How to make flexbox children 100% height of their parent using CSS?
Last Updated :
15 Mar, 2024
CSS Flexbox is a powerful tool that makes creating responsive layouts easy. It allows you to distribute available space among child elements in an efficient way.Â
To make flexbox children 100% the height of their parent, you need to set the parent’s `display` property to `flex`. To make the children 100% height to their parent, use either `height: 100%` or the `align` property. This ensures that the children expand to fill the entire height of the parent, and the available space is distributed evenly among them.
Let’s learn these methods in detail with examples to get a better understanding.
How to Make Flex Child Equal Height of Parent Container
Making flexbox child elements equal to the same height as their flexbox parent helps in the proper utilization of space and adds symmetry to the parent flexbox element. It is also used to create flexbox height fit content. There are two methods to make flexbox children 100% height of the container and make flex height fit content:
Using Flexbox and Height Property
We can use the display: flex property to create a flexbox child and use the height: 100% property to make flex height 100% of container. This method ensures that the child flexbox takes the full height of the parent container.
Example: This example makes a child flex-box of height 100% of container using CSS.Â
html
<!DOCTYPE html>
<html>
<head>
<title>
Using flex-box and height 100% property
</title>
<style>
* {
padding: 0;
margin: 0;
}
.container {
width: 100vw;
height: 20vh;
background-color: green;
display: flex;
justify-content: center;
align-items: center;
}
.child-div {
height: 100%;
background-color: red;
margin: 0 20px;
}
</style>
</head>
<body>
<div class="container">
<div class="child-div">
One
</div>
<div class="child-div">
Two
</div>
<div class="child-div">
Three
</div>
<div class="child-div">
Four
</div>
<div class="child-div">
Five
</div>
</div>
</body>
</html>
Output:Â Â
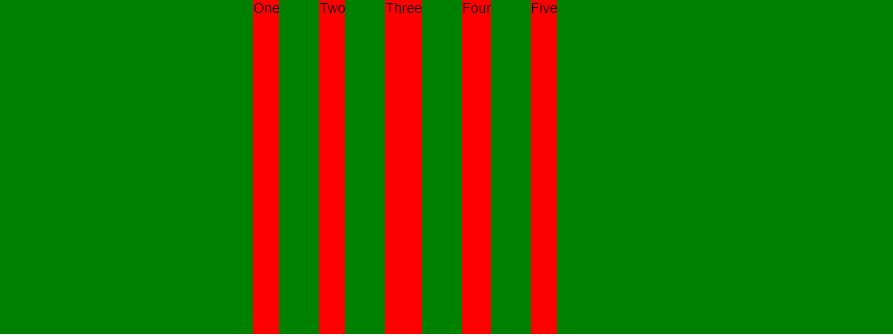
Using align-item Property
The align-items: stretch; approach ensures that flexbox children automatically stretch vertically to fill the entire height of their parent container, eliminating the need for explicit height declarations on the children.
Example: The example uses the align-items property in the parent div to ‘stretch’. It makes every .child-div 100% height of its parent height. Â
html
<!DOCTYPE html>
<html>
<head>
<title>
Using align property
</title>
<style>
* {
padding: 0;
margin: 0;
}
.container {
width: 100vw;
background-color: green;
display: flex;
justify-content: center;
align-items: stretch;
height: 30vh;
}
.child-div {
background-color: red;
margin: 0 20px;
}
</style>
</head>
<body>
<div class="container">
<div class="child-div">
One
</div>
<div class="child-div">
Two
</div>
<div class="child-div">
Three
</div>
<div class="child-div">
Four
</div>
<div class="child-div">
Five
</div>
</div>
</body>
</html>
Output:Â Â
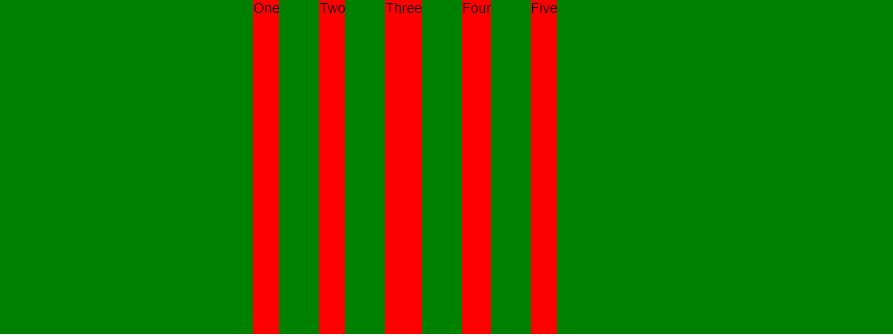
Additional Tip
You can also use these same methods to make flexbox children 100% width of their parent container in CSS. To make flexbox children same width as parent flexbox we can do following modifications:
Using flexbox and width property: Use display: flex and width: 100% properties to flexbox make child full width of parent flex. This will allows to create flex width fit content.
CSS is the foundation of webpages and is used for webpage development by styling websites and web apps. You can learn CSS from the ground up by following this CSS Tutorial and CSS Examples.
Summing Up
Aligning flexbox children inside parent flexbox can be annoying for many young developers. In this tutorial we have covered the methods to make flexbox children same height as the parent.
Creating flexbox children full height of the parent allows us to use all height of parent container. We have also shared a quick tip to flexbox make child full width, which utilizes the 100% width of the parent container.
Share your thoughts in the comments
Please Login to comment...