step 5: Create a JavaScript file named index.js in your project directory and add the provided JavaScript code to it.
Step 6: Run your application.
node index.js
Example: The below code can be used in you xml and JavaScript file once you have done with all the suggested steps.
JavaScript
// index.js
const fs = require("fs");
const xml2js = require("xml2js");
fs.readFile("tutorials.xml", "utf-8",
(err, data) => {
if (err) {
console.error(err);
return;
}
xml2js.parseString(data,
(err, result) => {
if (err) {
console.error(err);
return;
}
const tutorials =
result.tutorials.
tutorial;
tutorials.forEach(
(tutorial) => {
const id =
tutorial.id[0];
const title =
tutorial.title[0];
console.log(
`Tutorial ID: ${id},
Title: ${title}`);
});
});
});
XML
<!-- tutorials.xml -->
<tutorials>
<tutorial>
<id>1</id>
<title>Introduction to JavaScript</title>
</tutorial>
<tutorial>
<id>2</id>
<title>Advanced CSS Techniques</title>
</tutorial>
</tutorials>
Output: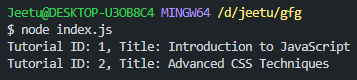
Share your thoughts in the comments
Please Login to comment...