How to dynamically load module in React.js ?
Last Updated :
02 Nov, 2023
In React JS loading of modules statically is cumbersome as it loads the modules beforehand even if it is not rendered. Some components render only when the user interacts, so in such cases, it can lead to more resource consumption. When you statically import modules, you are loading larger data than you may actually need, which could lead to a slower initial page load. To solve this problem we import the modules dynamically. Further, will understand how this can be done.Â
Prerequisite
Let’s understand using step-by-step implementation.Â
Steps to Create the React application
Step 1: Use create-react-app to build a react
npm create-react-app <project_name>
Step 2: Move to the project directory
cd <project_name>
Project Structure:
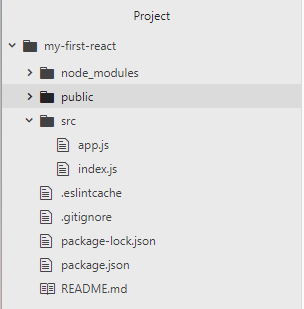
Â
Using static loading Component
Example: This example recognize the static load of resources in ReactJS. The component displays text and that is imported statically from another file into the main file. It gets loaded beforehand.
Javascript
import React from 'react' ;
import * as ReactDom from 'react-dom' ;
import App from './app'
const Btn = () => {
const [disp, setDisp] = React.useState( true );
return (disp) ? (<button onClick={() =>
setDisp( false )}>Click Me</button>) : <App />
}
ReactDom.render(<Btn />, document.getElementById( 'root' ));
|
Javascript
import React from "react" ;
import ReactDom from "react-dom" ;
class App extends React.Component {
constructor(props) {
super (props);
}
render() {
return (
<p>
The Content is loaded on GeeksforGeeks....
</p>
);
}
}
export default App;
|
Steps to Run the Application: Use this command in the terminal in the project directory.
npm start
Output: This output will be visible on http://localhost:3000/ in the browser
.gif)
Explanation: In the above example, the 0.chunk.js is loaded beforehand immediately when the app loads. Here the modules are imported at the compile time.
Dynamically loading module using React.lazy()
To import the modules dynamically ReactJS supports React.lazy( ) method. Â This method accepts a function as an argument and renders the component as a regular component and the function must call the import( ) that returns a promise. This is used along with <Suspense> because it allows us to display the fallback content while we are waiting for the module to load.
Example: This example lazy load the app component when the button is clicked to imnplement dynamic loading.
Javascript
import React from 'react' ;
import * as ReactDom from 'react-dom' ;
const Component = React.lazy(() => import( './app' ))
const Btn = () => {
const [disp, setDisp] = React.useState( true );
return (disp) ? (<button onClick={() =>
setDisp( false )}>Click Me</button>) :
(<React.Suspense fallback={<div>Loading...</div>}>
<Component />
</React.Suspense>)
}
ReactDom.render(<Btn />, document.getElementById( 'root' ));
|
Javascript
import React from "react" ;
class Text extends React.Component {
constructor(props) {
super (props);
}
render() {
return (
<p>
The Content is loaded on GeeksforGeeks....
</p>
);
}
}
export default Text;
|
Steps to Run the Application: Use this command in the terminal in the project directory.
npm start
Output: This output will be visible on http://localhost:3000/ in the browser.
.gif)
Share your thoughts in the comments
Please Login to comment...