How to Hide a Paragraph Under an Image ?
Last Updated :
16 Apr, 2024
Hiding a paragraph under an image is a common requirement in web development, especially in cases where you want to reveal additional content when the user interacts with an image.
This article will explore various approaches to achieve this functionality using HTML and CSS.
Using CSS Positioning
This approach involves positioning the paragraph absolutely relative to the image container and setting its initial visibility to hidden. When the user interacts with the image, such as hovering over it, the paragraph’s visibility is changed to visible.
Example: This example shows the use of the above-explained approach.
HTML
<!DOCTYPE html>
<html>
<head>
<title>CSS Positioning</title>
</head>
<style>
/* CSS Here */
.image-container {
position: relative;
display: inline-block;
}
.hidden-paragraph {
position: absolute;
top: 0;
left: 0;
visibility: hidden;
}
.image-container:hover .hidden-paragraph {
visibility: visible;
}
</style>
<body>
<div class="image-container">
<img src=
"https://media.geeksforgeeks.org/wp-content/cdn-uploads/20190710102234/download3.png"
alt="Image">
<p class="hidden-paragraph">GeeksforGeeks is a computer science
portal that offers resources for programmers and technology
enthusiasts. It provides a wide range of courses, tutorials,
coding challenges, and interview preparation materials </p>
</div>
</body>
</html>
Output:
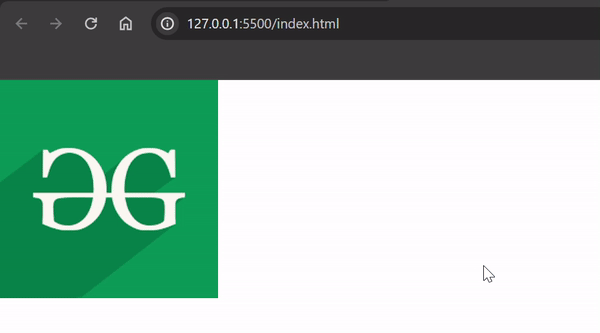
In this approach, the paragraph is initially hidden by setting its opacity to 0. When the user interacts with the image, such as hovering over it, the paragraph’s opacity is changed to 1, making it visible
Example: This example shows the use of the above-explained approach.
HTML
<!DOCTYPE html>
<html>
<head>
<title>CSS Positioning</title>
</head>
<style>
/* CSS Here */
.image-container {
position: relative;
display: inline-block;
}
.hidden-paragraph {
position: absolute;
top: 0;
left: 0;
opacity: 0;
transition: opacity 0.3s ease;
}
.image-container:hover .hidden-paragraph {
opacity: 1;
}
</style>
<body>
<div class="image-container">
<img src=
"https://media.geeksforgeeks.org/wp-content/cdn-uploads/20190710102234/download3.png"
alt="Image">
<p class="hidden-paragraph">GeeksforGeeks is a computer science
portal that offers resources for programmers and technology
enthusiasts. It provides a wide range of courses, tutorials,
coding challenges, and interview preparation materials </p>
</div>
</body>
</html>
Output:
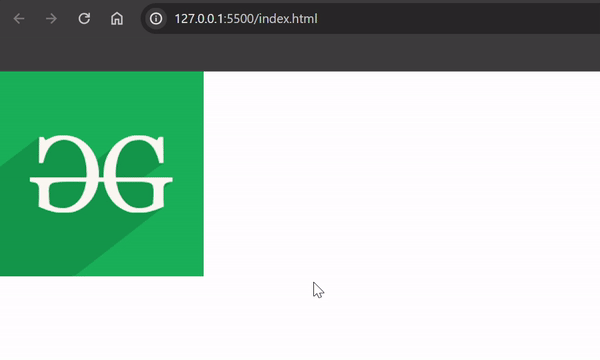
This approach involves initially hiding the paragraph by setting its display property to “none”. When the user interacts with the image, such as hovering over it, the display property of the paragraph is changed to block or inline, making it visible.
Example: This example shows the use of the above-explained approach.
HTML
<!DOCTYPE html>
<html>
<head>
<title>CSS Positioning</title>
</head>
<style>
/* CSS Here */
.image-container {
position: relative;
display: inline-block;
}
.hidden-paragraph {
position: absolute;
top: 0;
left: 0;
display: none;
}
.image-container:hover .hidden-paragraph {
display: block;
}
</style>
<body>
<div class="image-container">
<img src=
"https://media.geeksforgeeks.org/wp-content/cdn-uploads/20190710102234/download3.png"
alt="Image">
<p class="hidden-paragraph">GeeksforGeeks is a computer science
portal that offers resources for programmers and technology
enthusiasts. It provides a wide range of courses, tutorials,
coding challenges, and interview preparation materials </p>
</div>
</body>
</html>
Output:
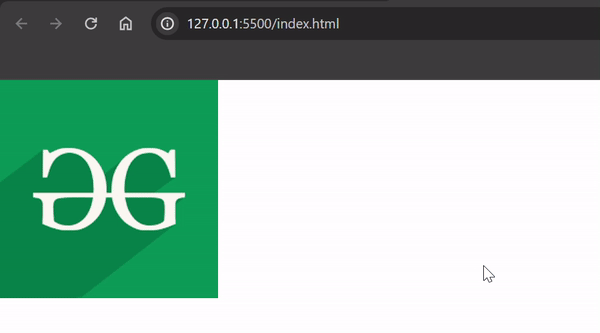
Using JavaScript
This approach involves using JavaScript to toggle the visibility of the paragraph based on user interaction with the image, such as clicking on it.
Example: This example shows the use of the above-explained approach.
HTML
<!DOCTYPE html>
<html>
<head>
<title>CSS Positioning</title>
</head>
<style>
/* CSS Here */
.image-container {
position: relative;
display: inline-block;
}
#hidden-paragraph {
position: absolute;
top: 0;
left: 0;
display: none;
}
</style>
<body>
<div class="image-container" onclick="toggleParagraphVisibility()">
<img src=
"https://media.geeksforgeeks.org/wp-content/cdn-uploads/20190710102234/download3.png"
alt="Image">
<p id="hidden-paragraph">GeeksforGeeks is a computer science
portal that offers resources for programmers and technology
enthusiasts. It provides a wide range of courses, tutorials,
coding challenges, and interview preparation materials </p>
</div>
</body>
<script>
function toggleParagraphVisibility() {
let paragraph = document.getElementById('hidden-paragraph');
paragraph.style.display = paragraph.style.display === 'none' ?
'block' : 'none';
}
</script>
</html>
Output:
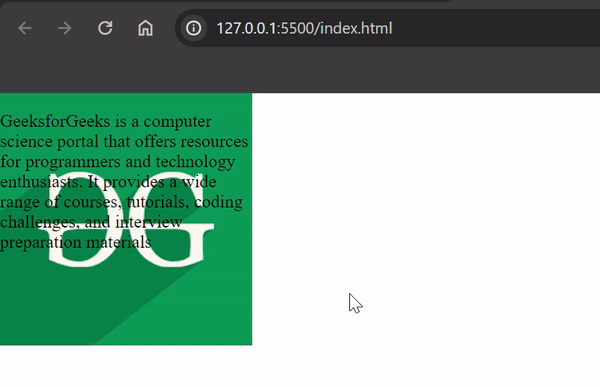
Share your thoughts in the comments
Please Login to comment...