How to Handle Different Environments in a Next.js?
Last Updated :
06 May, 2024
Environment variables in NextJS are fundamental settings or values that can change based on the application’s deployment environment. They are crucial for separating sensitive information, such as API keys, database credentials, or configuration details, from the application codebase.
In a NextJS project, managing environment variables is essential for handling sensitive information and configuring applications across different deployment environments.
Understanding Environment Variables in NextJS
NextJS supports different types of environment variables:
- Server-Side Environment Variables: These variables are accessible only on the server side of the application. They are ideal for storing secrets or sensitive information that should not be exposed to the client-side code running in the browser.
- Client-Side Environment Variables (Prefixed with NEXT_PUBLIC_): Variables prefixed with NEXT_PUBLIC_ are exposed to the browser and can be accessed in client-side code. This is useful for configurations that need to be available on the client side, such as public API keys.
Setting Up Environment Variables in NextJS
There are several approaches to set up Environment Variables in Next.js:
Using Environment Variables (`process.env`):
NextJS supports reading environment variables using `process.env` which allows you to define different values for different environments.
- Approach: Define environment-specific variables directly in the host environment (e.g., local machine, staging server, production server) or through tools like `.env` files.
const apiUrl = process.env.NEXT_PUBLIC_API_URL;
`.env` Files for Environment-Specific Configuration:
Use different `.env` files for each environment (development, staging, production) to store environment-specific configuration.
- Approach: Create `.env.development`, `.env.staging`,`.env.production` files to define environment-specific variables.
// .env.development
API_URL=http://localhost:3000/api
// .env.production
API_URL=https://api.example.com
Conditional Logic Based on Environment:
Use conditional logic to determine environment-specific behavior or configurations.
- Approach: Check the current environment (process.env.NODE_ENV) and conditionally set variables or configurations.
const apiUrl = process.env.NODE_ENV === 'production' ? 'https://api.example.com' : 'http://localhost:3000/api';
Configuration Modules or Files:
Create separate configuration modules or files (`config.js`, `config.json`) for different environments and import the appropriate configuration based on the environment.
- Approach: Define environment-specific configurations in separate files and import the correct configuration module.
const config = {
development: {
apiUrl: 'http://localhost:3000/api',
},
production: {
apiUrl: 'https://api.example.com',
},
};
module.exports = config[process.env.NODE_ENV];
Using NextJS `publicRuntimeConfig` and `serverRuntimeConfig`
Leverage NextJS specific runtime configuration options to manage environment-specific variables.
- Approach: Use `publicRuntimeConfig` for variables that are exposed to the client-side and `serverRuntimeConfig` for variables that are only accessible on the server-side.
module.exports = {
publicRuntimeConfig: {
apiUrl: process.env.API_URL,
},
};
Using dotenv for Environment Variable Management
Step 1: Setting Up a NextJS Project:
npx create-next-app my-next-app
cd my-next-app
Step 3: Install Dependencies using the following command:
npm install dotenv
Project Structure:
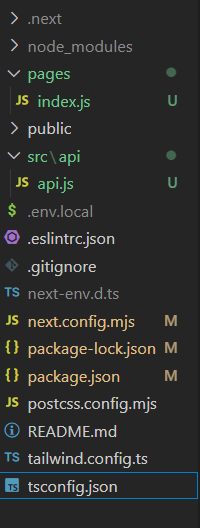
project structure
After installation the dependencies will be updated in package.json:
"dependencies": {
"dotenv": "^16.4.5",
"next": "14.2.2",
"react": "^18",
"react-dom": "^18"
},
Step 4: Set Up Environment Variables
# .env.local
API_URL=http://localhost:3000/workout
Example: Below is an example to Handle Environments in a NextJS app.
JavaScript
// next.config.mjs
import dotenv from 'dotenv';
dotenv.config();
const config = {
env: {
API_URL: process.env.API_URL,
},
};
export default config;
JavaScript
// src/api/api.js
const fetchUserData = async () => {
const apiUrl = process.env.API_URL;
const response = await fetch(`${apiUrl}`);
const data = await response.json();
return data;
};
export default fetchUserData;
JavaScript
import React, {
useState,
useEffect
} from 'react';
const Home = () => {
const [apiContent, setApiContent] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(process.env.API_URL);
if (response.ok) {
const data = await response.json();
setApiContent(data);
} else {
throw new Error('Failed to fetch API data');
}
} catch (error) {
console.error('Error fetching API data:', error);
}
};
fetchData();
}, []);
return (
<div>
<h1>Welcome to my Next.js App</h1>
{apiContent ? (
<div>
<h2>API Content</h2>
<pre>{JSON.stringify(apiContent, null, 2)}</pre>
</div>
) : (
<p>Loading API content...</p>
)}
</div>
);
};
Start your application using the following command:
npm run dev
Output:
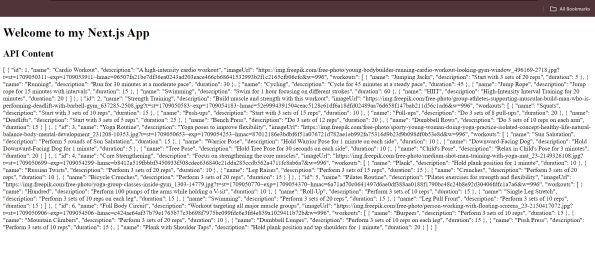
output: api data
Best Practices for Managing Environment Variables in NextJS
- Security: Keep sensitive information like API keys or credentials separate from the codebase and use .env files.
- Environment-specific Files: Create distinct configuration files or modules for different environments to maintain clarity and organization.
- Avoid Hardcoding: Refrain from hardcoding secrets directly into the application code.
- Version Control: Exclude .env files from version control systems to prevent exposing secrets.
Conclusion
In conclusion, effective environment handling in NextJS is crucial for securely managing configuration settings and sensitive information across different deployment environments. By utilizing environment variables, developers can ensure that critical details like API keys, database credentials, and other confidential information remain isolated from the application codebase. This separation enhances security and flexibility, enabling applications to seamlessly adapt to diverse environments such as development, staging, and production.
Share your thoughts in the comments
Please Login to comment...