How to create XML Dynamically using JavaScript?
Last Updated :
24 Apr, 2024
XML stands for Extensible Markup Language. It is a popular format for storing and exchanging data on the web. It provides a structured way to represent data that is both human-readable and machine-readable.
There are various approaches to create XML Dynamically using JavaScript:
Using DOM Manipulation
In this approach, We are going to use Document Object Model (DOM) to dynamically create XML elements. This approach allows you to programmatically construct XML structures by creating elements, setting attributes, and appending them to parent nodes.
Example: This example demonstrate the using of DOM Manipulation Approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Dynamic XML Creation using DOM Manipulation</title>
</head>
<body>
<h2 style="color: green;">
GeeksForGeeks | Dynamic XML Creation
using DOM Manipulation
</h2>
<p id="xmlOutput"></p>
<script>
// Create XML document
let xmlDoc = document
.implementation
.createDocument(null, "root");
// Create elements
let element1 = xmlDoc
.createElement("employee");
let element2 = xmlDoc
.createElement("name");
let element3 = xmlDoc
.createElement("age");
// Create text nodes
let textNode1 = xmlDoc
.createTextNode("abc xyz");
let textNode2 = xmlDoc
.createTextNode("21");
element2
.appendChild(textNode1);
element3
.appendChild(textNode2);
// Append elements to root element
element1
.appendChild(element2);
element1
.appendChild(element3);
xmlDoc
.documentElement
.appendChild(element1);
let xmlString = new XMLSerializer()
.serializeToString(xmlDoc);
// Display XML string
console.log(xmlString);
document
.getElementById("xmlOutput")
.innerText = xmlString;
</script>
</body>
</html>
Output:

Using Template Literals
In this approach, we are going to use Template Literals it involves defining XML structures using JavaScript template literals. Template literals provide a convenient way to create multi-line strings with embedded expressions.
Example: This example demonstrate the using of Template Literals Approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Dynamic XML Creation using Template Literals</title>
</head>
<body>
<h2 style="color: green;">
GeeksForGeeks | Dynamic XML Creation using
Template Literals
</h2>
<p id="xmlOutput"></p>
<script>
let name = 'abc xyz'
let age = 21
// Define XML structure
// using template literals
let xmlString = `
<root>
<employee>
<name>${name}</name>
<age>${age}</age>
</employee>
</root>`;
// Display XML string
console.log(xmlString);
document
.getElementById("xmlOutput")
.innerText = xmlString;
</script>
</body>
</html>
Output:
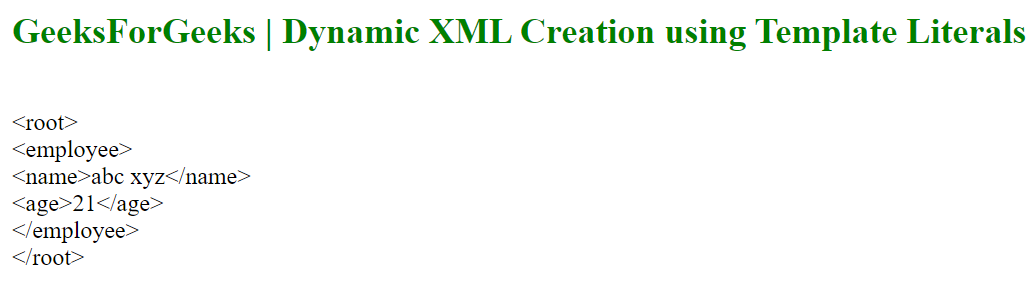
Share your thoughts in the comments
Please Login to comment...