How to hide div element by default and show it on click using JavaScript and Bootstrap ?
Last Updated :
15 Dec, 2023
We have to first hide the div and when a user will click the button we need to display that hiden div on the screen. It is helpul for creating any type of form.
There are two methods to solve this problem which are discussed below:Â
Example: This example implements the above approach.Â
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< style >
#div {
display: none;
background: green;
height: 100px;
width: 200px;
color: white;
}
</ style >
< link rel = "stylesheet" href =
< script src =
</ script >
</ head >
< body >
< h1 style = "color:green;" >
GeeksforGeeks
</ h1 >
< p id = "GFG_UP" ></ p >
< div id = "div" >
This is Div box.
</ div >
< br >
< button onClick = "GFG_Fun()" >
click here
</ button >
< br >
< p id = "GFG_DOWN" style = "color: green;" ></ p >
< script >
$('#GFG_UP').text(
`Click on button to toggle
the DIV Box using Bootstrap.`);
function show(divId) {
$("#" + divId).show();
}
function GFG_Fun() {
show('div');
$('#GFG_DOWN').text("DIV Box is visible.");
}
</ script >
</ body >
</ html >
|
Output:
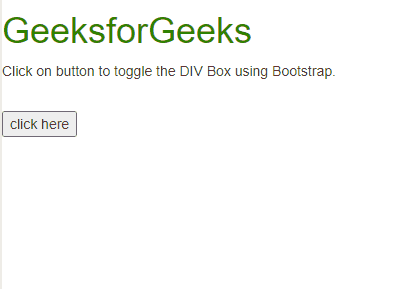
How to hide div element by default and show it on click using JavaScript and Bootstrap ?
- Set display: none property of the div that needs to be displayed.
- Use .toggle() method to display the Div. However, this method can be used to again hide the div.
Example: This example implements the above approach.Â
html
<!DOCTYPE html>
< html lang = "en" >
< head >
< style >
#div {
display: none;
background: green;
height: 100px;
width: 200px;
color: white;
}
</ style >
< link rel = "stylesheet" href =
< script src =
</ script >
</ head >
< body >
< h1 style = "color:green;" >
GeeksforGeeks
</ h1 >
< p id = "GFG_UP" >
</ p >
< div id = "div" >
This is Div box.
</ div >
< br >
< button onClick = "GFG_Fun()" >
click here
</ button >
< br >
< p id = "GFG_DOWN" style = "color: green;" >
</ p >
< script >
$('#GFG_UP').text(
`Click on button to toggle
the DIV Box using Bootstrap.`);
function toggler(divId) {
$("#" + divId).toggle();
}
function GFG_Fun() {
toggler('div');
$('#GFG_DOWN').text("DIV Box is toggling.");
}
</ script >
</ body >
</ html >
|
Output:
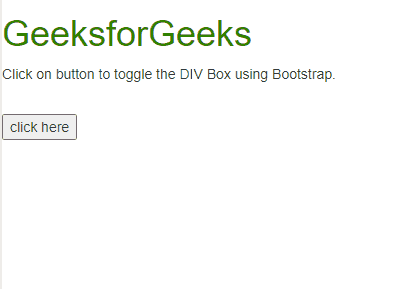
How to hide div element by default and show it on click using JavaScript and Bootstrap ?
Share your thoughts in the comments
Please Login to comment...