How to Load NextJS Images from an External URL
Last Updated :
22 Apr, 2024
In Next.js, loading external URL images is important because it allows developers to incorporate dynamic content and enrich user experiences by displaying images from various sources seamlessly within their applications.
In this article, we will explore two different approaches to loading NextJS Images from an External URL.
Steps to Create the Next.js Application
Step 1: Set up React Project using the Command:
npx create-next-app external-image
Step 2: Navigate to the Project folder using:
cd external-image
Project Structure:
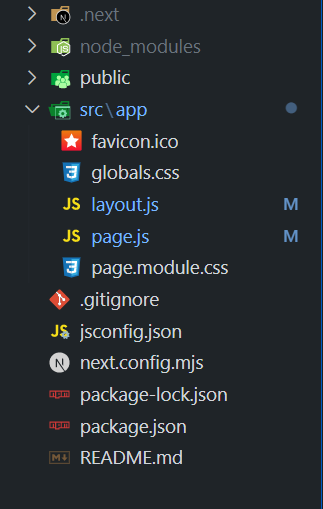
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18",
"react-dom": "^18",
"next": "14.2.1"
}
Approach 1: Using next/Image Component
In this approach, we are using the next/image component in a Next.js application to load an external image from a specified URL. This component optimizes image loading and provides control over dimensions.
Example: The below example uses the next/Image Component to Load NextJS Images from an External URL.
JavaScript
//page.js
"use client";
import React, { useState } from 'react';
import Image from 'next/image';
const Page = () => {
const [showImage, setShowImage] = useState(false);
const btnFn = () => {
setShowImage(true);
};
return (
<div>
<h1>Approach 1: Using next/Image Component</h1>
{ !showImage && (
<button onClick={btnFn}>Load Image</button>
)
}
{ showImage && (
<Image src=
"https://media.geeksforgeeks.org/wp-content/uploads/20230816191453/gfglogo.png"
alt="External Image"
unoptimized
width={300}
height={200}
/>
)}
</div>
);
};
export default Page;
Step to run the application: Now run the application with the below command:
npm run dev
Output:
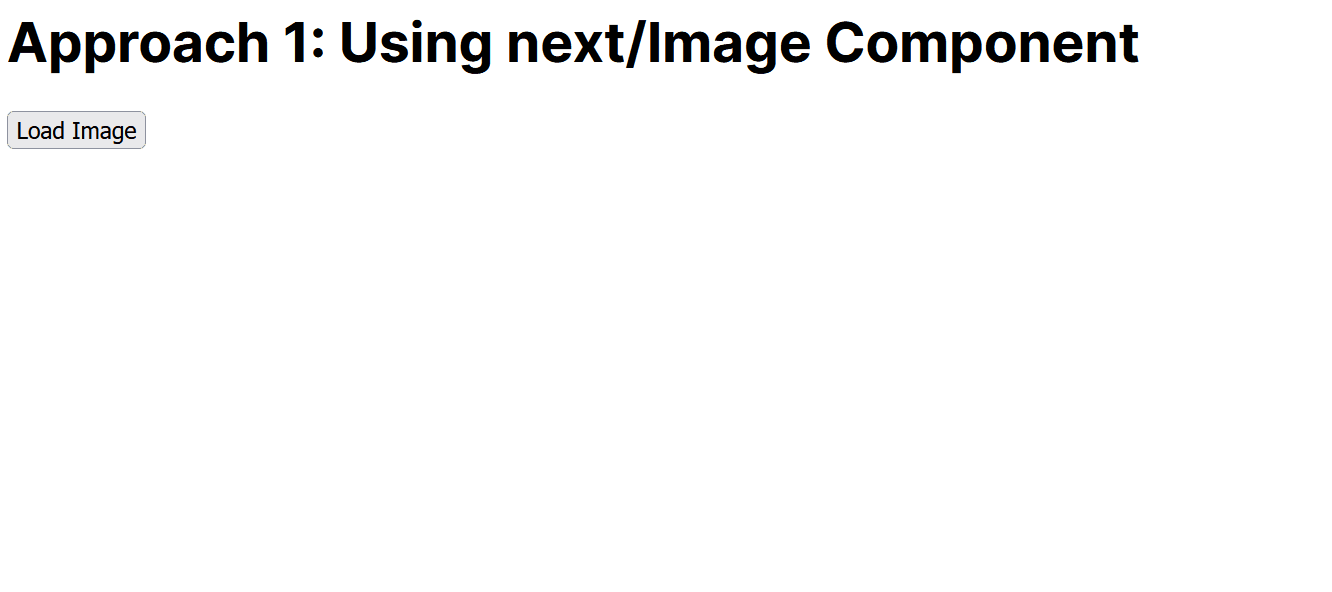
Approach 2: Using an <img> Tag
In this approach, we are using React state (useState) to manage the image source (imageSrc) dynamically based on user button clicks, allowing the loading of external images into a <img> tag within a Next.js application.
Example: The below example uses the <img> Tag to Load NextJS Images from an External URL.
JavaScript
//page.js
"use client";
import React, { useState } from 'react';
const Page = () => {
const [imageSrc, setImageSrc] = useState('');
const loadFn = (imageUrl) => {
setImageSrc(imageUrl);
};
return (
<div>
<h1>Using an <img> Tag</h1>
<div>
<button onClick={() =>
loadFn('https://media.geeksforgeeks.org/wp-content/uploads/20230816191453/gfglogo.png')}>
Load Image 1
</button>
<button onClick={() =>
loadFn('https://media.geeksforgeeks.org/wp-content/uploads/20240419201444/scalaPng.png')}>
Load Image 2
</button>
</div>
{imageSrc && (
<div>
<h3>Loaded Image:</h3>
<img src={imageSrc} alt="External Image" width={300} height={200} />
</div>
)}
</div>
);
};
export default Page;
Step to run the application: Now run the application with the below command:
npm run dev
Output:
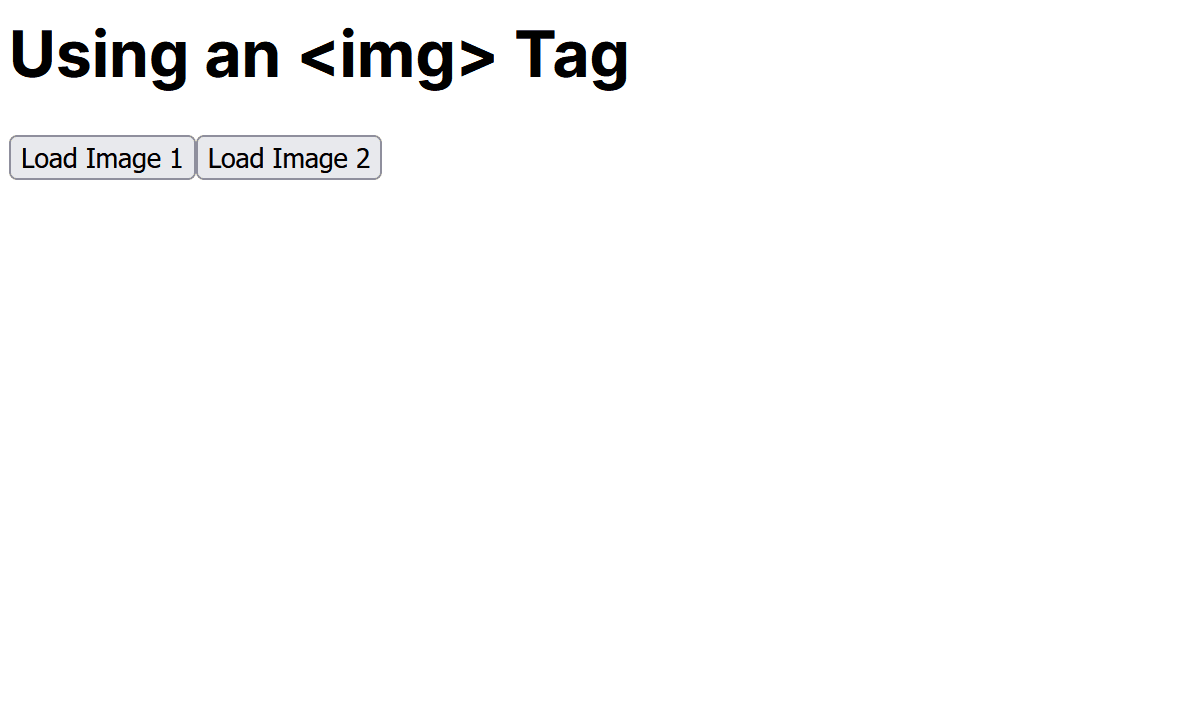
Share your thoughts in the comments
Please Login to comment...