How to listen for Prop Changes in VueJS ?
Last Updated :
25 Apr, 2024
In Vue.js, props provide the mechanism for parent components to share data with their child components. While props themselves are reactive, meaning changes in the parent component automatically trigger a re-render of the child component, sometimes we might need to react to specific prop changes within the child component.
Vue offers two primary approaches to achieve this: watchers and computed properties.
Setting Up the Project Environment
Step 1:Â Create a Vue.js application
npm create vue@latest
Step 2:Â Navigate to your project directory and install dependencies
cd your-project-name
npm install
Project Structure:
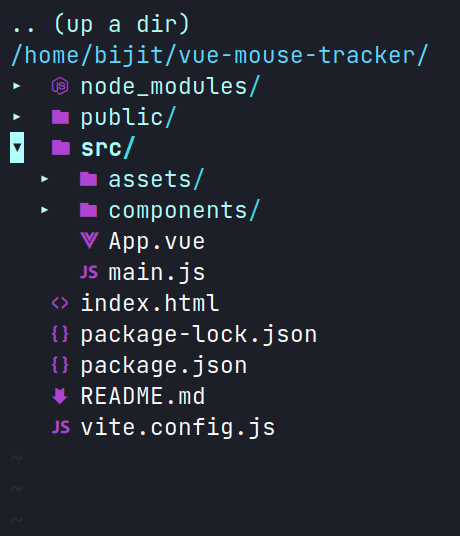
Project Structure
Listen for ‘Prop’ Changes Using Watchers
Watchers is used to monitor changes in specific data properties within component. When a prop being watched is updated, the watcher’s handler function is invoked. In the App.vue component the auth object is passing as props to ProfileShow Component. In Show.vue component the deep: true part of the set up is if we were watching an Object or an Array which would allow the watch to look into the data structure and check for changes.
Example: This example shows the use of watchers to listen the prop changes.
HTML
<!-- App.vue -->
<template>
<div class="home">
<vs-input label-placeholder="Enter your name"
v-model="auth.name" />
</div>
<ProfileShow :auth="auth" />
</template>
<script>
import ProfileShow from '@/components/Show.vue'
export default {
data() {
return {
auth: {
name: '',
}
}
},
components: {
ProfileShow
}
}
</script>
HTML
<!-- components/Show.vue -->
<template>
<div>
<h1> {{ auth.name }} </h1>
</div>
</template>
<script>
export default {
name: 'ProfileShow',
props: {
auth: {
type: Object,
required: true
}
},
watch: {
auth: {
handler(newVal) {
console.log(newVal.name)
},
deep: true
}
}
}
</script>
Output:
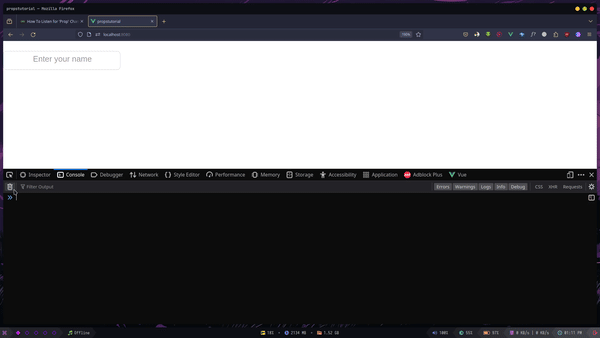
Output
Listen for ‘Prop’ Changes Using computed property
In this approach we are using computed property. Since props are reactive in Vue.js, whenever the prop value changes, the computed property will be automatically re-evaluated. Within the computed property’s function, we can perform calculations, manipulations, or any logic based on the updated prop value.
Example: This example shows the use of computed property to listen the prop changes.
HTML
<!-- App.vue -->
<template>
<div class="home">
<vs-input label-placeholder="Enter your name"
v-model="auth.name"/>
</div>
<ProfileShow :auth="auth"/>
</template>
<script>
import ProfileShow from '@/components/Show.vue'
export default {
data() {
return {
auth: {
name: '',
}
}
},
components: {
ProfileShow
}
}
</script>
HTML
<!-- Show.vue -->
<template>
<div>
<h1> {{ name }} </h1>
</div>
</template>
<script>
export default {
name: 'ProfileShow',
props: {
auth:{
type: Object,
required: true
}
},
computed: {
// Computed property for getting the user name
name() {
return `Hello ${this.auth.name} `;
}
}
}
</script>
Step to run the application:
Open the terminal and type the following command and Open your browser. Open a tab with localhost running (http://localhost:5173/).
npm installnpm run dev
Output:
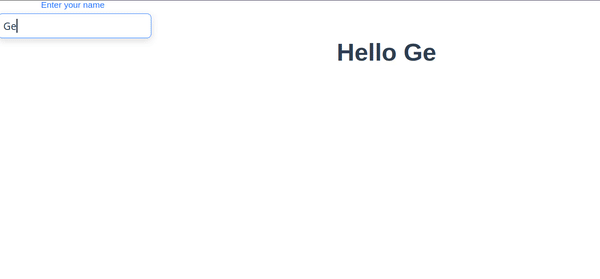
Output
Share your thoughts in the comments
Please Login to comment...