How to Get the Content of an HTML Comment using JavaScript ?
Last Updated :
14 Jun, 2023
In this article, we will learn how to get the content of an HTML Comment Using Javascript. Comments are a best practice in programming and software development. In general, they can explain why a coding decision was made or what needs to be done to improve the code you’re working on.
HTML tags (including HTML comments) are represented in the DOM tree as nodes, with each node having a nodeType property which is a numerical value that identifies the type of node. The childNodes property returns a nodeList object containing the node’s child nodes. Comments and white spaces are also considered nodes. The nodes are given index numbers beginning with 0. Searching and sorting operations on the node list can be performed using the index number.
Syntax
<! -- Comments here -->
Approaches: Using the Array.from() method: The JavaScript Array from() method returns an Array object from any object with a length property or an iterable object.
Example 1: In this example, we use an array.from() method, The code creates a div element with a comment and a paragraph. It extracts the comment text from the div and displays it as an h1 element with the ID “content” using querySelector and childNodes.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >GeeksforGeeks</ title >
< style >
#content {
color: green;
font-weight: bold;
font-size: 2.3rem;
margin: 2rem;
}
p {
color: rgb(32, 29, 29);
}
</ style >
</ head >
< body >
< div >
< p >
A Computer Science portal for geeks.
It contains well written, well thought and
well explained computer
science and programming articles
</ p >
</ div >
< script >
const comment =
Array.from(document.querySelector('div').childNodes)
.find(node => node.nodeType === Node.COMMENT_NODE
&& node.textContent.trim());
document.write
(`< h1 id = "content" >
${comment ? comment.textContent.trim() : ''}</ h1 >`);
</ script >
</ body >
</ html >
|
Output:
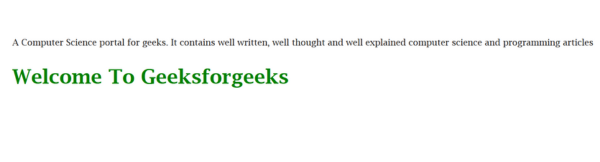
How to Get the Content of an HTML Comment Using JavaScript
Using the replace() method: The replace() method in JavaScript is used to search a string for a value or any expression and replace it with the new value provided in the parameters.
Example 2: In this example, The JavaScript code extracts text from HTML comments to update the heading dynamically, displaying “Welcome To Geekforgeeks”.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Geeksforgeeks</ title >
</ head >
< style >
div {
color: green;
}
</ style >
< body >
< div >
</ div >
< p >
Geeksforgeeks is a computer science portal
</ p >
< script >
let htmlContent = document.body.innerHTML;
let commentRegex = '/ /';
// Extract the content of the HTML comment
let commentContent =
htmlContent.replace(commentRegex, function (match, group) {
return group.trim();
// Trim any leading/trailing whitespace
});
let modifiedHtmlContent =
htmlContent.replace(commentRegex, '< h1 >$1</ h1 >');
document.body.innerHTML = modifiedHtmlContent;
</ script >
</ body >
</ html >
|
Output:
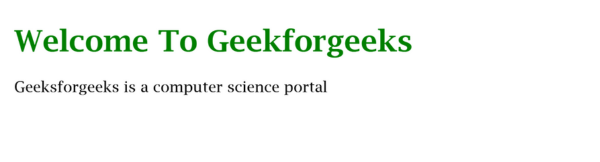
How to Get the Content of an HTML Comment Using JavaScript
Share your thoughts in the comments
Please Login to comment...