How to Fix the “partial is not defined” Error in EJS ?
Last Updated :
08 Apr, 2024
Encountering an error message like “partial is not defined” can be a stumbling block when you’re working with EJS (Embedded JavaScript) templates. This error typically pops up when you’re trying to use the `partial` function in EJS, but it’s not being recognized. Don’t worry, though it’s a common hiccup for many developers, and we’ve got you covered with some straightforward solutions.
Using the include Syntax
EJS has moved away from the `partial` function in favor of the `include` statement. Here’s how you can use it:
<%- include('path/to/partial') %>
Ensuring EJS is Properly Installed
Sometimes, the error arises because EJS isn’t installed correctly. Run the following command to install or update EJS:
npm install ejs
Checking the Version of EJS
Make sure you’re using a version of EJS that supports the `include` syntax. Update your EJS if it’s outdated:
npm update ejs
Steps to Create a NodeJS App and Installing Module:
Step 1: Firstly, we will make the folder named root by using the below command in the VScode Terminal. After creation use the cd command to navigate to the newly created folder.
mkdir root
cd root
Step 2: Once the folder is been created, we will initialize NPM using the below command, this will give us the package.json file.
npm init -y
Step 3: Once the project is been initialized, we need to install Express and EJS dependencies in our project by using the below installation command of NPM.
npm i express ejs
Project Structure:
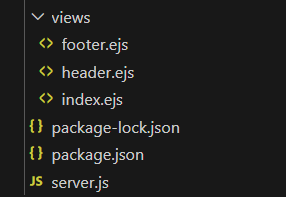
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2",
"ejs": "^3.1.9",
}
Example: Here’s a simple example of an EJS file using the `include` statement:
HTML
<!DOCTYPE html>
<html>
<head>
<title>My EJS Page</title>
</head>
<body>
<%- include('header') %> <!-- This is where your header partial goes -->
<h1>Welcome to My Page</h1>
<%- include('footer') %> <!-- And this is your footer partial -->
</body>
</html>
HTML
<header>
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
<li><a href="/contact">Contact</a></li>
</ul>
</nav>
</header>
HTML
<footer>
<p>© 2024 My EJS Page. All rights reserved.</p>
</footer>
JavaScript
// app.js
const express = require('express');
const ejs = require('ejs');
const path = require('path');
const app = express();
// Set EJS as the view engine
app.set('view engine', 'ejs');
// Set the path for the views directory
app.set('views', path.join(__dirname, 'views'));
// Route to render the EJS file
app.get('/', (req, res) => {
res.render('index');
});
// Start the server
const PORT = process.env.PORT || 4000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Output:
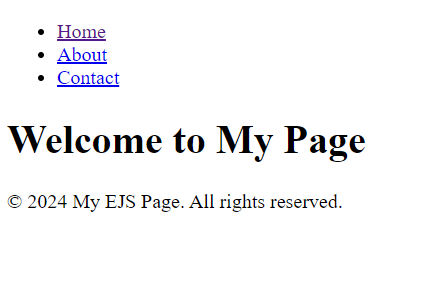
To Fix the “partial is not defined” Error in EJS
Share your thoughts in the comments
Please Login to comment...