How to Fetch data faster in Next.js?
Last Updated :
08 May, 2024
NextJS, a popular React framework provides various approaches to fetch data efficiently. Optimizing data fetching can significantly improve the performance and user experience of your NextJS applications.
We will discuss different approaches to Fetch data faster in NextJS:
Steps to Create the NextJS App
Step 1: Create the app using the following command:
npx create-react-app fetchdata
Step 2: Navigate to the root directory of your project using the following command:
cd fetchdata
Project Structure: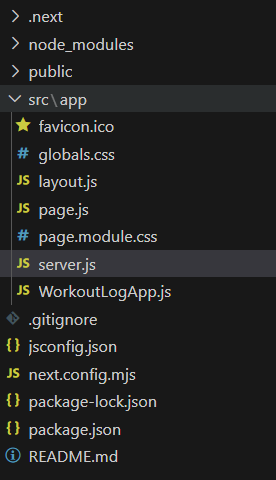
Example: Create a file name it as “server.js” for API setup for data fetching:
JavaScript
//server.js
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3000;
const posts = [
{
"userId": 1,
"id": 1,
"title": `sunt aut facere repellat provident occaecati
excepturi optio reprehenderit`,
"body": `quia et suscipit\nsuscipit recusandae consequuntur expedita
et cum\nreprehenderit molestiae ut ut quas totam\nnostrum
rerum est autem sunt rem eveniet architecto`
},
{
"userId": 1,
"id": 2,
"title": "qui est esse",
"body": `est rerum tempore vitae\nsequi sint nihil reprehenderit dolor
beatae ea dolores neque\nfugiat blanditiis voluptate porro vel
nihil molestiae ut reiciendis\nqui aperiam non debitis possimus
qui neque nisi nulla`
},
{
"userId": 1,
"id": 3,
"title": "ea molestias quasi exercitationem repellat qui ipsa sit aut",
"body": `et iusto sed quo iure\nvoluptatem occaecati omnis eligendi aut
ad\nvoluptatem doloribus vel accusantium quis pariatur\nmolestiae
porro eius odio et labore et velit aut`
},
{
"userId": 1,
"id": 4,
"title": "eum et est occaecati",
"body": `ullam et saepe reiciendis voluptatem adipisci\nsit amet
autem assumenda provident rerum culpa\nquis hic commodi
nesciunt rem tenetur doloremque ipsam iure\nquis sunt
voluptatem rerum illo velit`
},
{
"userId": 1,
"id": 5,
"title": "nesciunt quas odio",
"body": `repudiandae veniam quaerat sunt sed\nalias aut fugiat sit autem
\sed est\nvoluptatem omnis possimus esse voluptatibus quis\nest
aut tenetur dolor neque`
},
{
"userId": 1,
"id": 6,
"title": "dolorem eum magni eos aperiam quia",
"body": `ut aspernatur corporis harum nihil quis provident sequi\nmollitia
nobis aliquid molestiae\nperspiciatis et ea nemo ab reprehenderit
accusantium quas\nvoluptate dolores velit et doloremque molestiae`
},
{
"userId": 1,
"id": 7,
"title": "magnam facilis autem",
"body": `dolore placeat quibusdam ea quo vitae\nmagni quis enim qui quis
quo nemo aut saepe\nquidem repellat excepturi ut quia\nsunt ut
sequieos ea sed quas`
}
];
// Route to get all posts
app.get('/posts', (req, res) => {
res.json(posts);
});
// Route to get a specific post by its ID
app.get('/posts/:id', (req, res) => {
const postId = parseInt(req.params.id);
const post = posts.find(post => post.id === postId);
if (post) {
res.json(post);
} else {
res.status(404).json({ error: 'Post not found' });
}
});
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Static Generation using getStaticProps
Static Generation is a Next.js feature that generates HTML pages at build time. This means that the content of these pages is pre-rendered and served as static files, making them extremely fast to load. Static Generation is suitable for pages with content that doesn’t change frequently, such as marketing pages, blogs, or product listings.
How it Works:
- When you use getStaticProps in a Next.js page, Next.js will pre-render that page at build time.
- During the build process, Next.js calls getStaticProps, fetches data, and generates the HTML for the page with that data.
- The generated HTML is then served to the client, making subsequent visits to the page very fast as the content is already there.
Use Cases:
- Content that doesn’t change often (e.g., blog posts, product descriptions).
- SEO-friendly pages as search engines can crawl and index the static content easily.
- Improving initial load times for users.
Syntax:
export async function getStaticProps() {
// Fetch data from an API or database
const data = await fetchData();
return {
props: {
data,
},
};
}
Example: Below is an example of Fetching data using getStaticProps in NextJS App.
JavaScript
// index.js
export default function Home({ data }) {
return <div>{JSON.stringify(data)}</div>;
}
export async function getStaticProps() {
const res = await fetch('https://jsonplaceholder.typicode.com/posts');
const data = await res.json();
return {
props: {
data,
},
};
}
Output
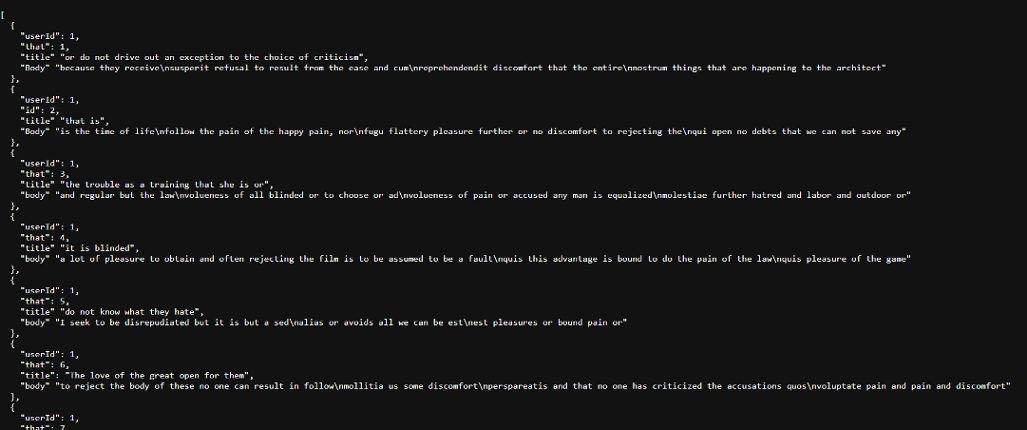
Server-side Rendering using getServerSideProps
Server-side Rendering (SSR) in Next.js involves fetching data on each request from the server before rendering the page. This ensures that the content is always up-to-date and can be personalized for each user. SSR is suitable for pages with dynamic content or data that changes frequently.
How it Works:
- When a user visits an SSR-enabled page, Next.js calls getServerSideProps.
- Inside getServerSideProps, you can fetch data from an API, database, or any other source.
- Next.js then generates the HTML for the page with the fetched data and sends it to the client.
Use Cases:
- Pages with dynamic content (e.g., user dashboards, real-time analytics).
- Personalized content based on user sessions or preferences.
- Ensuring data freshness for each user visit.
Syntax:
export async function getServerSideProps() {
// Fetch data from an API or database
const data = await fetchData();
return {
props: {
data,
},
};
}
Example: Below is an example of fetching data using getServerSideProps in NextJS App.
JavaScript
// product/[id].js
export default function Product({ data }) {
return <div>{JSON.stringify(data)}</div>;
}
export async function getServerSideProps(context) {
const { params } = context;
const res = await fetch(`https://jsonplaceholder.typicode.com/posts/[id]`);
const data = await res.json();
return {
props: {
data,
},
};
}
Output

Client-side Data Fetching with useSWR and fetch
Client-side Data Fetching involves fetching data directly from the client-side, after the initial HTML has been loaded. This approach is suitable for parts of the application that require frequent data updates without changing the entire page structure.
How it Works:
- In Next.js, you can use libraries like SWR or native JavaScript fetch to fetch data on the client-side.
- SWR is a popular library that handles data fetching, caching, and revalidating data automatically.
- When data is fetched on the client-side, it can be dynamically updated without requiring a full page reload.
Use Cases:
- Real-time updates (e.g., live chat messages, stock prices).
- Interactive components that fetch data based on user interactions (e.g., search results, infinite scrolling).
- Parts of the page that don’t need SEO optimization or initial server-side rendering.
Syntax (useSWR):
import useSWR from 'swr';
const fetcher = (url) => fetch(url).then((res) => res.json());
export default function Profile() {
const { data, error } = useSWR('/api/user', fetcher);
if (error) return <div>Error loading data</div>;
if (!data) return <div>Loading...</div>;
return <div>{JSON.stringify(data)}</div>;
}
Example: Below is an example of fetching data using useSWR in NextJS App
JavaScript
// index.js
import { useEffect, useState } from 'react';
export default function Profile() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('/api/user')
.then((res) => res.json())
.then((data) => setData(data))
.catch((error) => console.error('Error fetching data:', error));
}, []);
if (!data) return <div>Loading...</div>;
return <div>{JSON.stringify(data)}</div>;
}
Output:

Share your thoughts in the comments
Please Login to comment...