How to get Matching Data from a Prompt on an Existing Object ?
Last Updated :
15 Mar, 2024
In JavaScript, getting matching data from an object can be done using various approaches like using traditional looping or by using the Object properties. JavaScript provided various approaches for matching data from a prompt on an existing object which are as follows:
Using for…in Loop
In this approach, we are using a for…in loop to iterate through the properties of the existing object, allowing the user to enter a key. The script checks if the entered key matches any property in the object and, if found, displays the corresponding information.
Syntax:
for (key in object) {
// code
}
Example: The below example uses for…in Loop to get matching data from a prompt on an existing object.
HTML
<!DOCTYPE html>
<head>
<title>Example 1</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
padding: 20px;
}
h1 {
color: green;
}
h3 {
margin-top: 10px;
}
button {
padding: 10px;
margin-top: 20px;
cursor: pointer;
}
</style>
</head>
<body>
<h1>GeeksforGeeks</h1>
<h3>Using a for...in loop</h3>
<button onclick="approach1Fn()">Find Data</button>
<script>
function approach1Fn() {
const obj = {
name: 'GeeksforGeeks',
category: 'Educational',
website: 'https://www.geeksforgeeks.org/',
founded: 2009,
};
const uInput = prompt(
'Enter data key to retrieve information:\n\nReference keys: '
+ Object.keys(obj).join(', '));
for (const key in obj) {
if (key === uInput) {
alert(`${key}: ${obj[key]}`);
return;
}
}
alert('Data not found');
}
</script>
</body>
</html>
Output:
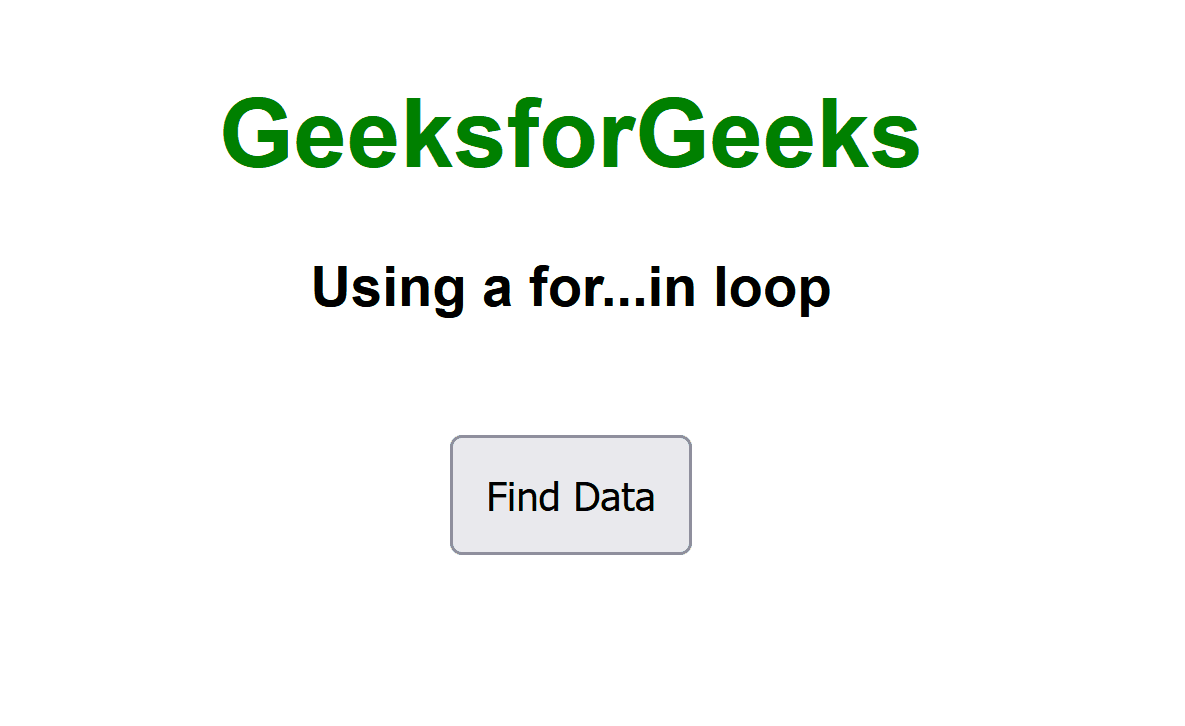
Using Object.hasOwnProperty
In this approach, we are using Object.hasOwnProperty to check if the entered key exists in the existing object, allowing for the retrieval of corresponding data. The user is prompted to enter a key, and the script loops through the object properties, handling nested structures and displaying the result in a user-friendly format.
Syntax:
object.hasOwnProperty( prop );
Example: The below example uses Object.hasOwnProperty to get matching data from a prompt on an existing object.
HTML
<!DOCTYPE html>
<head>
<title>Example 2</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
}
h1 {
color: green;
}
</style>
</head>
<body>
<h1>GeeksforGeeks</h1>
<h3>Using Object.hasOwnProperty </h3>
<button onclick="approach2Fn()">Find Data</button>
<script>
function approach2Fn() {
const obj = {
name: 'GeeksforGeeks',
category: 'Educational',
website: 'https://www.geeksforgeeks.org/',
founded: 2009,
details: {
founder: 'Sandeep Jain',
headquarters: 'Noida, India',
courses: ['DSA', 'Web Development', 'Machine Learning'],
},
};
while (true) {
const uInput = prompt(
`Enter a key to retrieve GeeksforGeeks data. Available keys:
${getFn(obj)}`);
if (uInput === null) {
break;
}
const res = getfromPathFn(obj, uInput.split('.'));
const resStr = resFn(res);
if (res !== undefined) {
alert(`GeeksforGeeks ${uInput}: ${resStr}`);
} else {
alert(`Data with key "${uInput}" not found.`);
}
}
}
function getFn(object, parentKey = '') {
return Object.keys(object)
.map(key => parentKey ? `${parentKey}.${key}` : key)
.join(', ');
}
function getfromPathFn(object, keyPath) {
let curr = object;
for (const key of keyPath) {
if (!curr.hasOwnProperty(key)) {
return undefined;
}
curr = curr[key];
}
return curr;
}
function resFn(result) {
if (typeof result === 'object' && result !== null) {
if (Array.isArray(result)) {
return `[${result.join(', ')}]`;
} else {
return JSON.stringify(result);
}
}
return result;
}
</script>
</body>
</html>
Output:
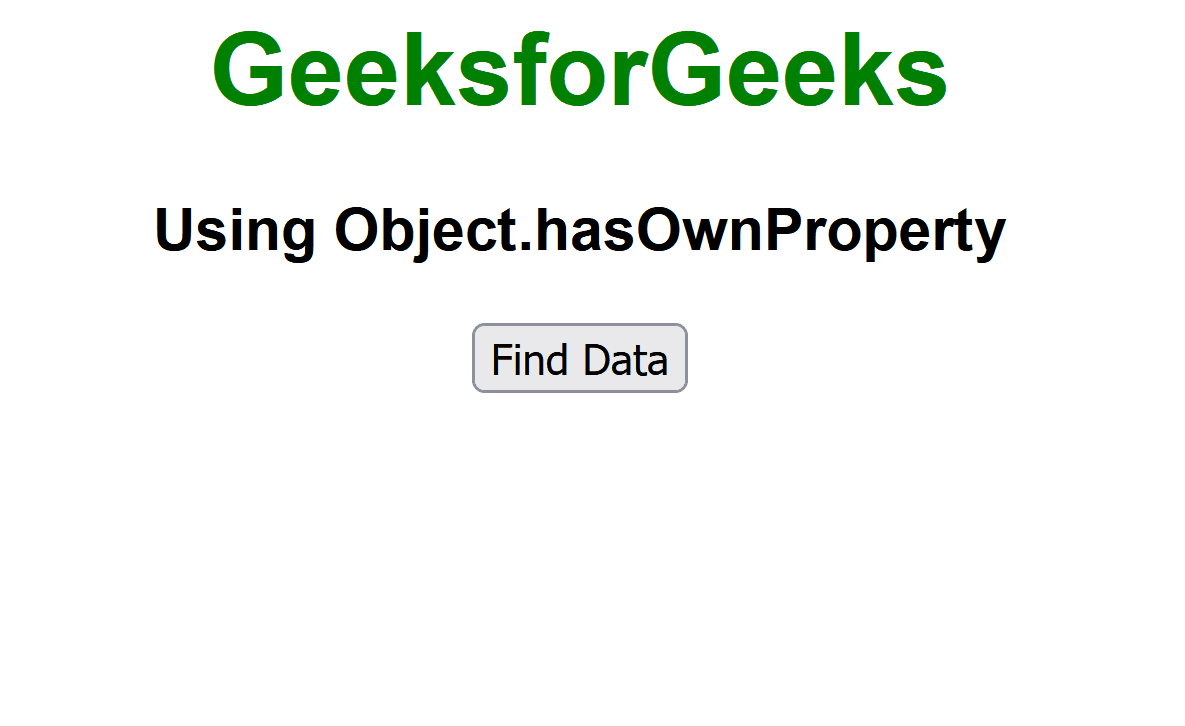
Share your thoughts in the comments
Please Login to comment...