How to include a JavaScript File in Angular and call a Function from that Script ?
Last Updated :
07 Dec, 2023
In this article, we will learn how to include a JavaScript file in Angular and call a function, defined inside that file, from that script.
In Angular, we can include JavaScript script files to import some features or utilize some external libraries or pieces of code, which can be a great asset while developing a certain feature that is dependent on external sources. This can also save a significant amount of development time as it would involve not writing everything from scratch, and importing some already developed utilities.
Create an Angular app & Install Modules
Step 1: Create an Angular application by using the following command:
ng new AngularApp
Step 2: Create the Project Structure
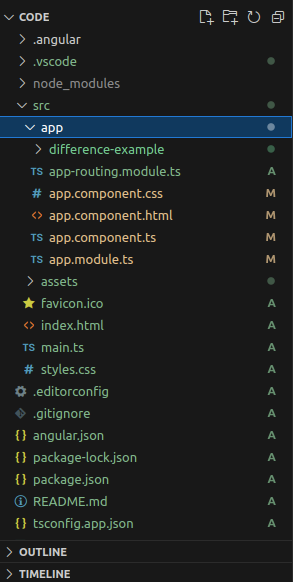
Â
Step 3: Create a script file named “logger.js“
Now, create a scripts directory inside the src directory, and inside that create a logger.js file. The logger.js file should have a function, print, that prints “Hello World!” to the console.
export function print() {
console.log('Hello World!!')
}
Step 4: Update the angular.json file to include the logger script
Update the angular.json file to include the newly created script, logger.js.
Javascript
{
"$schema" : "./node_modules/@angular/cli/lib/config/schema.json" ,
"version" : 1,
"newProjectRoot" : "projects" ,
"projects" : {
"my-angular-app" : {
"projectType" : "application" ,
"schematics" : {},
"root" : "" ,
"sourceRoot" : "src" ,
"prefix" : "app" ,
"architect" : {
"build" : {
"builder" : "@angular-devkit/build-angular:browser" ,
"options" : {
"outputPath" : "dist/my-angular-app" ,
"index" : "src/index.html" ,
"main" : "src/main.ts" ,
"polyfills" : [
"zone.js"
],
"tsConfig" : "tsconfig.app.json" ,
"assets" : [
"src/favicon.ico" ,
"src/assets"
],
"styles" : [
"src/styles.css"
],
"scripts" : [
"src/scripts/logger.js"
]
},
"configurations" : {
"production" : {
"budgets" : [
{
"type" : "initial" ,
"maximumWarning" : "500kb" ,
"maximumError" : "1mb"
},
{
"type" : "anyComponentStyle" ,
"maximumWarning" : "2kb" ,
"maximumError" : "4kb"
}
],
"outputHashing" : "all"
},
"development" : {
"buildOptimizer" : false ,
"optimization" : false ,
"vendorChunk" : true ,
"extractLicenses" : false ,
"sourceMap" : true ,
"namedChunks" : true
}
},
"defaultConfiguration" : "production"
},
"serve" : {
"builder" : "@angular-devkit/build-angular:dev-server" ,
"configurations" : {
"production" : {
"browserTarget" : "my-angular-app:build:production"
},
"development" : {
"browserTarget" : "my-angular-app:build:development"
}
},
"defaultConfiguration" : "development"
},
"extract-i18n" : {
"builder" : "@angular-devkit/build-angular:extract-i18n" ,
"options" : {
"browserTarget" : "my-angular-app:build"
}
},
"test" : {
"builder" : "@angular-devkit/build-angular:karma" ,
"options" : {
"polyfills" : [
"zone.js" ,
"zone.js/testing"
],
"tsConfig" : "tsconfig.spec.json" ,
"assets" : [
"src/favicon.ico" ,
"src/assets"
],
"styles" : [
"src/styles.css"
],
"scripts" : []
}
}
}
}
}
}
|
Step 5: Import the file and call the function inside the script
Go to the app.component.ts file, and import the logger.js script inside that file. Also, call the print() function which is declared inside that file.
Javascript
import { Component } from '@angular/core' ;
import * as logger
from "../scripts/logger"
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
title = 'my-angular-app' ;
ngOnInit(): void {
logger.print();
}
}
|
Step 6: Update tsconfig.json to allow js scripts
Finally, update the tsconfig.json to allow the use of js scripts inside the project.
Javascript
{
"compileOnSave" : false ,
"compilerOptions" : {
"baseUrl" : "./" ,
"outDir" : "./dist/out-tsc" ,
"allowJs" : true ,
"forceConsistentCasingInFileNames" : true ,
"strict" : true ,
"noImplicitOverride" : true ,
"noPropertyAccessFromIndexSignature" : true ,
"noImplicitReturns" : true ,
"noFallthroughCasesInSwitch" : true ,
"sourceMap" : true ,
"declaration" : false ,
"downlevelIteration" : true ,
"experimentalDecorators" : true ,
"moduleResolution" : "node" ,
"importHelpers" : true ,
"target" : "ES2022" ,
"module" : "ES2022" ,
"useDefineForClassFields" : false ,
"lib" : [
"ES2022" ,
"dom"
]
},
"angularCompilerOptions" : {
"enableI18nLegacyMessageIdFormat" : false ,
"strictInjectionParameters" : true ,
"strictInputAccessModifiers" : true ,
"strictTemplates" : true
}
}
|
Step 7: Run the application
Finally, Run the application with the command below:
ng serve
Output:
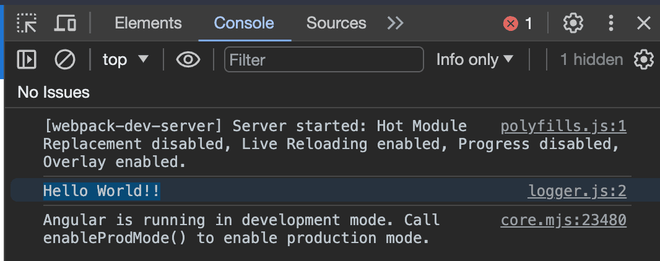
Example: In this example, we have changed the logger.js file to create an alertFn that will show an alert with “Hello World” at the click of a button.
HTML
app.component.html
< button (click)="handleClick()">Click Me!</ button >
|
Javascript
export function alertFn() {
alert( 'Hello World!!' )
}
|
Javascript
import { Component } from '@angular/core' ;
import * as logger from "../scripts/logger"
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
title = 'my-angular-app' ;
handleClick() {
logger.alertFn()
}
}
|
Output:

Share your thoughts in the comments
Please Login to comment...